Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / Activities / Core / Presentation / FlowchartDesigner.Helpers.cs / 1305376 / FlowchartDesigner.Helpers.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Activities.Core.Presentation { using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Shapes; using System.Activities.Statements; using System.Activities.Presentation.Model; using System.Linq; using System.Runtime; using System.Globalization; using System.Activities.Presentation; partial class FlowchartDesigner { //Returns true if visual is on the visual tree for point p relative to the panel. bool IsVisualHit(UIElement visual, UIElement reference, Point point) { bool visualIsHit = false; HitTestResult result = VisualTreeHelper.HitTest(reference, point); if (result != null) { DependencyObject obj = result.VisualHit; while (obj != null) { if (visual.Equals(obj)) { visualIsHit = true; break; } obj = VisualTreeHelper.GetParent(obj); } } return visualIsHit; } internal void FindClosestConnectionPointPair(ListsrcConnPoints, List destConnPoints, out ConnectionPoint srcConnPoint, out ConnectionPoint destConnPoint) { double minDist = double.PositiveInfinity; double dist; ConnectionPoint tempConnPoint; srcConnPoint = null; destConnPoint = null; foreach (ConnectionPoint connPoint in srcConnPoints) { tempConnPoint = FindClosestConnectionPoint(connPoint, destConnPoints, out dist); if (dist < minDist) { minDist = dist; srcConnPoint = connPoint; destConnPoint = tempConnPoint; } } Fx.Assert(srcConnPoint != null, "No ConnectionPoint found"); Fx.Assert(destConnPoint != null, "No ConnectionPoint found"); } internal ConnectionPoint FindClosestConnectionPoint(ConnectionPoint srcConnPoint, List destConnPoints, out double minDist) { return FindClosestConnectionPoint(srcConnPoint.Location, destConnPoints, out minDist); } internal ConnectionPoint FindClosestConnectionPoint(Point srcConnPointLocation, List destConnPoints, out double minDist) { minDist = double.PositiveInfinity; double dist = 0; ConnectionPoint closestPoint = null; foreach (ConnectionPoint destConnPoint in destConnPoints) { dist = DesignerGeometryHelper.DistanceBetweenPoints(srcConnPointLocation, destConnPoint.Location); if (dist < minDist) { minDist = dist; closestPoint = destConnPoint; } } return closestPoint; } ConnectionPoint FindClosestConnectionPointNotOfType(ConnectionPoint srcConnectionPoint, List targetConnectionPoints, ConnectionPointType illegalConnectionPointType) { double minDist; List filteredConnectionPoints = new List (); foreach (ConnectionPoint connPoint in targetConnectionPoints) { if (connPoint.PointType != illegalConnectionPointType && !connPoint.Equals(srcConnectionPoint)) { filteredConnectionPoints.Add(connPoint); } } return FindClosestConnectionPoint(srcConnectionPoint, filteredConnectionPoints, out minDist); } void RemoveAdorner(UIElement adornedElement, Type adornerType) { Fx.Assert(adornedElement != null, "Invalid argument"); Fx.Assert(typeof(Adorner).IsAssignableFrom(adornerType), "Invalid argument"); AdornerLayer adornerLayer = AdornerLayer.GetAdornerLayer(adornedElement); if (adornerLayer != null) { Adorner[] adorners = adornerLayer.GetAdorners(adornedElement); if (adorners != null) { foreach (Adorner adorner in adorners) { if (adornerType.IsAssignableFrom(adorner.GetType())) { adornerLayer.Remove(adorner); } } } } } //Returns true if child is a member of the tree rooted at the parent; bool IsParentOf(ModelItem parent, ModelItem child) { Fx.Assert(parent != null, "Invalid argument"); bool isParentOf = false; while (child != null) { if (parent.Equals(child)) { isParentOf = true; break; } child = child.Parent; } return isParentOf; } ConnectionPoint ConnectionPointHitTest(UIElement element, Point hitPoint) { ConnectionPoint hitConnectionPoint = null; List connectionPoints = new List (); List defaultConnectionPoints = FlowchartDesigner.GetConnectionPoints(element); connectionPoints.InsertRange(0, defaultConnectionPoints); connectionPoints.Add(FlowchartDesigner.GetTrueConnectionPoint(element)); connectionPoints.Add(FlowchartDesigner.GetFalseConnectionPoint(element)); foreach (ConnectionPoint