Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / ActivityAction.cs / 1305376 / ActivityAction.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System.ComponentModel; using System.Collections.Generic; public sealed class ActivityAction : ActivityDelegate { static readonly IListEmptyDelegateParameters = new List (0); public ActivityAction() { } internal override IList InternalGetRuntimeDelegateArguments() { return ActivityAction.EmptyDelegateParameters; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (1) { {new RuntimeDelegateArgument(ActivityDelegate.ArgumentName, typeof(T), ArgumentDirection.In, this.Argument)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (2) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (3) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (4) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (5) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (6) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (7) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (8) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (9) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (10) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument11 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (11) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument11Name, typeof(T11), ArgumentDirection.In, this.Argument11)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument11 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument12 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (12) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument11Name, typeof(T11), ArgumentDirection.In, this.Argument11)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument12Name, typeof(T12), ArgumentDirection.In, this.Argument12)}, }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument11 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument12 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument13 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (13) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument11Name, typeof(T11), ArgumentDirection.In, this.Argument11)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument12Name, typeof(T12), ArgumentDirection.In, this.Argument12)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument13Name, typeof(T13), ArgumentDirection.In, this.Argument13)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument11 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument12 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument13 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument14 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (14) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument11Name, typeof(T11), ArgumentDirection.In, this.Argument11)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument12Name, typeof(T12), ArgumentDirection.In, this.Argument12)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument13Name, typeof(T13), ArgumentDirection.In, this.Argument13)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument14Name, typeof(T14), ArgumentDirection.In, this.Argument14)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument11 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument12 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument13 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument14 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument15 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (15) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument11Name, typeof(T11), ArgumentDirection.In, this.Argument11)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument12Name, typeof(T12), ArgumentDirection.In, this.Argument12)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument13Name, typeof(T13), ArgumentDirection.In, this.Argument13)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument14Name, typeof(T14), ArgumentDirection.In, this.Argument14)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument15Name, typeof(T15), ArgumentDirection.In, this.Argument15)} }; return result; } } public sealed class ActivityAction : ActivityDelegate { public ActivityAction() { } [DefaultValue(null)] public DelegateInArgument Argument1 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument2 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument3 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument4 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument5 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument6 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument7 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument8 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument9 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument10 