Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / WasHosting / System / ServiceModel / WasHosting / BaseProcessProtocolHandler.cs / 1305376 / BaseProcessProtocolHandler.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.WasHosting { using System; using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.ServiceModel; using System.Web; using System.Web.Hosting; using System.ServiceModel.Channels; using System.ServiceModel.Activation; using System.Runtime; abstract class BaseProcessProtocolHandler : ProcessProtocolHandler { string protocolId; IAdphManager adphManager; // Mapping from listenerChannelId->listenerChannelContext (i.e. IAdphManager, appId) DictionarylistenerChannelIdMapping = new Dictionary (); [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.AvoidUncalledPrivateCode, Justification = "Instantiated by ASP.NET")] protected BaseProcessProtocolHandler(string protocolId) : base() { this.protocolId = protocolId; } internal virtual void HandleStartListenerChannelError(IListenerChannelCallback listenerChannelCallback, Exception ex) { // This is the workaround to let WAS know that the LC is started and then gracefully stopped. listenerChannelCallback.ReportStarted(); listenerChannelCallback.ReportStopped(0); } // Start per-process listening for messages public override void StartListenerChannel(IListenerChannelCallback listenerChannelCallback, IAdphManager adphManager) { DiagnosticUtility.DebugAssert(listenerChannelCallback != null, "The listenerChannelCallback parameter must not be null"); DiagnosticUtility.DebugAssert(adphManager != null, "The adphManager parameter must not be null"); int channelId = listenerChannelCallback.GetId(); ListenerChannelContext listenerChannelContext; lock (this.listenerChannelIdMapping) { if (!listenerChannelIdMapping.TryGetValue(channelId, out listenerChannelContext)) { int listenerChannelDataLength = listenerChannelCallback.GetBlobLength(); byte[] listenerChannelData = new byte[listenerChannelDataLength]; listenerChannelCallback.GetBlob(listenerChannelData, ref listenerChannelDataLength); Debug.Print("BaseProcessProtocolHandler.StartListenerChannel() GetBlob() contains " + listenerChannelDataLength + " bytes"); listenerChannelContext = ListenerChannelContext.Hydrate(listenerChannelData); this.listenerChannelIdMapping.Add(channelId, listenerChannelContext); Debug.Print("BaseProcessProtocolHandler.StartListenerChannel() listenerChannelContext.ListenerChannelId: " + listenerChannelContext.ListenerChannelId); } } if (this.adphManager == null) { this.adphManager = adphManager; } try { // wether or not a previous AppDomain was running, we're going to start a new one now: Debug.Print("BaseProcessProtocolHandler.StartListenerChannel() calling StartAppDomainProtocolListenerChannel(appKey:" + listenerChannelContext.AppKey + " protocolId:" + protocolId + ")"); adphManager.StartAppDomainProtocolListenerChannel(listenerChannelContext.AppKey, protocolId, listenerChannelCallback); } catch (Exception ex) { if (Fx.IsFatal(ex)) { throw; } if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.ExceptionUtility.TraceHandledException(ex, TraceEventType.Error); } HandleStartListenerChannelError(listenerChannelCallback, ex); } } public override void StopProtocol(bool