Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / MetadataPropertyAttribute.cs / 1305376 / MetadataPropertyAttribute.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Attribute used to mark up properties that should appear in the MetadataItem.MetadataProperties collection /// [AttributeUsage(AttributeTargets.Property, AllowMultiple = false, Inherited = false)] internal sealed class MetadataPropertyAttribute : Attribute { ////// Initializes a new attribute with built in type kind /// /// Built in type setting Type property /// Sets IsCollectionType property internal MetadataPropertyAttribute(BuiltInTypeKind builtInTypeKind, bool isCollectionType) : this(MetadataItem.GetBuiltInType(builtInTypeKind), isCollectionType) { } ////// Initializes a new attribute with primitive type kind /// /// Primitive type setting Type property /// Sets IsCollectionType property internal MetadataPropertyAttribute(PrimitiveTypeKind primitiveTypeKind, bool isCollectionType) : this(MetadataItem.EdmProviderManifest.GetPrimitiveType(primitiveTypeKind), isCollectionType) { } ////// Initialize a new attribute with complex type kind (corresponding the the CLR type) /// /// CLR type setting Type property /// Sets IsCollectionType property internal MetadataPropertyAttribute(Type type, bool isCollection) : this(ClrComplexType.CreateReadonlyClrComplexType(type, type.Namespace ?? string.Empty, type.Name), isCollection) { } ////// Initialize a new attribute /// /// Sets Type property /// Sets IsCollectionType property private MetadataPropertyAttribute(EdmType type, bool isCollectionType) { Debug.Assert(null != type); _type = type; _isCollectionType = isCollectionType; } private readonly EdmType _type; private readonly bool _isCollectionType; ////// Gets EDM type for values stored in property. /// internal EdmType Type { get { return _type; } } ////// Gets bool indicating whether this is a collection type. /// internal bool IsCollectionType { get { return _isCollectionType; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
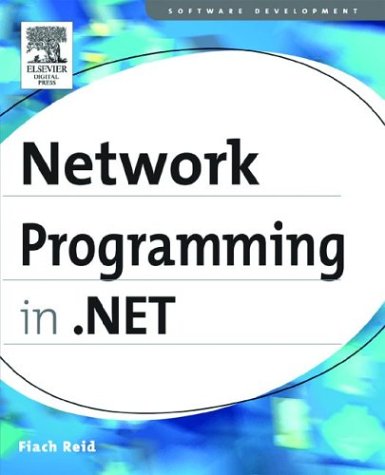
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KeyNotFoundException.cs
- ControlDesigner.cs
- AppDomainProtocolHandler.cs
- ResourceManager.cs
- TransportOutputChannel.cs
- SmtpSection.cs
- StylusShape.cs
- DataTableMapping.cs
- ServiceEndpointAssociationProvider.cs
- XamlBrushSerializer.cs
- TableLayoutRowStyleCollection.cs
- Peer.cs
- ReadOnlyDataSourceView.cs
- MaterialCollection.cs
- StorageComplexTypeMapping.cs
- ClaimSet.cs
- MSAANativeProvider.cs
- RelationshipNavigation.cs
- WebPartsSection.cs
- TrackingStringDictionary.cs
- OleDbMetaDataFactory.cs
- returneventsaver.cs
- ArgIterator.cs
- SQLCharsStorage.cs
- NavigationEventArgs.cs
- PermissionListSet.cs
- TextBox.cs
- TranslateTransform3D.cs
- EntitySqlQueryCacheKey.cs
- AccessViolationException.cs
- TextEndOfParagraph.cs
- NetDataContractSerializer.cs
- TempEnvironment.cs
- CodeParameterDeclarationExpression.cs
- ContextMenuAutomationPeer.cs
- PingReply.cs
- NumericUpDownAcceleration.cs
- assemblycache.cs
- NetworkInformationException.cs
- WorkflowInstanceSuspendedRecord.cs
- IntegerValidatorAttribute.cs
- MasterPageBuildProvider.cs
- SrgsToken.cs
- UnionCodeGroup.cs
- SafeNativeMethods.cs
- UnauthorizedAccessException.cs
- MimeImporter.cs
- ScriptControlDescriptor.cs
- Rfc4050KeyFormatter.cs
- Buffer.cs
- WebPartConnectionsCancelEventArgs.cs
- XmlAttributeProperties.cs
- MediaTimeline.cs
- GridViewHeaderRowPresenter.cs
- KeyboardDevice.cs
- Interlocked.cs
- EmissiveMaterial.cs
- Italic.cs
- PriorityRange.cs
- StringUtil.cs
- EntitySetBase.cs
- HttpCapabilitiesBase.cs
- ExpandoClass.cs
- DebugInfoGenerator.cs
- _Events.cs
- Int64AnimationBase.cs
- Invariant.cs
- StylusEventArgs.cs
- TypeHelpers.cs
- ZipIOCentralDirectoryFileHeader.cs
- IFormattable.cs
- ExtensibleClassFactory.cs
- ServiceDescriptionData.cs
- SqlCharStream.cs
- XmlEntity.cs
- ProviderException.cs
- SiteMapSection.cs
- SafeMILHandleMemoryPressure.cs
- FormViewRow.cs
- Certificate.cs
- CategoryNameCollection.cs
- DataTableReaderListener.cs
- ProfilePropertySettingsCollection.cs
- SecurityDescriptor.cs
- DataReceivedEventArgs.cs
- MeasureItemEvent.cs
- DirectoryGroupQuery.cs
- XhtmlBasicTextBoxAdapter.cs
- MouseActionConverter.cs
- ToolStripDropDownMenu.cs
- StringResourceManager.cs
- WinFormsSecurity.cs
- TaskSchedulerException.cs
- StylusPointPropertyUnit.cs
- Container.cs
- CqlLexer.cs
- EncryptedReference.cs
- filewebrequest.cs
- IQueryable.cs
- OperatingSystem.cs