Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Log / System / IO / Log / FileRecordSequenceCompletedAsyncResult.cs / 1305376 / FileRecordSequenceCompletedAsyncResult.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IO.Log { using System; using System.Diagnostics; using System.Runtime; using System.Threading; enum Work { Append, Flush, ReserveAndAppend, WriteRestartArea } sealed class FileRecordSequenceCompletedAsyncResult : IAsyncResult { SequenceNumber result; object userState; AsyncCallback callback; bool endCalled; Work work; object syncRoot; ManualResetEvent waitHandle; public FileRecordSequenceCompletedAsyncResult( SequenceNumber result, AsyncCallback callback, object userState, Work work) { this.result = result; this.callback = callback; this.userState = userState; this.work = work; this.syncRoot = new object(); if (this.callback != null) { try { this.callback(this); } #pragma warning suppress 56500 // This is a callback exception catch(Exception e) { if (Fx.IsFatal(e)) throw; throw DiagnosticUtility.ExceptionUtility.ThrowHelperCallback(e); } } } public Object AsyncState { get { return this.userState; } } public WaitHandle AsyncWaitHandle { get { lock(this.syncRoot) { // We won't ever close it (it must be GC'd instead), but try // not to be too excessive in allocations. // if (this.waitHandle == null) this.waitHandle = new ManualResetEvent(true); } return this.waitHandle; } } public bool CompletedSynchronously { get { return true; } } public Work CompletedWork { get { return this.work; } } public bool IsCompleted { get { return true; } } internal SequenceNumber End() { if (this.endCalled) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.DuplicateEnd()); } this.endCalled = true; return this.result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
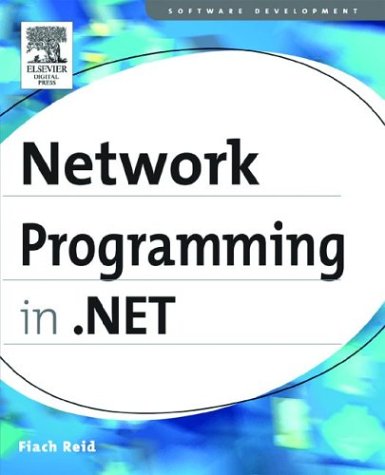
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XslException.cs
- DeploymentSection.cs
- listitem.cs
- ToolboxSnapDragDropEventArgs.cs
- Context.cs
- NumberFormatInfo.cs
- ValidationErrorEventArgs.cs
- WindowsGraphicsCacheManager.cs
- FillRuleValidation.cs
- AmbientValueAttribute.cs
- BuildProvidersCompiler.cs
- ViewGenerator.cs
- CustomExpressionEventArgs.cs
- Effect.cs
- TreeViewBindingsEditor.cs
- X509CertificateValidationMode.cs
- ProfileManager.cs
- StorageInfo.cs
- BamlRecords.cs
- ParagraphVisual.cs
- GestureRecognizer.cs
- ReflectionTypeLoadException.cs
- ThumbAutomationPeer.cs
- XmlSchemaObjectTable.cs
- PlainXmlDeserializer.cs
- StringWriter.cs
- PermissionAttributes.cs
- ChangePassword.cs
- OverrideMode.cs
- GridItem.cs
- SchemaSetCompiler.cs
- EndPoint.cs
- AppDomainAttributes.cs
- Paragraph.cs
- FileAuthorizationModule.cs
- TextBox.cs
- WaitHandle.cs
- Pkcs7Recipient.cs
- IPipelineRuntime.cs
- StickyNoteHelper.cs
- List.cs
- DataSourceXmlAttributeAttribute.cs
- OdbcPermission.cs
- SecurityRuntime.cs
- SystemDiagnosticsSection.cs
- TransformDescriptor.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- TabPage.cs
- LocalTransaction.cs
- ClientClassGenerator.cs
- BookmarkOptionsHelper.cs
- StringExpressionSet.cs
- WebPartRestoreVerb.cs
- StorageInfo.cs
- Rect.cs
- SequenceNumber.cs
- SqlDependency.cs
- SHA384.cs
- KeySplineConverter.cs
- DbConnectionPoolOptions.cs
- GACMembershipCondition.cs
- CanExecuteRoutedEventArgs.cs
- HotSpotCollection.cs
- DbConnectionFactory.cs
- PingOptions.cs
- RadioButton.cs
- TableRowCollection.cs
- DesignerLoader.cs
- TargetPerspective.cs
- Canvas.cs
- MailFileEditor.cs
- LinkTarget.cs
- SoapHeaderAttribute.cs
- GregorianCalendarHelper.cs
- ContentPlaceHolder.cs
- HashMembershipCondition.cs
- StylusPointPropertyInfoDefaults.cs
- DnsPermission.cs
- SetState.cs
- FullTextState.cs
- BitmapCodecInfo.cs
- ExpressionVisitor.cs
- UrlPath.cs
- ElementHostAutomationPeer.cs
- Overlapped.cs
- TextEditorDragDrop.cs
- MouseGesture.cs
- BufferedReadStream.cs
- TextHidden.cs
- PauseStoryboard.cs
- UIElement.cs
- ContainerParaClient.cs
- ReadOnlyDictionary.cs
- RepeaterItemCollection.cs
- FigureHelper.cs
- Listbox.cs
- PkcsMisc.cs
- counter.cs
- ListManagerBindingsCollection.cs
- WebPartsPersonalization.cs