immediate) { Debug.Print("BaseProcessProtocolHandler.StopProtocol(protocolId:" + protocolId + ", immediate:" + immediate + ")"); } public override void StopListenerChannel(int listenerChannelId, bool immediate) { Debug.Print("BaseProcessProtocolHandler.StopListenerChannel(protocolId:" + protocolId + ", listenerChannelId:" + listenerChannelId + ", immediate:" + immediate + ")"); ListenerChannelContext listenerChannelContext = this.listenerChannelIdMapping[listenerChannelId]; adphManager.StopAppDomainProtocolListenerChannel(listenerChannelContext.AppKey, protocolId, listenerChannelId, immediate); lock (this.listenerChannelIdMapping) { // Remove the channel id. this.listenerChannelIdMapping.Remove(listenerChannelId); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
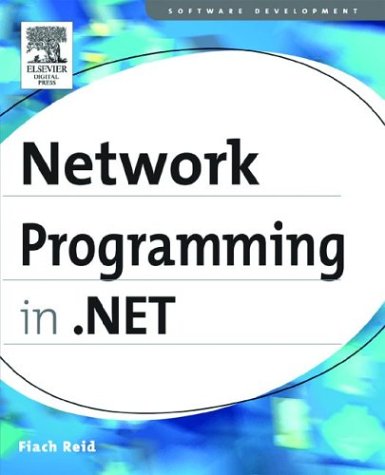
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseTemplateParser.cs
- FormatVersion.cs
- _AutoWebProxyScriptEngine.cs
- ObjectItemLoadingSessionData.cs
- ShaderEffect.cs
- PointAnimationBase.cs
- ProviderConnectionPointCollection.cs
- basenumberconverter.cs
- EncoderFallback.cs
- ClientBuildManager.cs
- TreeViewEvent.cs
- RichTextBoxConstants.cs
- IndependentlyAnimatedPropertyMetadata.cs
- XmlSignatureProperties.cs
- WebPartVerb.cs
- PerformanceCounterPermissionEntry.cs
- SoapSchemaMember.cs
- XmlNodeReader.cs
- FocusWithinProperty.cs
- SetState.cs
- GcHandle.cs
- DrawListViewColumnHeaderEventArgs.cs
- Gdiplus.cs
- LogicalExpr.cs
- XappLauncher.cs
- RemotingAttributes.cs
- SoapConverter.cs
- SchemaInfo.cs
- StateMachine.cs
- DrawingGroupDrawingContext.cs
- PropertyChangingEventArgs.cs
- VariableBinder.cs
- ProjectionRewriter.cs
- SqlInfoMessageEvent.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- GacUtil.cs
- SqlCacheDependencyDatabase.cs
- SqlMultiplexer.cs
- ExtendedProtectionPolicyElement.cs
- OleDbConnectionFactory.cs
- Int32AnimationUsingKeyFrames.cs
- ExpressionBuilder.cs
- IPAddressCollection.cs
- ToolStripControlHost.cs
- NativeCppClassAttribute.cs
- EntityCommandDefinition.cs
- SerializationStore.cs
- DataSourceControl.cs
- ScriptHandlerFactory.cs
- DragDeltaEventArgs.cs
- CustomErrorCollection.cs
- NameValuePair.cs
- StrokeNode.cs
- Bits.cs
- AutomationProperty.cs
- OuterProxyWrapper.cs
- CaseInsensitiveHashCodeProvider.cs
- FileStream.cs
- InkCanvasInnerCanvas.cs
- CheckBoxBaseAdapter.cs
- OdbcConnectionPoolProviderInfo.cs
- CodeEntryPointMethod.cs
- PerformanceCounterNameAttribute.cs
- WebBrowserPermission.cs
- XLinq.cs
- SequentialActivityDesigner.cs
- AdPostCacheSubstitution.cs
- IntSecurity.cs
- cookieexception.cs
- RubberbandSelector.cs
- Types.cs
- TypeConverterBase.cs
- SessionPageStateSection.cs
- RelationshipEndCollection.cs
- EventToken.cs
- DesignerSerializerAttribute.cs
- UmAlQuraCalendar.cs
- ParameterSubsegment.cs
- SubqueryTrackingVisitor.cs
- MinMaxParagraphWidth.cs
- DocumentPage.cs
- ProtectedConfiguration.cs
- SendContent.cs
- TextSimpleMarkerProperties.cs
- ItemCollection.cs
- SpellCheck.cs
- DataIdProcessor.cs
- NullExtension.cs
- CodeSnippetStatement.cs
- StrokeCollection2.cs
- ActiveDocumentEvent.cs
- WorkflowWebHostingModule.cs
- CollectionViewSource.cs
- CaseInsensitiveOrdinalStringComparer.cs
- CurrencyWrapper.cs
- DoWorkEventArgs.cs
- StringFormat.cs
- CheckoutException.cs
- GridSplitter.cs
- MouseActionValueSerializer.cs