Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Location.cs / 1305376 / Location.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Activities.Runtime; using System.ComponentModel; using System.Diagnostics; using System.Runtime.Serialization; using System.Runtime; using System.Collections.Specialized; [DataContract] [DebuggerDisplay("{Value}")] public abstract class Location { [DataMember(EmitDefaultValue = false)] TemporaryResolutionData temporaryResolutionData; protected Location() { } public abstract Type LocationType { get; } public object Value { get { return this.ValueCore; } set { this.ValueCore = value; } } internal virtual bool CanBeMapped { get { return false; } } // When we are resolving an expression that resolves to a // reference to a location we need some way of notifying the // LocationEnvironment that it should extract the inner location // and throw away the outer one. OutArgument and InOutArgument // create these TemporaryResolutionLocations if their expression // resolution goes async and LocationEnvironment gets rid of them // in CollapseTemporaryResolutionLocations(). internal LocationEnvironment TemporaryResolutionEnvironment { get { return this.temporaryResolutionData.TemporaryResolutionEnvironment; } } internal bool BufferGetsOnCollapse { get { return this.temporaryResolutionData.BufferGetsOnCollapse; } } protected abstract object ValueCore { get; set; } internal void SetTemporaryResolutionData(LocationEnvironment resolutionEnvironment, bool bufferGetsOnCollapse) { this.temporaryResolutionData = new TemporaryResolutionData { TemporaryResolutionEnvironment = resolutionEnvironment, BufferGetsOnCollapse = bufferGetsOnCollapse }; } internal virtual Location CreateReference(bool bufferGets) { if (this.CanBeMapped || bufferGets) { return new ReferenceLocation(this, bufferGets); } return this; } internal virtual object CreateDefaultValue() { Fx.Assert("We should only call this on Location"); return null; } [DataContract] struct TemporaryResolutionData { [DataMember(EmitDefaultValue = false)] public LocationEnvironment TemporaryResolutionEnvironment { get; set; } [DataMember(EmitDefaultValue = false)] public bool BufferGetsOnCollapse { get; set; } } [DataContract] class ReferenceLocation : Location { [DataMember] Location innerLocation; [DataMember(EmitDefaultValue = false)] bool bufferGets; [DataMember(EmitDefaultValue = false)] object bufferedValue; public ReferenceLocation(Location innerLocation, bool bufferGets) { this.innerLocation = innerLocation; this.bufferGets = bufferGets; } public override Type LocationType { get { return this.innerLocation.LocationType; } } protected override object ValueCore { get { if (this.bufferGets) { return this.bufferedValue; } else { return this.innerLocation.Value; } } set { this.innerLocation.Value = value; this.bufferedValue = value; } } public override string ToString() { if (bufferGets) { return base.ToString(); } else { return this.innerLocation.ToString(); } } } } [DataContract] public class Location : Location { [DataMember(EmitDefaultValue = false)] T value; public Location() : base() { } public override Type LocationType { get { return typeof(T); } } public virtual new T Value { get { return this.value; } set { this.value = value; } } internal T TypedValue { get { return this.Value; } set { this.Value = value; } } protected override sealed object ValueCore { get { return this.Value; } set { this.Value = TypeHelper.Convert (value); } } internal override Location CreateReference(bool bufferGets) { if (this.CanBeMapped || bufferGets) { return new ReferenceLocation(this, bufferGets); } return