Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / AspNet / BuildProviderUtils.cs / 1305376 / BuildProviderUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.CodeDom; using System.CodeDom.Compiler; using System.Globalization; using System.IO; using System.Resources; using System.Web; using System.Web.Compilation; using System.Web.Hosting; namespace System.Data.Entity.Design.AspNet { ////// A place to put common methods used by our build providers /// /// internal class BuildProviderUtils { ////// Default constructor /// private BuildProviderUtils() { } internal static void AddArtifactReference(AssemblyBuilder assemblyBuilder, BuildProvider prov, string virtualPath) { // add the artifact as a resource to the DLL using (Stream input = VirtualPathProvider.OpenFile(virtualPath)) { // derive the resource name string name = BuildProviderUtils.GetResourceNameForVirtualPath(virtualPath); using (Stream resStream = assemblyBuilder.CreateEmbeddedResource(prov, name)) { int byteRead = input.ReadByte(); while (byteRead != -1) { resStream.WriteByte((byte)byteRead); byteRead = input.ReadByte(); } } } } ////// Transforms a virtual path string into a valid resource name. /// /// ///internal static string GetResourceNameForVirtualPath(string virtualPath) { string name = VirtualPathUtility.ToAppRelative(virtualPath); Debug.Assert(name.StartsWith("~/"), "Expected app-relative path to start with ~/"); if (name.StartsWith("~/", StringComparison.OrdinalIgnoreCase)) { name = name.Substring(2); } name = name.Replace("/", "."); Debug.Assert(name.StartsWith(".") == false, "resource name unexpectedly starts with ."); return name; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
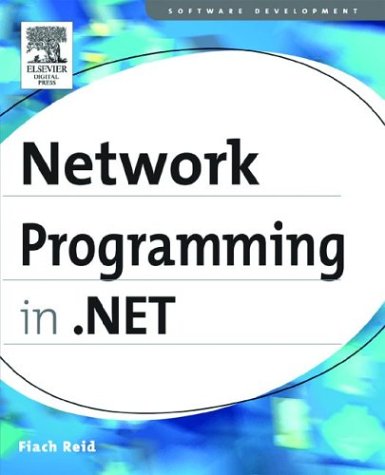
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExtendedProtectionPolicyTypeConverter.cs
- SplayTreeNode.cs
- ComPlusInstanceContextInitializer.cs
- MasterPageCodeDomTreeGenerator.cs
- MetadataExporter.cs
- safelink.cs
- DllHostedComPlusServiceHost.cs
- AttributeUsageAttribute.cs
- InfocardExtendedInformationCollection.cs
- Exceptions.cs
- GlobalizationAssembly.cs
- UrlEncodedParameterWriter.cs
- InternalBufferOverflowException.cs
- ObjectStateFormatter.cs
- XmlCustomFormatter.cs
- TimeSpanParse.cs
- GenerateTemporaryTargetAssembly.cs
- ElementHost.cs
- PipelineModuleStepContainer.cs
- DataSourceCache.cs
- InheritanceContextHelper.cs
- LabelDesigner.cs
- StructuralType.cs
- BitmapEncoder.cs
- SignerInfo.cs
- Vector3DCollectionValueSerializer.cs
- DesignerToolboxInfo.cs
- Soap.cs
- SymbolMethod.cs
- Random.cs
- ping.cs
- BitmapSource.cs
- MaskedTextBoxDesignerActionList.cs
- FixedSOMGroup.cs
- SqlExpressionNullability.cs
- CompositeDesignerAccessibleObject.cs
- AccessibleObject.cs
- exports.cs
- Bold.cs
- InputGestureCollection.cs
- BinaryObjectWriter.cs
- IgnorePropertiesAttribute.cs
- FullTextState.cs
- LocalizableAttribute.cs
- ObjectTypeMapping.cs
- ServerValidateEventArgs.cs
- ValidationPropertyAttribute.cs
- TextElement.cs
- CanonicalFontFamilyReference.cs
- ParentQuery.cs
- ProfileBuildProvider.cs
- ReadOnlyObservableCollection.cs
- ExpressionContext.cs
- UserPreferenceChangedEventArgs.cs
- DataView.cs
- AssociationSet.cs
- FragmentQueryKB.cs
- AnnotationObservableCollection.cs
- AutomationFocusChangedEventArgs.cs
- HitTestWithGeometryDrawingContextWalker.cs
- XpsColorContext.cs
- DataRelation.cs
- DrawingContext.cs
- Component.cs
- CommandValueSerializer.cs
- ToolStripPanel.cs
- AssociationTypeEmitter.cs
- SettingsPropertyWrongTypeException.cs
- SoapCodeExporter.cs
- GrammarBuilderDictation.cs
- ProfileService.cs
- PerformanceCounter.cs
- WpfKnownMemberInvoker.cs
- mediaeventshelper.cs
- NonParentingControl.cs
- PixelFormatConverter.cs
- SimpleFieldTemplateUserControl.cs
- ToolboxBitmapAttribute.cs
- DragEvent.cs
- AbsoluteQuery.cs
- StrokeNodeEnumerator.cs
- QualifiedCellIdBoolean.cs
- OdbcUtils.cs
- RegisterInfo.cs
- ContextBase.cs
- AuthenticationConfig.cs
- ImageCodecInfo.cs
- ServiceNameCollection.cs
- ResolveDuplexAsyncResult.cs
- GenericEnumerator.cs
- OdbcParameter.cs
- Light.cs
- NonParentingControl.cs
- ProfilePropertyNameValidator.cs
- WizardStepBase.cs
- GlyphShapingProperties.cs
- ObjectListItemCollection.cs
- ResourceDefaultValueAttribute.cs
- InOutArgumentConverter.cs
- HtmlTable.cs