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument11 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument12 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument13 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument14 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument15 { get; set; } [DefaultValue(null)] public DelegateInArgument Argument16 { get; set; } internal override IList InternalGetRuntimeDelegateArguments() { IList result = new List (16) { {new RuntimeDelegateArgument(ActivityDelegate.Argument1Name, typeof(T1), ArgumentDirection.In, this.Argument1)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument2Name, typeof(T2), ArgumentDirection.In, this.Argument2)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument3Name, typeof(T3), ArgumentDirection.In, this.Argument3)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument4Name, typeof(T4), ArgumentDirection.In, this.Argument4)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument5Name, typeof(T5), ArgumentDirection.In, this.Argument5)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument6Name, typeof(T6), ArgumentDirection.In, this.Argument6)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument7Name, typeof(T7), ArgumentDirection.In, this.Argument7)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument8Name, typeof(T8), ArgumentDirection.In, this.Argument8)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument9Name, typeof(T9), ArgumentDirection.In, this.Argument9)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument10Name, typeof(T10), ArgumentDirection.In, this.Argument10)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument11Name, typeof(T11), ArgumentDirection.In, this.Argument11)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument12Name, typeof(T12), ArgumentDirection.In, this.Argument12)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument13Name, typeof(T13), ArgumentDirection.In, this.Argument13)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument14Name, typeof(T14), ArgumentDirection.In, this.Argument14)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument15Name, typeof(T15), ArgumentDirection.In, this.Argument15)}, {new RuntimeDelegateArgument(ActivityDelegate.Argument16Name, typeof(T16), ArgumentDirection.In, this.Argument16)} }; return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
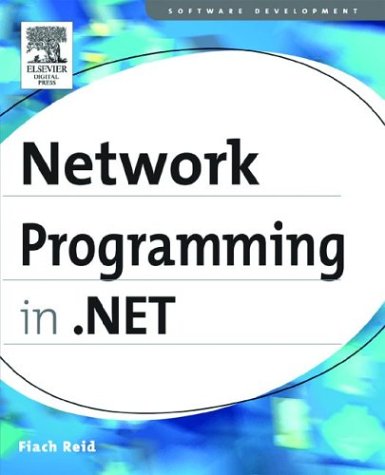
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadOnlyHierarchicalDataSource.cs
- ItemsChangedEventArgs.cs
- InvalidDataException.cs
- PersonalizationState.cs
- XsdDuration.cs
- ColumnPropertiesGroup.cs
- XmlValueConverter.cs
- CatalogPart.cs
- CustomCredentialPolicy.cs
- PointF.cs
- WebPageTraceListener.cs
- SizeIndependentAnimationStorage.cs
- TextEffect.cs
- StringUtil.cs
- WebServiceParameterData.cs
- coordinator.cs
- ModelPropertyDescriptor.cs
- MemberDescriptor.cs
- SvcMapFileSerializer.cs
- ComNativeDescriptor.cs
- DebugView.cs
- UserControlBuildProvider.cs
- PathStreamGeometryContext.cs
- ObjectStorage.cs
- CheckBoxList.cs
- NativeMethods.cs
- NamespaceEmitter.cs
- StickyNote.cs
- SHA384Cng.cs
- FtpWebRequest.cs
- DataGridViewCellValueEventArgs.cs
- Point3DCollection.cs
- TextBoxBaseDesigner.cs
- TableAdapterManagerGenerator.cs
- RunWorkerCompletedEventArgs.cs
- BitmapEffectDrawingContextState.cs
- ServicePointManager.cs
- QuaternionValueSerializer.cs
- ToolboxComponentsCreatedEventArgs.cs
- ContextQuery.cs
- StylusPoint.cs
- DescendantBaseQuery.cs
- TemplatedMailWebEventProvider.cs
- FontFaceLayoutInfo.cs
- RequestNavigateEventArgs.cs
- AbstractExpressions.cs
- ToolStrip.cs
- BitmapImage.cs
- SplitterPanel.cs
- Form.cs
- Stack.cs
- SecurityElement.cs
- ColorContext.cs
- IssuanceLicense.cs
- EntityDataSourceWizardForm.cs
- Expression.cs
- QilUnary.cs
- MetadataItemSerializer.cs
- InfoCardSymmetricAlgorithm.cs
- Site.cs
- ClientScriptManager.cs
- ListViewItem.cs
- MultiBinding.cs
- NativeMethods.cs
- SmiRecordBuffer.cs
- HostSecurityManager.cs
- DivideByZeroException.cs
- FontCollection.cs
- OpenFileDialog.cs
- SaveFileDialog.cs
- RijndaelManaged.cs
- HelpEvent.cs
- ReadContentAsBinaryHelper.cs
- KnownBoxes.cs
- Bidi.cs
- ProbeMatchesMessage11.cs
- LayoutTable.cs
- CodeParameterDeclarationExpressionCollection.cs
- TemplateColumn.cs
- Floater.cs
- MetadataWorkspace.cs
- HttpCookie.cs
- _DisconnectOverlappedAsyncResult.cs
- ConnectionPointCookie.cs
- DataListItem.cs
- InheritanceService.cs
- InfocardExtendedInformationCollection.cs
- DataFormats.cs
- TraceListeners.cs
- PageCodeDomTreeGenerator.cs
- Grant.cs
- WebServiceFault.cs
- FamilyMapCollection.cs
- EdmScalarPropertyAttribute.cs
- CellParagraph.cs
- StrongNamePublicKeyBlob.cs
- PresentationAppDomainManager.cs
- PrintPreviewControl.cs
- FormatterServices.cs
- ParallelTimeline.cs