Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TextTreePropertyUndoUnit.cs / 1305600 / TextTreePropertyUndoUnit.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Undo unit for TextContainer.SetValue, etc. calls. // // History: // 03/03/2004 : [....] - Created // //--------------------------------------------------------------------------- using System; using MS.Internal; namespace System.Windows.Documents { // Undo unit for TextContainer.SetValue, etc. calls. internal class TextTreePropertyUndoUnit : TextTreeUndoUnit { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Create a new undo unit instance. // symbolOffset is where property values will be set. internal TextTreePropertyUndoUnit(TextContainer tree, int symbolOffset, PropertyRecord propertyRecord) : base(tree, symbolOffset) { _propertyRecord = propertyRecord; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods // Called by the undo manager. Restores tree state to its condition // when the unit was created. Assumes the tree state matches conditions // just after the unit was created. public override void DoCore() { TextPointer position; VerifyTreeContentHashCode(); position = new TextPointer(this.TextContainer, this.SymbolOffset, LogicalDirection.Forward); Invariant.Assert(position.GetPointerContext(LogicalDirection.Backward) == TextPointerContext.ElementStart, "TextTree undo unit out of [....] with TextTree."); if (_propertyRecord.Value != DependencyProperty.UnsetValue) { this.TextContainer.SetValue(position, _propertyRecord.Property, _propertyRecord.Value); } else { position.Parent.ClearValue(_propertyRecord.Property); } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // Property/value pair to restore. private readonly PropertyRecord _propertyRecord; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Undo unit for TextContainer.SetValue, etc. calls. // // History: // 03/03/2004 : [....] - Created // //--------------------------------------------------------------------------- using System; using MS.Internal; namespace System.Windows.Documents { // Undo unit for TextContainer.SetValue, etc. calls. internal class TextTreePropertyUndoUnit : TextTreeUndoUnit { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Create a new undo unit instance. // symbolOffset is where property values will be set. internal TextTreePropertyUndoUnit(TextContainer tree, int symbolOffset, PropertyRecord propertyRecord) : base(tree, symbolOffset) { _propertyRecord = propertyRecord; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods // Called by the undo manager. Restores tree state to its condition // when the unit was created. Assumes the tree state matches conditions // just after the unit was created. public override void DoCore() { TextPointer position; VerifyTreeContentHashCode(); position = new TextPointer(this.TextContainer, this.SymbolOffset, LogicalDirection.Forward); Invariant.Assert(position.GetPointerContext(LogicalDirection.Backward) == TextPointerContext.ElementStart, "TextTree undo unit out of [....] with TextTree."); if (_propertyRecord.Value != DependencyProperty.UnsetValue) { this.TextContainer.SetValue(position, _propertyRecord.Property, _propertyRecord.Value); } else { position.Parent.ClearValue(_propertyRecord.Property); } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // Property/value pair to restore. private readonly PropertyRecord _propertyRecord; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
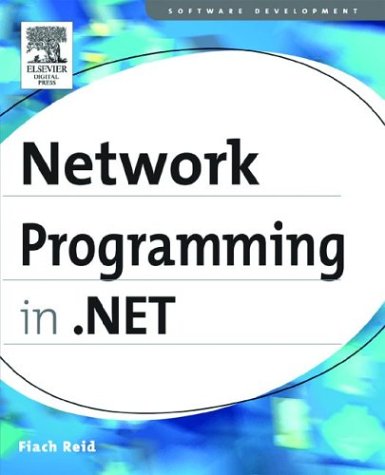
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiscoveryClientOutputChannel.cs
- ServiceHostFactory.cs
- BypassElementCollection.cs
- XhtmlBasicLinkAdapter.cs
- WrappedIUnknown.cs
- InkCanvasSelection.cs
- DataContract.cs
- D3DImage.cs
- CrossContextChannel.cs
- DetailsViewRowCollection.cs
- SecUtil.cs
- StylusPointPropertyInfoDefaults.cs
- LayoutDump.cs
- WindowsScrollBar.cs
- ValidationErrorInfo.cs
- ScriptControl.cs
- UriParserTemplates.cs
- CompModSwitches.cs
- QilLoop.cs
- NullableIntSumAggregationOperator.cs
- WindowAutomationPeer.cs
- IsolatedStorageFilePermission.cs
- StateDesigner.cs
- Vector3DAnimation.cs
- VisualCollection.cs
- StringInfo.cs
- Soap12ServerProtocol.cs
- EntityParameterCollection.cs
- NumericUpDownAccelerationCollection.cs
- FreezableCollection.cs
- DataMisalignedException.cs
- LicenseProviderAttribute.cs
- CommandEventArgs.cs
- SafeHandles.cs
- InternalConfigHost.cs
- DataGridLinkButton.cs
- DynamicValidator.cs
- TextureBrush.cs
- XmlSignatureProperties.cs
- X509SecurityToken.cs
- CompoundFileStreamReference.cs
- XmlSchemaValidator.cs
- CapacityStreamGeometryContext.cs
- MethodBody.cs
- ElementInit.cs
- _NtlmClient.cs
- ComboBoxItem.cs
- CodeStatementCollection.cs
- TypedElement.cs
- ColorContext.cs
- Library.cs
- TableLayoutSettingsTypeConverter.cs
- smtpconnection.cs
- SplineKeyFrames.cs
- TargetConverter.cs
- DataPagerFieldItem.cs
- _FtpControlStream.cs
- DefaultAsyncDataDispatcher.cs
- CharacterBuffer.cs
- Pen.cs
- ThreadStartException.cs
- Rect3DValueSerializer.cs
- LinkTarget.cs
- SeverityFilter.cs
- DataTableClearEvent.cs
- PropertyItemInternal.cs
- TextEditorMouse.cs
- SizeFConverter.cs
- WebMessageEncoderFactory.cs
- DbConnectionClosed.cs
- wgx_commands.cs
- SerializationObjectManager.cs
- DbMetaDataColumnNames.cs
- RIPEMD160Managed.cs
- HttpCapabilitiesBase.cs
- ReferentialConstraint.cs
- PieceNameHelper.cs
- HtmlCommandAdapter.cs
- LightweightCodeGenerator.cs
- SemanticTag.cs
- GridItemPattern.cs
- _ProxyChain.cs
- AtomParser.cs
- Aggregates.cs
- Timer.cs
- Pair.cs
- FloaterBaseParaClient.cs
- DnsPermission.cs
- KnownAssemblyEntry.cs
- GlobalizationSection.cs
- HeaderedItemsControl.cs
- XmlDataImplementation.cs
- BinaryMessageEncodingElement.cs
- BindToObject.cs
- Triangle.cs
- TreeNodeConverter.cs
- DrawToolTipEventArgs.cs
- CodeNamespaceImportCollection.cs
- StringOutput.cs
- ToolStripSeparator.cs