connPoint in connectionPoints) { if (connPoint != null) { // connPoint.Location is the point on the Edge. // hitPoint is the mouse pointer location. // both Points are defined with respect to the coordinate system defined by the topLeft corner of the flowchart designer canvas. if (new Rect(connPoint.Location + connPoint.HitTestOffset, connPoint.HitTestSize).Contains(hitPoint)) { hitConnectionPoint = connPoint; break; } } } return hitConnectionPoint; } internal int NumberOfIncomingLinks(UIElement designer) { return GetInComingConnectors(designer).Count; } List GetAttachedConnectors(UIElement shape) { HashSet attachedConnectors = new HashSet (); List allConnectionPoints = GetAllConnectionPoints(shape); foreach (ConnectionPoint connPoint in allConnectionPoints) { if (connPoint != null) { foreach(Connector connector in connPoint.AttachedConnectors) { attachedConnectors.Add(connector); } } } return attachedConnectors.ToList (); } List GetOutGoingConnectors(UIElement shape) { List outGoingConnectors = new List (); List allConnectionPoints = GetAllConnectionPoints(shape); foreach (ConnectionPoint connPoint in allConnectionPoints) { if (connPoint != null) { outGoingConnectors.AddRange(connPoint.AttachedConnectors.Where(p => FreeFormPanel.GetSourceConnectionPoint(p).Equals(connPoint))); } } return outGoingConnectors; } List GetInComingConnectors(UIElement shape) { List inComingConnectors = new List (); List allConnectionPoints = GetAllConnectionPoints(shape); foreach (ConnectionPoint connPoint in allConnectionPoints) { if (connPoint != null) { inComingConnectors.AddRange(connPoint.AttachedConnectors.Where(p => FreeFormPanel.GetDestinationConnectionPoint(p).Equals(connPoint))); } } return inComingConnectors; } List GetAllConnectionPoints(UIElement shape) { List allConnectionPoints = new List (6); allConnectionPoints.AddRange(FlowchartDesigner.GetConnectionPoints(shape)); allConnectionPoints.Add(FlowchartDesigner.GetTrueConnectionPoint(shape)); allConnectionPoints.Add(FlowchartDesigner.GetFalseConnectionPoint(shape)); return allConnectionPoints; } Point SnapPointToGrid(Point pt) { pt.X -= pt.X % FlowchartDesigner.GridSize; pt.Y -= pt.Y % FlowchartDesigner.GridSize; pt.X = pt.X < 0 ? 0 : pt.X; pt.Y = pt.Y < 0 ? 0 : pt.Y; return pt; } //This snaps the center of the element to grid. //This is called only when dropping an item //Whereever, shapeAnchorPoint is valid, it is made co-incident with the drop location. Point SnapVisualToGrid(UIElement element, Point location, Point shapeAnchorPoint) { Fx.Assert(element != null, "Input UIElement is null"); element.Measure(new Size(double.PositiveInfinity, double.PositiveInfinity)); Point oldCenter = location; if (shapeAnchorPoint.X < 0 && shapeAnchorPoint.Y < 0) { //shapeAnchorPoint is set to (-1, -1) in case where it does not make sense (Eg. toolbox drop). //In that scenario align the center of the shape to the drop point. location.X -= element.DesiredSize.Width / 2; location.Y -= element.DesiredSize.Height / 2; } else { //The else part also takes care of the ActivityDesigner case, //where the drag handle is outside the shape. location.X -= shapeAnchorPoint.X; location.Y -= shapeAnchorPoint.Y; oldCenter = new Point(location.X + element.DesiredSize.Width / 2, location.Y + element.DesiredSize.Height / 2); } Point newCenter = SnapPointToGrid(oldCenter); location.Offset(newCenter.X - oldCenter.X, newCenter.Y - oldCenter.Y); if (location.X < 0) { double correction = FlowchartDesigner.GridSize - ((location.X * (-1)) % FlowchartDesigner.GridSize); location.X = (correction == FlowchartDesigner.GridSize) ? 0 : correction; } if (location.Y < 0) { double correction = FlowchartDesigner.GridSize - ((location.Y * (-1)) % FlowchartDesigner.GridSize); location.Y = (correction == FlowchartDesigner.GridSize) ? 