Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Serializer / PrimitiveCodeDomSerializer.cs / 1305376 / PrimitiveCodeDomSerializer.cs
namespace System.Workflow.ComponentModel.Serialization { using System; using System.CodeDom; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Resources; using System.Workflow.ComponentModel.Design; using System.Collections.Generic; using Microsoft.CSharp; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Compiler; using System.CodeDom.Compiler; using System.IO; using System.Reflection; using System.Diagnostics; #region Class PrimitiveCodeDomSerializer // work around : PD7's PrimitiveCodeDomSerializer does not handle well strings bigger than 200 characters, // we push our own version to fix it. internal class PrimitiveCodeDomSerializer : CodeDomSerializer { private static readonly string JSharpFileExtension = ".jsl"; private static PrimitiveCodeDomSerializer defaultSerializer; internal static PrimitiveCodeDomSerializer Default { get { if (defaultSerializer == null) { defaultSerializer = new PrimitiveCodeDomSerializer(); } return defaultSerializer; } } public override object Serialize(IDesignerSerializationManager manager, object value) { CodeExpression expression = new CodePrimitiveExpression(value); if (value == null || value is bool || value is char || value is int || value is float || value is double) { // work aroundf for J#, since they don't support auto-boxing of value types yet. CodeDomProvider codeProvider = manager.GetService(typeof(CodeDomProvider)) as CodeDomProvider; if (codeProvider != null && String.Equals(codeProvider.FileExtension, JSharpFileExtension)) { // See if we are boxing - if so, insert a cast. ExpressionContext cxt = manager.Context[typeof(ExpressionContext)] as ExpressionContext; //Debug.Assert(cxt != null, "No expression context on stack - J# boxing cast will not be inserted"); if (cxt != null) { if (cxt.ExpressionType == typeof(object)) { expression = new CodeCastExpression(value.GetType(), expression); expression.UserData.Add("CastIsBoxing", true); } } } return expression; } String stringValue = value as string; if (stringValue != null) { // WinWS: The commented code breaks us when we have long strings //if (stringValue.Length > 200) //{ // return SerializeToResourceExpression(manager, stringValue); //} //else return expression; } // generate a cast for non-int types because we won't parse them properly otherwise because we won't know to convert // them to the narrow form. // return new CodeCastExpression(new CodeTypeReference(value.GetType()), expression); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
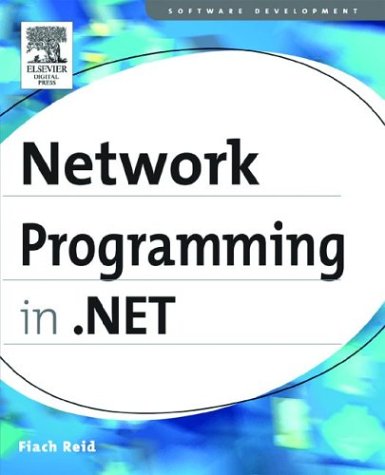
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectDataSourceStatusEventArgs.cs
- MessageEncodingBindingElementImporter.cs
- HttpProfileBase.cs
- WebEventTraceProvider.cs
- ButtonPopupAdapter.cs
- FormsIdentity.cs
- WbmpConverter.cs
- XmlSecureResolver.cs
- DataGridViewCellConverter.cs
- CodeNamespace.cs
- FixedSOMElement.cs
- PropertyGroupDescription.cs
- Assert.cs
- XamlFrame.cs
- Baml2006ReaderFrame.cs
- FullTextBreakpoint.cs
- NativeActivityAbortContext.cs
- IgnoreSectionHandler.cs
- TextMarkerSource.cs
- StorageComplexPropertyMapping.cs
- PageCanvasSize.cs
- sitestring.cs
- BookmarkWorkItem.cs
- RunClient.cs
- MaskDescriptor.cs
- GridViewCellAutomationPeer.cs
- TrackBar.cs
- OpacityConverter.cs
- PagedDataSource.cs
- TransformGroup.cs
- WrapperEqualityComparer.cs
- HttpServerVarsCollection.cs
- TargetParameterCountException.cs
- BlockUIContainer.cs
- Pen.cs
- ExceptionHandlerDesigner.cs
- DataGridViewCellMouseEventArgs.cs
- CompilerState.cs
- AmbientLight.cs
- PingOptions.cs
- InstanceDataCollection.cs
- FixedHyperLink.cs
- ToolStripItemTextRenderEventArgs.cs
- TreeViewImageKeyConverter.cs
- ExtentJoinTreeNode.cs
- XmlSerializableWriter.cs
- RadioButtonFlatAdapter.cs
- RadioButton.cs
- designeractionbehavior.cs
- Page.cs
- StyleHelper.cs
- FrameAutomationPeer.cs
- CachingParameterInspector.cs
- TextTreeDeleteContentUndoUnit.cs
- CollectionDataContract.cs
- AutoScrollExpandMessageFilter.cs
- IncrementalReadDecoders.cs
- TreeViewEvent.cs
- OrthographicCamera.cs
- FileSecurity.cs
- SimpleTypeResolver.cs
- ImageList.cs
- HostProtectionPermission.cs
- _FtpDataStream.cs
- log.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- ClientConfigurationHost.cs
- HttpClientCertificate.cs
- CharConverter.cs
- MachineKeySection.cs
- SamlSubjectStatement.cs
- StateWorkerRequest.cs
- StringInfo.cs
- RegisteredScript.cs
- ModelTreeEnumerator.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- DbgUtil.cs
- ForwardPositionQuery.cs
- RoutedEventConverter.cs
- PersistChildrenAttribute.cs
- WorkflowDesignerColors.cs
- InputReportEventArgs.cs
- SafeRightsManagementHandle.cs
- ProxyWebPartManagerDesigner.cs
- LoginUtil.cs
- DataGridViewHeaderCell.cs
- ConfigsHelper.cs
- FormsAuthentication.cs
- DocumentApplicationJournalEntry.cs
- SecurityElement.cs
- LingerOption.cs
- AsyncSerializedWorker.cs
- DataBindingList.cs
- VsPropertyGrid.cs
- ReaderOutput.cs
- SapiRecoContext.cs
- Binding.cs
- SecurityIdentifierElementCollection.cs
- DocumentPageTextView.cs
- ListSortDescriptionCollection.cs