Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / LateBoundBitmapDecoder.cs / 1305600 / LateBoundBitmapDecoder.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: LateBoundBitmapDecoder.cs // //----------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // Allow suppression of certain presharp messages using System; using System.IO; using System.IO.Packaging; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Collections.ObjectModel; using System.Reflection; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows.Media.Imaging; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Net; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region LateBoundBitmapDecoder ////// LateBoundBitmapDecoder is a container for bitmap frames. Each bitmap frame is an BitmapFrame. /// Any BitmapFrame it returns are frozen /// be immutable. /// public sealed class LateBoundBitmapDecoder : BitmapDecoder { #region Constructors ////// Constructor /// ////// Critical: This code calls into BitmapDownload.BeginDownload which is critical /// [SecurityCritical] internal LateBoundBitmapDecoder( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy requestCachePolicy ) : base(true) { _baseUri = baseUri; _uri = uri; _stream = stream; _createOptions = createOptions; _cacheOption = cacheOption; _requestCachePolicy = requestCachePolicy; // Check to see if we need to download content off thread Uri uriToDecode = (_baseUri != null) ? new Uri(_baseUri, _uri) : _uri; if (uriToDecode != null) { if (uriToDecode.Scheme == Uri.UriSchemeHttp || uriToDecode.Scheme == Uri.UriSchemeHttps) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } if (_stream != null && !_stream.CanSeek) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } #endregion #region Properties ////// If there is an palette, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned Palette is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting palette data is OK /// public override BitmapPalette Palette { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Palette; } } ////// If there is an embedded color profile, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned ColorContext is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting colorcontext data is OK /// public override ReadOnlyCollectionColorContexts { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.ColorContexts; } } /// /// If there is a global thumbnail, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Thumbnail is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting thumbnail data is OK /// public override BitmapSource Thumbnail { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Thumbnail; } } ////// The info that identifies this codec. /// If the LateBoundDecoder is still downloading, the returned CodecInfo is null. /// ////// The getter demands RegistryPermission(PermissionState.Unrestricted) /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Demands RegistryPermission(PermissionState.Unrestricted) /// public override BitmapCodecInfo CodecInfo { [SecurityCritical] get { VerifyAccess(); SecurityHelper.DemandRegistryPermission(); if (_isDownloading) { return null; } return Decoder.CodecInfo; } } ////// Access to the individual frames. /// Since a LateBoundBitmapDecoder is downloaded asynchronously, /// its possible the underlying frame collection may change once /// content has been downloaded and decoded. When content is initially /// downloading, the collection will always return at least one item /// in the collection. When the download/decode is complete, the BitmapFrame /// will automatically change its underlying content. i.e. Only the collection /// object may change. The actual frame object will remain the same. /// public override ReadOnlyCollectionFrames { get { VerifyAccess(); // If the content is still being downloaded, create a collection // with 1 item that will point to an empty bitmap if (_isDownloading) { if (_readOnlyFrames == null) { _frames = new List ((int)1); _frames.Add( new BitmapFrameDecode( 0, _createOptions, _cacheOption, this ) ); _readOnlyFrames = new ReadOnlyCollection (_frames); } return _readOnlyFrames; } else { return Decoder.Frames; } } } /// /// If there is a global preview image, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Preview is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting preview data is OK /// public override BitmapSource Preview { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Preview; } } ////// Returns the underlying decoder associated with this late bound decoder. /// If the LateBoundDecoder is still downloading, the underlying decoder is null, /// otherwise the underlying decoder is created on first access. /// public BitmapDecoder Decoder { get { VerifyAccess(); if (_isDownloading || _failed) { return null; } EnsureDecoder(); return _realDecoder; } } ////// Returns if the decoder is downloading content /// public override bool IsDownloading { get { VerifyAccess(); return _isDownloading; } } #endregion #region Methods /// /// Ensure that the underlying decoder is created /// private void EnsureDecoder() { if (_realDecoder == null) { _realDecoder = BitmapDecoder.CreateFromUriOrStream( _baseUri, _uri, _stream, _createOptions & ~BitmapCreateOptions.DelayCreation, _cacheOption, _requestCachePolicy, true ); // Check to see if someone already got the frames // If so, we need to ensure that the real decoder // references the same frame as the one we already created // Creating a new object would be bad. if (_readOnlyFrames != null) { _realDecoder.SetupFrames(null, _readOnlyFrames); // // The frames have been transfered to the real decoder, so we no // longer need them. // _readOnlyFrames = null; _frames = null; } } } /// /// Called when download is complete /// internal object DownloadCallback(object arg) { Stream newStream = (Stream)arg; // Assert that we are able to seek the new stream Debug.Assert(newStream.CanSeek == true); _stream = newStream; // If we are not supposed to delay create, then ensure the decoder // otherwise it will be done on first access if ((_createOptions & BitmapCreateOptions.DelayCreation) == 0) { try { EnsureDecoder(); } catch(Exception e) { #pragma warning disable 6500 return ExceptionCallback(e); #pragma warning restore 6500 } } _isDownloading = false; _downloadEvent.InvokeEvents(this, null); return null; } /// /// Called when download progresses /// internal object ProgressCallback(object arg) { int percentComplete = (int)arg; _progressEvent.InvokeEvents(this, new DownloadProgressEventArgs(percentComplete)); return null; } /// /// Called when an exception occurs /// internal object ExceptionCallback(object arg) { _isDownloading = false; _failed = true; _failedEvent.InvokeEvents(this, new ExceptionEventArgs((Exception)arg)); return null; } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members /// Is downloading data private bool _isDownloading; /// Is downloading data private bool _failed; /// Real decoder private BitmapDecoder _realDecoder; ////// the cache policy to use for web requests. /// private RequestCachePolicy _requestCachePolicy; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: LateBoundBitmapDecoder.cs // //----------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // Allow suppression of certain presharp messages using System; using System.IO; using System.IO.Packaging; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Collections.ObjectModel; using System.Reflection; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Windows.Threading; using System.Windows.Media.Imaging; using MS.Internal.PresentationCore; // SecurityHelper using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Net; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region LateBoundBitmapDecoder ////// LateBoundBitmapDecoder is a container for bitmap frames. Each bitmap frame is an BitmapFrame. /// Any BitmapFrame it returns are frozen /// be immutable. /// public sealed class LateBoundBitmapDecoder : BitmapDecoder { #region Constructors ////// Constructor /// ////// Critical: This code calls into BitmapDownload.BeginDownload which is critical /// [SecurityCritical] internal LateBoundBitmapDecoder( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy requestCachePolicy ) : base(true) { _baseUri = baseUri; _uri = uri; _stream = stream; _createOptions = createOptions; _cacheOption = cacheOption; _requestCachePolicy = requestCachePolicy; // Check to see if we need to download content off thread Uri uriToDecode = (_baseUri != null) ? new Uri(_baseUri, _uri) : _uri; if (uriToDecode != null) { if (uriToDecode.Scheme == Uri.UriSchemeHttp || uriToDecode.Scheme == Uri.UriSchemeHttps) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } if (_stream != null && !_stream.CanSeek) { // Begin the download BitmapDownload.BeginDownload(this, uriToDecode, _requestCachePolicy, _stream); _isDownloading = true; } } #endregion #region Properties ////// If there is an palette, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned Palette is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting palette data is OK /// public override BitmapPalette Palette { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Palette; } } ////// If there is an embedded color profile, return it. /// Otherwise, return null. /// If the LateBoundDecoder is still downloading, the returned ColorContext is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting colorcontext data is OK /// public override ReadOnlyCollectionColorContexts { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.ColorContexts; } } /// /// If there is a global thumbnail, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Thumbnail is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting thumbnail data is OK /// public override BitmapSource Thumbnail { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Thumbnail; } } ////// The info that identifies this codec. /// If the LateBoundDecoder is still downloading, the returned CodecInfo is null. /// ////// The getter demands RegistryPermission(PermissionState.Unrestricted) /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Demands RegistryPermission(PermissionState.Unrestricted) /// public override BitmapCodecInfo CodecInfo { [SecurityCritical] get { VerifyAccess(); SecurityHelper.DemandRegistryPermission(); if (_isDownloading) { return null; } return Decoder.CodecInfo; } } ////// Access to the individual frames. /// Since