Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / ActivityDesigner.cs / 1305376 / ActivityDesigner.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System.Activities.Presentation.Model; using System.Activities.Presentation.View; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Runtime; using System.Text; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Markup; using System.Windows.Media; using System.Windows.Controls; using System.Windows.Input; using System.Windows.Threading; public class ActivityDesigner : WorkflowViewElement { UserControl defaultDisplayNameReadOnlyControl; TextBox defaultDisplayNameBox; bool defaultDisplayNameReadOnlyControlMouseDown; [Fx.Tag.KnownXamlExternal] public DrawingBrush Icon { get { return (DrawingBrush)GetValue(IconProperty); } set { SetValue(IconProperty, value); } } public static readonly DependencyProperty IconProperty = DependencyProperty.Register("Icon", typeof(DrawingBrush), typeof(ActivityDesigner), new UIPropertyMetadata(null)); [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.InitializeReferenceTypeStaticFieldsInline, Justification="Calls to OverrideMetadata for a dependency property should be done in the static constructor.")] static ActivityDesigner() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ActivityDesigner), new FrameworkPropertyMetadata(typeof(ActivityDesigner))); } public ActivityDesigner() { this.Loaded += (sender, args) => { this.SetupDefaultIcon(); }; } protected override string GetAutomationIdMemberName() { return "DisplayName"; } protected override string GetAutomationHelpText() { StringBuilder descriptiveText = new StringBuilder(); EmitPropertyValuePair(descriptiveText, "IsPrimarySelection"); EmitPropertyValuePair(descriptiveText, "IsSelection"); EmitPropertyValuePair(descriptiveText, "IsCurrentLocation"); EmitPropertyValuePair(descriptiveText, "IsCurrentContext"); EmitPropertyValuePair(descriptiveText, "IsBreakpointEnabled"); EmitPropertyValuePair(descriptiveText, "IsBreakpointBounded"); EmitPropertyValuePair(descriptiveText, "ValidationState"); return descriptiveText.ToString(); } void EmitPropertyValuePair(StringBuilder description,string propertyName) { PropertyDescriptor property = TypeDescriptor.GetProperties(this.ModelItem)[propertyName]; object propertyValue = (property == null) ? null : property.GetValue(this.ModelItem); string propertyValueString = propertyValue == null ? "null" : propertyValue.ToString(); description.AppendFormat("{0}={1} ", propertyName, propertyValueString); } public override void OnApplyTemplate() { base.OnApplyTemplate(); if (this.defaultDisplayNameBox != null) { this.defaultDisplayNameBox.LostFocus -= new RoutedEventHandler(OnDefaultDisplayNameBoxLostFocus); this.defaultDisplayNameBox.ContextMenuOpening -= new ContextMenuEventHandler(OnDefaultDisplayNameBoxContextMenuOpening); } if(this.defaultDisplayNameReadOnlyControl != null) { this.defaultDisplayNameReadOnlyControl.MouseLeftButtonDown -= new MouseButtonEventHandler(OnDefaultDisplayNameReadOnlyControlMouseLeftButtonDown); this.defaultDisplayNameReadOnlyControl.GotKeyboardFocus -= new KeyboardFocusChangedEventHandler(OnDefaultDisplayNameReadOnlyControlGotKeyboardFocus); } this.defaultDisplayNameReadOnlyControl = this.Template.FindName("DisplayNameReadOnlyControl_6E8E4954_F6B2_4c6c_9E28_33A7A78F1E81", this) as UserControl; this.defaultDisplayNameBox = this.Template.FindName("DisplayNameBox_570C5205_7195_4d4e_953A_8E4B57EF7E7F", this) as TextBox; if (this.defaultDisplayNameBox != null && this.defaultDisplayNameReadOnlyControl != null) { this.defaultDisplayNameBox.LostFocus += new RoutedEventHandler(OnDefaultDisplayNameBoxLostFocus); this.defaultDisplayNameBox.ContextMenuOpening += new ContextMenuEventHandler(OnDefaultDisplayNameBoxContextMenuOpening); this.defaultDisplayNameReadOnlyControl.MouseLeftButtonDown += new MouseButtonEventHandler(OnDefaultDisplayNameReadOnlyControlMouseLeftButtonDown); this.defaultDisplayNameReadOnlyControl.GotKeyboardFocus += new KeyboardFocusChangedEventHandler(OnDefaultDisplayNameReadOnlyControlGotKeyboardFocus); } } void OnDefaultDisplayNameReadOnlyControlGotKeyboardFocus(object sender, KeyboardFocusChangedEventArgs e) { if (this.defaultDisplayNameBox != null && this.defaultDisplayNameReadOnlyControl != null) { DesignerView designerView = this.Context.Services.GetService(); if (!designerView.IsReadOnly && !designerView.IsMultipleSelectionMode) { this.EnterDisplayNameEditMode(); } } } void OnDefaultDisplayNameReadOnlyControlMouseLeftButtonDown(object sender, MouseButtonEventArgs e) { this.defaultDisplayNameReadOnlyControlMouseDown = true; } void OnDefaultDisplayNameBoxLostFocus(object sender, RoutedEventArgs e) { if (this.defaultDisplayNameBox != null && this.defaultDisplayNameReadOnlyControl != null) { this.ExitDisplayNameEditMode(); } } void OnDefaultDisplayNameBoxContextMenuOpening(object sender, ContextMenuEventArgs e) { // to disable the context menu e.Handled = true; } protected override void OnPreviewMouseDown(MouseButtonEventArgs e) { this.defaultDisplayNameReadOnlyControlMouseDown = false; base.OnPreviewMouseDown(e); } protected override void OnMouseUp(MouseButtonEventArgs e) { // We have to check the defaultDisplayNameReadOnlyControlMouseDown flag to determine whether the mouse is clicked on // the defaultDisplayNameReadOnlyControl. This is because the mouse capture is set on the WorkflowViewElement in // OnMouseDown, and as a result MouseUp event is not fired on the defaultDisplayNameReadOnlyControl. if (this.defaultDisplayNameBox != null && this.defaultDisplayNameReadOnlyControl != null && this.defaultDisplayNameReadOnlyControlMouseDown) { this.defaultDisplayNameReadOnlyControlMouseDown = false; DesignerView designerView = this.Context.Services.GetService (); if (!designerView.IsReadOnly) { this.EnterDisplayNameEditMode(); } } base.OnMouseUp(e); } void EnterDisplayNameEditMode() { this.defaultDisplayNameBox.Visibility = Visibility.Visible; this.defaultDisplayNameReadOnlyControl.Visibility = Visibility.Collapsed; this.Dispatcher.BeginInvoke(DispatcherPriority.ApplicationIdle, (Action)(() => { Keyboard.Focus(this.defaultDisplayNameBox); this.defaultDisplayNameBox.ScrollToHome(); })); } void ExitDisplayNameEditMode() { this.defaultDisplayNameReadOnlyControl.Visibility = Visibility.Visible; this.defaultDisplayNameBox.Visibility = Visibility.Collapsed; } private void SetupDefaultIcon() { if (this.Icon == null ) { // Look for a named icon if this property is not set if (this.ModelItem != null) { string iconKey = this.ModelItem.ItemType.IsGenericType ? this.ModelItem.ItemType.GetGenericTypeDefinition().Name : this.ModelItem.ItemType.Name; int genericParamsIndex = iconKey.IndexOf('`'); if (genericParamsIndex > 0) { iconKey = iconKey.Remove(genericParamsIndex); } iconKey = iconKey + "Icon"; try { object resourceItem = this.TryFindResource(iconKey); if (resourceItem is DrawingBrush) { this.Icon = (DrawingBrush)resourceItem; } } catch (ResourceReferenceKeyNotFoundException) { } catch (InvalidCastException) { } } if (this.Icon == null) { // as a last resort fall back to the default generic leaf activity icon. this.Icon = (DrawingBrush)this.FindResource("GenericLeafActivityIcon"); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
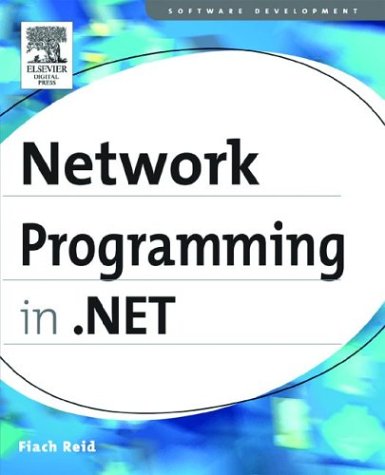
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceView.cs
- SchemaSetCompiler.cs
- FileUpload.cs
- DateTimeParse.cs
- SqlDataSourceCache.cs
- Serializer.cs
- HotSpotCollection.cs
- RemoteWebConfigurationHostServer.cs
- OracleMonthSpan.cs
- StringReader.cs
- WindowProviderWrapper.cs
- XmlSchemaExternal.cs
- Types.cs
- DiscreteKeyFrames.cs
- AggregateNode.cs
- ImageSource.cs
- SparseMemoryStream.cs
- SecurityPermission.cs
- Group.cs
- DesignerDataTableBase.cs
- HttpApplicationStateBase.cs
- CommandDevice.cs
- XmlSerializationReader.cs
- XsltArgumentList.cs
- HtmlEncodedRawTextWriter.cs
- BuildDependencySet.cs
- RubberbandSelector.cs
- Type.cs
- PolyLineSegment.cs
- CriticalHandle.cs
- ICollection.cs
- DbMetaDataFactory.cs
- DBNull.cs
- WebBrowserBase.cs
- TypeSemantics.cs
- CustomValidator.cs
- CurrentChangedEventManager.cs
- FilteredAttributeCollection.cs
- ClientCultureInfo.cs
- ProtocolViolationException.cs
- AddInEnvironment.cs
- ApplicationBuildProvider.cs
- XmlDataSourceView.cs
- InProcStateClientManager.cs
- DelegatedStream.cs
- MimeMapping.cs
- StrokeCollectionDefaultValueFactory.cs
- Int16AnimationBase.cs
- Command.cs
- ToolStripArrowRenderEventArgs.cs
- bindurihelper.cs
- DataGridViewTextBoxColumn.cs
- DocumentApplicationJournalEntry.cs
- TextRunProperties.cs
- _TransmitFileOverlappedAsyncResult.cs
- MobilePage.cs
- SpellerInterop.cs
- SchemaCollectionCompiler.cs
- BufferedGraphicsManager.cs
- ExpandCollapsePattern.cs
- WorkflowInspectionServices.cs
- BoundingRectTracker.cs
- EmbeddedMailObjectsCollection.cs
- DeferredElementTreeState.cs
- MetadataArtifactLoaderCompositeResource.cs
- PopupRoot.cs
- RawStylusInput.cs
- CqlLexerHelpers.cs
- CryptoProvider.cs
- DataMemberFieldConverter.cs
- OdbcDataReader.cs
- RelAssertionDirectKeyIdentifierClause.cs
- BamlLocalizabilityResolver.cs
- FixedPageAutomationPeer.cs
- CurrentChangingEventArgs.cs
- Vector3D.cs
- Animatable.cs
- DataBoundControlAdapter.cs
- MenuItemStyleCollection.cs
- DrawingGroup.cs
- ContractHandle.cs
- StandardBindingElement.cs
- TextServicesCompartment.cs
- SafeReadContext.cs
- WindowsIPAddress.cs
- TreeNodeCollection.cs
- HostedBindingBehavior.cs
- TypefaceCollection.cs
- InputBinder.cs
- IISMapPath.cs
- ToolBar.cs
- MulticastIPAddressInformationCollection.cs
- ProfileInfo.cs
- AssociationType.cs
- MatrixUtil.cs
- AttributeAction.cs
- SchemaImporterExtensionElement.cs
- FontDifferentiator.cs
- FieldInfo.cs
- XmlHierarchicalEnumerable.cs