0 : correction; } return location; } // This creates a link from modelItems[i] to modelItems[i+1] - foreach i between 0 and modelItems.Count-2; void CreateLinks(List modelItems) { Fx.Assert(modelItems.Count > 1, "Link creation requires more than one ModelItem"); modelItems.ForEach(p => { Fx.Assert(this.modelElement.ContainsKey(p), "View should be in the flowchart"); }); ModelItem[] modelItemsArray = modelItems.ToArray(); string errorMessage = string.Empty; for (int i = 0; i < modelItemsArray.Length - 1; i++) { string error = string.Empty; CreateLinkGesture(this.modelElement[modelItemsArray[i]], this.modelElement[modelItemsArray[i + 1]], out error, null); if (!string.Empty.Equals(error)) { errorMessage += string.Format(CultureInfo.CurrentUICulture, "Link{0}:{1}\n", i + 1, error); } } if(!string.Empty.Equals(errorMessage)) { ErrorReporting.ShowErrorMessage(errorMessage); } } // This is a utility function to pack all the elements in an array that match a particular predicate // to the end of the array, while maintaining the rest of the system unchanged. public static bool Pack (T[] toPack, Func isPacked) where T : class { if (toPack == null) { throw FxTrace.Exception.ArgumentNull("toPack"); } if (isPacked == null) { throw FxTrace.Exception.ArgumentNull("isPacked"); } int count = toPack.Length; bool needRearrange = false; bool found = false; T[] arranged = new T[count]; for (int i = 0; i < count; i++) { if (isPacked(toPack[i])) { arranged[i] = toPack[i]; toPack[i] = null; found = true; } else { if (found) { needRearrange = true; } arranged[i] = null; } } if (needRearrange) { int j = 0; for (int i = 0; i < count; i++) { if (toPack[i] != null) { toPack[j++] = toPack[i]; } } j = count; for (int i = 0; i < count; i++) { int k = count - i - 1; if (arranged[k] != null) { toPack[--j] = arranged[k]; } } } else { for (int i = 0; i < count; i++) { if (arranged[i] != null) { toPack[i] = arranged[i]; } } } return needRearrange; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
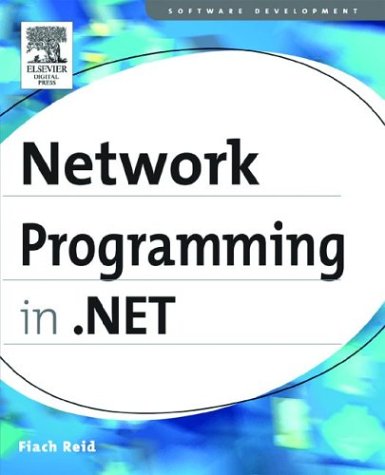
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Viewport3DAutomationPeer.cs
- StorageMappingItemCollection.cs
- CommandManager.cs
- NegotiationTokenProvider.cs
- CollectionBase.cs
- COAUTHIDENTITY.cs
- WasEndpointConfigContainer.cs
- FileChangesMonitor.cs
- TreeView.cs
- ClientOptions.cs
- TextPointer.cs
- DesignerOptions.cs
- StreamGeometry.cs
- TreeNode.cs
- SendMailErrorEventArgs.cs
- DetailsView.cs
- HyperLinkField.cs
- XmlSignatureProperties.cs
- Freezable.cs
- TTSEngineTypes.cs
- ImageList.cs
- XmlElementAttribute.cs
- DEREncoding.cs
- SafeRightsManagementPubHandle.cs
- FactoryId.cs
- ParameterReplacerVisitor.cs
- X509SecurityTokenAuthenticator.cs
- CodeTypeDelegate.cs
- AppDomainFactory.cs
- HealthMonitoringSectionHelper.cs
- OrthographicCamera.cs
- ToolConsole.cs
- AssociationType.cs
- Attributes.cs
- Int16Storage.cs
- HtmlElementErrorEventArgs.cs
- StreamReader.cs
- NullableDoubleSumAggregationOperator.cs
- ScriptDescriptor.cs
- HtmlImage.cs
- MergeFilterQuery.cs
- XPathNodePointer.cs
- CompositeCollectionView.cs
- FontStretch.cs
- ThemeDirectoryCompiler.cs
- GlyphRun.cs
- IdentityHolder.cs
- OracleParameterBinding.cs
- ScalarConstant.cs
- IsolatedStorageException.cs
- IndependentAnimationStorage.cs
- TouchPoint.cs
- COM2Properties.cs
- CatalogZoneBase.cs
- ControlLocalizer.cs
- DrawingImage.cs
- Timer.cs
- WebPartRestoreVerb.cs
- UInt16Converter.cs
- DiscoveryDocument.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- PageRouteHandler.cs
- XmlSiteMapProvider.cs
- XamlSerializerUtil.cs
- EventLogPermissionEntryCollection.cs
- MultipartIdentifier.cs
- WebPartManagerDesigner.cs
- PageThemeParser.cs
- HttpBindingExtension.cs
- AsyncOperationLifetimeManager.cs
- WebConfigurationHost.cs
- XslCompiledTransform.cs
- EntityConnectionStringBuilder.cs
- MatrixKeyFrameCollection.cs
- Int32Rect.cs
- XmlSchemaAttributeGroupRef.cs
- DependentList.cs
- PersonalizationProvider.cs
- ManagedFilter.cs
- ServiceBuildProvider.cs
- BindingList.cs
- RelationshipType.cs
- TextElementCollectionHelper.cs
- XPathConvert.cs
- TableSectionStyle.cs
- XamlTypeMapper.cs
- ProtectedConfigurationProviderCollection.cs
- ColumnMapProcessor.cs
- DbUpdateCommandTree.cs
- ActivityBindForm.Designer.cs
- TimeManager.cs
- ArgumentException.cs
- ContextMenu.cs
- LineServicesCallbacks.cs
- WCFModelStrings.Designer.cs
- CancelEventArgs.cs
- Aggregates.cs
- SoapSchemaImporter.cs
- DataSourceView.cs
- BindingMemberInfo.cs