Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / Generic / ICollection.cs / 1305376 / ICollection.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Interface: ICollection ** **[....] ** ** ** Purpose: Base interface for all generic collections. ** ** ===========================================================*/ namespace System.Collections.Generic { using System; using System.Runtime.CompilerServices; using System.Diagnostics.Contracts; // Base interface for all collections, defining enumerators, size, and // synchronization methods. // Note that T[] : IList, and we want to ensure that if you use // IList , we ensure a YourValueType[] can be used // without jitting. Hence the TypeDependencyAttribute on SZArrayHelper. // This is a special hack internally though - see VM\compile.cpp. // The same attribute is on IEnumerable and ICollection . [ContractClass(typeof(ICollectionContract<>))] [TypeDependencyAttribute("System.SZArrayHelper")] public interface ICollection : IEnumerable { // Number of items in the collections. int Count { get; } bool IsReadOnly { get; } void Add(T item); void Clear(); bool Contains(T item); // CopyTo copies a collection into an Array, starting at a particular // index into the array. // void CopyTo(T[] array, int arrayIndex); //void CopyTo(int sourceIndex, T[] destinationArray, int destinationIndex, int count); bool Remove(T item); } [ContractClassFor(typeof(ICollection<>))] internal class ICollectionContract : ICollection { int ICollection .Count { get { Contract.Ensures(Contract.Result () >= 0); return default(int); } } bool ICollection .IsReadOnly { get { return default(bool); } } void ICollection .Add(T item) { //Contract.Ensures(((ICollection )this).Count == Contract.OldValue(((ICollection )this).Count) + 1); // not threadsafe } void ICollection .Clear() { } bool ICollection .Contains(T item) { return default(bool); } void ICollection .CopyTo(T[] array, int arrayIndex) { } bool ICollection .Remove(T item) { return default(bool); } IEnumerator IEnumerable .GetEnumerator() { Contract.Ensures(Contract.Result >() != null); return default(IEnumerator ); } IEnumerator IEnumerable.GetEnumerator() { Contract.Ensures(Contract.Result () != null); return default(IEnumerator); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
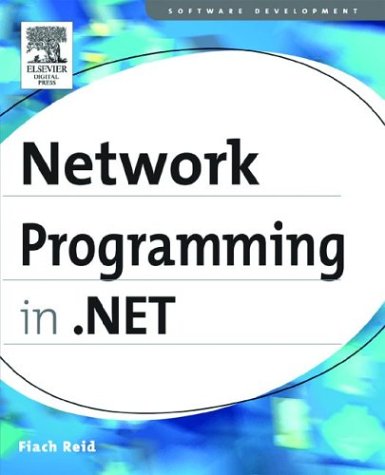
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityProxyFactory.cs
- SeekStoryboard.cs
- InstalledFontCollection.cs
- ReliableChannelFactory.cs
- ClientRuntimeConfig.cs
- BypassElement.cs
- unsafenativemethodsother.cs
- ExpressionNode.cs
- TextAnchor.cs
- PagerSettings.cs
- FormViewRow.cs
- _BufferOffsetSize.cs
- CredentialCache.cs
- ResourceWriter.cs
- DataServiceCollectionOfT.cs
- XmlSchemaSimpleTypeList.cs
- PropertyGrid.cs
- OutputCacheSection.cs
- PersistenceProviderDirectory.cs
- CallbackTimeoutsElement.cs
- BamlWriter.cs
- AttributeExtensions.cs
- SuspendDesigner.cs
- ReferentialConstraintRoleElement.cs
- PrePostDescendentsWalker.cs
- CacheRequest.cs
- InputLanguageEventArgs.cs
- DataGridViewCellStyle.cs
- EntityDesignerBuildProvider.cs
- DefaultBinder.cs
- ImageDrawing.cs
- Compiler.cs
- ScopelessEnumAttribute.cs
- SafeEventLogWriteHandle.cs
- ParameterCollectionEditor.cs
- FileUtil.cs
- CapabilitiesPattern.cs
- dataprotectionpermission.cs
- EditorPartChrome.cs
- KoreanCalendar.cs
- ServiceBehaviorElementCollection.cs
- ECDiffieHellmanCngPublicKey.cs
- ArithmeticLiteral.cs
- ObjectDisposedException.cs
- TextPattern.cs
- ExpressionBindings.cs
- IdentitySection.cs
- MimePart.cs
- Int32Rect.cs
- CodeFieldReferenceExpression.cs
- ExpressionBindings.cs
- UriSection.cs
- DeflateStream.cs
- ListViewCommandEventArgs.cs
- SudsWriter.cs
- OleDbConnection.cs
- X509CertificateCollection.cs
- XmlCharacterData.cs
- ISAPIRuntime.cs
- GraphicsContainer.cs
- GACIdentityPermission.cs
- NotEqual.cs
- ColorDialog.cs
- XPathExpr.cs
- DataConnectionHelper.cs
- LateBoundChannelParameterCollection.cs
- BasicSecurityProfileVersion.cs
- BitmapPalette.cs
- VirtualDirectoryMapping.cs
- ServiceDeploymentInfo.cs
- ServerValidateEventArgs.cs
- DropShadowEffect.cs
- SourceFilter.cs
- TableLayoutStyleCollection.cs
- UnauthorizedWebPart.cs
- WindowsScrollBar.cs
- RecordConverter.cs
- RowParagraph.cs
- GlyphRunDrawing.cs
- ToolStripDropDownButton.cs
- Delegate.cs
- MetadataHelper.cs
- DeleteMemberBinder.cs
- KeyValueConfigurationElement.cs
- WinEventHandler.cs
- PreviousTrackingServiceAttribute.cs
- HttpResponseInternalWrapper.cs
- SafeIUnknown.cs
- EntitySetBaseCollection.cs
- SafeThemeHandle.cs
- CultureSpecificCharacterBufferRange.cs
- EntityStoreSchemaFilterEntry.cs
- HebrewNumber.cs
- SqlUtils.cs
- RegexTree.cs
- FileDetails.cs
- TraceContextEventArgs.cs
- GridSplitter.cs
- BaseTemplateBuildProvider.cs
- AnnotationService.cs