this; } internal override object CreateDefaultValue() { Fx.Assert(typeof(T).GetGenericTypeDefinition() == typeof(Location<>), "We should only be calling this with location subclasses."); return Activator.CreateInstance (); } public override string ToString() { return this.value != null ? this.value.ToString() : " "; } [DataContract] class ReferenceLocation : Location { [DataMember] Location innerLocation; [DataMember(EmitDefaultValue = false)] bool bufferGets; public ReferenceLocation(Location innerLocation, bool bufferGets) { this.innerLocation = innerLocation; this.bufferGets = bufferGets; } public override T Value { get { if (this.bufferGets) { return this.value; } else { return this.innerLocation.Value; } } set { this.innerLocation.Value = value; if (this.bufferGets) { this.value = value; } } } public override string ToString() { if (this.bufferGets) { return base.ToString(); } else { return this.innerLocation.ToString(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
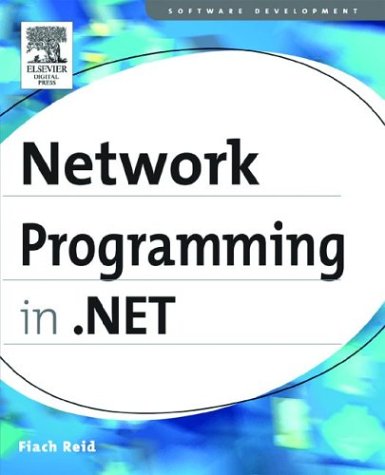
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsGraphics.cs
- RtfToken.cs
- Stroke.cs
- VerticalAlignConverter.cs
- BooleanProjectedSlot.cs
- Tokenizer.cs
- ParamArrayAttribute.cs
- Evidence.cs
- WindowsProgressbar.cs
- ButtonBaseAdapter.cs
- HostSecurityManager.cs
- LookupBindingPropertiesAttribute.cs
- TimerElapsedEvenArgs.cs
- ColorTransformHelper.cs
- AttributeCollection.cs
- TypeConstant.cs
- CompoundFileReference.cs
- DropTarget.cs
- TextBox.cs
- DataGridViewCellValueEventArgs.cs
- objectresult_tresulttype.cs
- CalendarDataBindingHandler.cs
- CrossAppDomainChannel.cs
- RegexWorker.cs
- InitializationEventAttribute.cs
- UriSection.cs
- TemplateBindingExpressionConverter.cs
- ActivityMarkupSerializationProvider.cs
- BindingManagerDataErrorEventArgs.cs
- RangeValidator.cs
- AuthenticationSection.cs
- HostProtectionPermission.cs
- ChtmlMobileTextWriter.cs
- TypeInitializationException.cs
- ISO2022Encoding.cs
- ImageListUtils.cs
- WebBrowserContainer.cs
- nulltextnavigator.cs
- IDispatchConstantAttribute.cs
- FindCriteriaCD1.cs
- WindowsClaimSet.cs
- DataGridViewSelectedRowCollection.cs
- HtmlAnchor.cs
- SqlWorkflowInstanceStore.cs
- LightweightCodeGenerator.cs
- SecurityRuntime.cs
- InstanceOwner.cs
- _SafeNetHandles.cs
- IndicShape.cs
- ImageAttributes.cs
- SubpageParagraph.cs
- DropShadowBitmapEffect.cs
- DocumentViewerConstants.cs
- httpapplicationstate.cs
- InvokeGenerator.cs
- TableCellCollection.cs
- DataGridViewToolTip.cs
- AppSecurityManager.cs
- AutomationAttributeInfo.cs
- WhitespaceRule.cs
- PageAdapter.cs
- ParsedRoute.cs
- FileCodeGroup.cs
- RadioButtonStandardAdapter.cs
- PathGeometry.cs
- ComponentResourceKeyConverter.cs
- ColorAnimationUsingKeyFrames.cs
- ListView.cs
- ContentDisposition.cs
- ConfigXmlCDataSection.cs
- DiscoveryReference.cs
- DataBindingExpressionBuilder.cs
- EntityTransaction.cs
- DataGridViewSelectedCellCollection.cs
- AutomationInteropProvider.cs
- DesignerTransactionCloseEvent.cs
- ECDiffieHellmanCng.cs
- AsymmetricKeyExchangeFormatter.cs
- XmlILTrace.cs
- TextTreeUndoUnit.cs
- ResourceCategoryAttribute.cs
- TranslateTransform3D.cs
- MLangCodePageEncoding.cs
- SmtpFailedRecipientsException.cs
- GenericRootAutomationPeer.cs
- Span.cs
- OleServicesContext.cs
- RequestCacheValidator.cs
- LambdaCompiler.Logical.cs
- AuthenticationService.cs
- BindingOperations.cs
- TabRenderer.cs
- DefaultTextStoreTextComposition.cs
- sqlstateclientmanager.cs
- MouseWheelEventArgs.cs
- SQLMoneyStorage.cs
- SortFieldComparer.cs
- DataGridViewComboBoxColumn.cs
- PeerApplication.cs
- TemplateInstanceAttribute.cs