Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / GeneratedView.cs / 1305376 / GeneratedView.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Common.CommandTrees; using System.Data.Common.EntitySql; using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Utils; using System.Diagnostics; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Query.InternalTrees; using System.Data.Query.PlanCompiler; namespace System.Data.Mapping.ViewGeneration { // Holds the view generated for a given OFTYPE(Extent, Type) combination internal class GeneratedView : InternalBase { // effects: Creates an object that stores a view, cqlString, for the combinationinternal GeneratedView(EntitySetBase extent, EdmType type, string cqlString, StorageMappingItemCollection mappingItemCollection, ConfigViewGenerator config) { m_extent = extent; m_type = type; m_cqlString = cqlString; m_config = config; m_mappingItemCollection = mappingItemCollection; if (m_config.IsViewTracing) { if (EqualityComparer .Default.Equals(type, extent.ElementType)) { Helpers.FormatTraceLine("CQL view for {0}", extent.Name); } else { Helpers.FormatTraceLine("CQL view for OFTYPE({0}, {1}.{2})", extent.Name, type.NamespaceName, type.Name); } Helpers.StringTraceLine(cqlString); } if (m_config.IsViewTracing) { ParseView(m_mappingItemCollection, false /*isUserSpecified*/); } } internal GeneratedView(EntitySetBase extent, EdmType type, DbQueryCommandTree commandTree, StorageMappingItemCollection mappingItemCollection, ConfigViewGenerator config) { m_extent = extent; m_type = type; m_cqlString = "Foreign Key Association Set View"; m_commandTree = commandTree; m_config = config; m_mappingItemCollection = mappingItemCollection; } #region Fields private EntitySetBase m_extent; private EdmType m_type; private string m_cqlString; private DbQueryCommandTree m_commandTree; //We cache CQTs for Update Views sicne that is the one update stack works of. private Node m_internalTreeNode; //we cache IQTs for Query Views since that is the one query stack works of. private ConfigViewGenerator m_config; private DiscriminatorMap m_discriminatorMap; private StorageMappingItemCollection m_mappingItemCollection; #endregion #region Properties internal EntitySetBase Extent { get { return m_extent; } } internal EdmType EntityType { get { return m_type; } } internal string CqlString { get { return m_cqlString; } } internal DiscriminatorMap DiscriminatorMap { get { return m_discriminatorMap; } } #endregion #region Methods /// /// Parses and validates a user-defined query mapping view. Outputs errors if any. /// internal IEnumerableParseUserSpecifiedView(StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { m_commandTree = ParseView(m_mappingItemCollection, true); // Verify that all expressions appearing in the view are supported. foreach (var error in ViewValidator.ValidateQueryView(m_commandTree, setMapping, elementType, includeSubtypes)) { yield return error; } // Verify that the result type of the query view is assignable to the element type of the entityset CollectionType queryResultType = (m_commandTree.Query.ResultType.EdmType) as CollectionType; if ((queryResultType == null) || (!setMapping.Set.ElementType.IsAssignableFrom(queryResultType.TypeUsage.EdmType))) { EdmSchemaError error = new EdmSchemaError(System.Data.Entity.Strings.Mapping_Invalid_QueryView_Type_1(setMapping.Set.Name), (int)StorageMappingErrorCode.InvalidQueryViewResultType, EdmSchemaErrorSeverity.Error, setMapping.EntityContainerMapping.SourceLocation, setMapping.StartLineNumber, setMapping.StartLinePosition); yield return error; } } // effects: Given an extent and its corresponding view, invokes the // parser to check if the view definition is syntactically correct // Returns true iff the parsing succeeds private DbQueryCommandTree ParseView(StorageMappingItemCollection mappingItemCollection, bool isUserSpecified) { // We do not catch any internal exceptions any more m_config.StartSingleWatch(PerfType.ViewParsing); //If it is a user specified view, //allow all queries. Otherwise parse the view in a restricted mode. ParserOptions.CompilationMode compilationMode = ParserOptions.CompilationMode.RestrictedViewGenerationMode; if (isUserSpecified) { compilationMode = ParserOptions.CompilationMode.UserViewGenerationMode; } DbQueryCommandTree commandTree = (DbQueryCommandTree)ExternalCalls.CompileView(CqlString, mappingItemCollection, compilationMode); // For non user-specified views, perform simplification if (!isUserSpecified) { commandTree = ViewSimplifier.SimplifyView(m_extent, commandTree); } // See if the view matches the "discriminated" pattern (allows simplification of generated store commands) if (m_extent.BuiltInTypeKind == BuiltInTypeKind.EntitySet) { DiscriminatorMap discriminatorMap; if (DiscriminatorMap.TryCreateDiscriminatorMap((EntitySet)m_extent, commandTree.Query, out discriminatorMap)) { m_discriminatorMap = discriminatorMap; } } m_config.StopSingleWatch(PerfType.ViewParsing); return commandTree; } internal DbQueryCommandTree GetCommandTree(MetadataWorkspace workspace) { //Ask command trees only for C space entity sets Debug.Assert(m_extent.EntityContainer.DataSpace == DataSpace.SSpace, "CQT should be asked only for update view"); if (m_commandTree == null) { m_commandTree = ParseView((StorageMappingItemCollection)workspace.GetItemCollection(DataSpace.CSSpace), false); } return m_commandTree; } internal Node GetInternalTree(MetadataWorkspace workspace) { Debug.Assert(m_extent.EntityContainer.DataSpace == DataSpace.CSpace, "Internal Tree should be asked only for query view"); if (m_internalTreeNode == null) { DbQueryCommandTree tree = m_commandTree ?? ParseView((StorageMappingItemCollection)workspace.GetItemCollection(DataSpace.CSSpace), false); // Convert this into an ITree first Command itree = ITreeGenerator.Generate(tree, this.DiscriminatorMap); // Pull out the root physical project-op, and copy this itree into our own itree PlanCompiler.Assert(itree.Root.Op.OpType == OpType.PhysicalProject, "Expected a physical projectOp at the root of the tree - found " + itree.Root.Op.OpType); // #554756: VarVec enumerators are not cached on the shared Command instance. itree.DisableVarVecEnumCaching(); m_internalTreeNode = itree.Root.Child0; } return m_internalTreeNode; } #endregion #region String Methods internal override void ToCompactString(StringBuilder builder) { builder.Append("OFTYPE(") .Append(Extent.Name) .Append(", ") .Append(EntityType.Name) .Append(") = ") .Append(CqlString); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
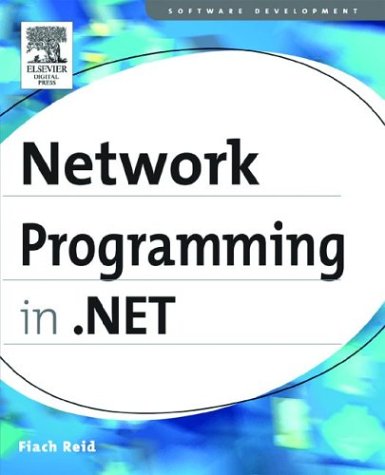
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommonDialog.cs
- HtmlControl.cs
- PageThemeBuildProvider.cs
- HotSpotCollectionEditor.cs
- OleDbRowUpdatingEvent.cs
- PrintingPermissionAttribute.cs
- TextSegment.cs
- ACE.cs
- DateTimePickerDesigner.cs
- Crypto.cs
- WindowsRegion.cs
- AppDomainManager.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- AppDomainAttributes.cs
- Exception.cs
- TransactionFlowElement.cs
- DataPagerField.cs
- TextBoxAutomationPeer.cs
- XmlSchemaValidationException.cs
- NetNamedPipeBindingCollectionElement.cs
- ValidationResult.cs
- EpmSourcePathSegment.cs
- DataFormats.cs
- DbDataReader.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- TextAnchor.cs
- Bind.cs
- PieceNameHelper.cs
- InvalidCastException.cs
- ImportContext.cs
- Matrix.cs
- TreeNodeSelectionProcessor.cs
- ClockGroup.cs
- ExtensibleClassFactory.cs
- UpdatePanelTriggerCollection.cs
- CacheHelper.cs
- RadioButton.cs
- PropertyChangedEventManager.cs
- ImageBrush.cs
- SqlBulkCopyColumnMapping.cs
- SortedList.cs
- ParagraphResult.cs
- SqlDataSourceConfigureFilterForm.cs
- TextWriterTraceListener.cs
- BamlResourceContent.cs
- DirtyTextRange.cs
- TextWriterTraceListener.cs
- TextEditor.cs
- ValueProviderWrapper.cs
- Schema.cs
- DisplayNameAttribute.cs
- OpenTypeLayout.cs
- UriParserTemplates.cs
- FontNameEditor.cs
- WebPartTransformerCollection.cs
- ConfigurationValidatorBase.cs
- TextEvent.cs
- SslStream.cs
- Padding.cs
- IisTraceListener.cs
- ZipIOLocalFileHeader.cs
- webbrowsersite.cs
- DatePickerAutomationPeer.cs
- XmlIgnoreAttribute.cs
- InputScopeConverter.cs
- GridView.cs
- XmlSchemaIdentityConstraint.cs
- TraceFilter.cs
- JumpPath.cs
- SqlUtils.cs
- ModelItemKeyValuePair.cs
- MachineKeySection.cs
- FixedPosition.cs
- X509ClientCertificateCredentialsElement.cs
- WindowHideOrCloseTracker.cs
- SystemShuttingDownException.cs
- XpsDocumentEvent.cs
- CustomTypeDescriptor.cs
- WorkflowLayouts.cs
- StrokeIntersection.cs
- QuaternionKeyFrameCollection.cs
- XamlTreeBuilder.cs
- X500Name.cs
- TypeGenericEnumerableViewSchema.cs
- CompilationLock.cs
- CipherData.cs
- MenuScrollingVisibilityConverter.cs
- ApplicationDirectoryMembershipCondition.cs
- AsymmetricAlgorithm.cs
- Stack.cs
- GraphicsContext.cs
- AppearanceEditorPart.cs
- SspiSecurityTokenParameters.cs
- XmlNamedNodeMap.cs
- wmiprovider.cs
- ZipIORawDataFileBlock.cs
- ValidationSummary.cs
- XmlReader.cs
- RegistryPermission.cs
- DataTemplateKey.cs