a LateBoundBitmapDecoder is downloaded asynchronously, /// its possible the underlying frame collection may change once /// content has been downloaded and decoded. When content is initially /// downloading, the collection will always return at least one item /// in the collection. When the download/decode is complete, the BitmapFrame /// will automatically change its underlying content. i.e. Only the collection /// object may change. The actual frame object will remain the same. /// public override ReadOnlyCollectionFrames { get { VerifyAccess(); // If the content is still being downloaded, create a collection // with 1 item that will point to an empty bitmap if (_isDownloading) { if (_readOnlyFrames == null) { _frames = new List ((int)1); _frames.Add( new BitmapFrameDecode( 0, _createOptions, _cacheOption, this ) ); _readOnlyFrames = new ReadOnlyCollection (_frames); } return _readOnlyFrames; } else { return Decoder.Frames; } } } /// /// If there is a global preview image, return it. /// Otherwise, return null. The returned source is frozen. /// If the LateBoundDecoder is still downloading, the returned Preview is null. /// ////// Critical - Access unmanaged code, codecs /// PublicOK - Getting preview data is OK /// public override BitmapSource Preview { [SecurityCritical] get { VerifyAccess(); if (_isDownloading) { return null; } return Decoder.Preview; } } ////// Returns the underlying decoder associated with this late bound decoder. /// If the LateBoundDecoder is still downloading, the underlying decoder is null, /// otherwise the underlying decoder is created on first access. /// public BitmapDecoder Decoder { get { VerifyAccess(); if (_isDownloading || _failed) { return null; } EnsureDecoder(); return _realDecoder; } } ////// Returns if the decoder is downloading content /// public override bool IsDownloading { get { VerifyAccess(); return _isDownloading; } } #endregion #region Methods /// /// Ensure that the underlying decoder is created /// private void EnsureDecoder() { if (_realDecoder == null) { _realDecoder = BitmapDecoder.CreateFromUriOrStream( _baseUri, _uri, _stream, _createOptions & ~BitmapCreateOptions.DelayCreation, _cacheOption, _requestCachePolicy, true ); // Check to see if someone already got the frames // If so, we need to ensure that the real decoder // references the same frame as the one we already created // Creating a new object would be bad. if (_readOnlyFrames != null) { _realDecoder.SetupFrames(null, _readOnlyFrames); // // The frames have been transfered to the real decoder, so we no // longer need them. // _readOnlyFrames = null; _frames = null; } } } /// /// Called when download is complete /// internal object DownloadCallback(object arg) { Stream newStream = (Stream)arg; // Assert that we are able to seek the new stream Debug.Assert(newStream.CanSeek == true); _stream = newStream; // If we are not supposed to delay create, then ensure the decoder // otherwise it will be done on first access if ((_createOptions & BitmapCreateOptions.DelayCreation) == 0) { try { EnsureDecoder(); } catch(Exception e) { #pragma warning disable 6500 return ExceptionCallback(e); #pragma warning restore 6500 } } _isDownloading = false; _downloadEvent.InvokeEvents(this, null); return null; } /// /// Called when download progresses /// internal object ProgressCallback(object arg) { int percentComplete = (int)arg; _progressEvent.InvokeEvents(this, new DownloadProgressEventArgs(percentComplete)); return null; } /// /// Called when an exception occurs /// internal object ExceptionCallback(object arg) { _isDownloading = false; _failed = true; _failedEvent.InvokeEvents(this, new ExceptionEventArgs((Exception)arg)); return null; } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members /// Is downloading data private bool _isDownloading; /// Is downloading data private bool _failed; /// Real decoder private BitmapDecoder _realDecoder; ////// the cache policy to use for web requests. /// private RequestCachePolicy _requestCachePolicy; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
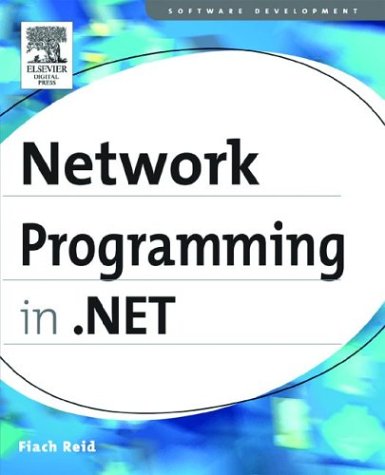
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SelfIssuedAuthAsymmetricKey.cs
- PassportAuthenticationModule.cs
- VersionedStream.cs
- InterleavedZipPartStream.cs
- ProfilePropertyNameValidator.cs
- WindowsPrincipal.cs
- XmlNamedNodeMap.cs
- GlobalEventManager.cs
- ArrayList.cs
- UnionCodeGroup.cs
- ProfilePropertyMetadata.cs
- VariableAction.cs
- PKCS1MaskGenerationMethod.cs
- MembershipSection.cs
- WinEventHandler.cs
- SafeLibraryHandle.cs
- ZipIORawDataFileBlock.cs
- EventSinkHelperWriter.cs
- X509SubjectKeyIdentifierClause.cs
- CalloutQueueItem.cs
- CircleHotSpot.cs
- JoinElimination.cs
- TimerElapsedEvenArgs.cs
- DocumentXmlWriter.cs
- Parser.cs
- Configuration.cs
- WebConfigurationFileMap.cs
- _ScatterGatherBuffers.cs
- CustomValidator.cs
- XmlSchemaImport.cs
- OpenTypeLayout.cs
- DataBinding.cs
- StreamedWorkflowDefinitionContext.cs
- FormViewInsertedEventArgs.cs
- Visual.cs
- LoginAutoFormat.cs
- precedingsibling.cs
- KeyBinding.cs
- FormViewDeleteEventArgs.cs
- ListViewSelectEventArgs.cs
- XPathQueryGenerator.cs
- RemotingSurrogateSelector.cs
- IdentityModelStringsVersion1.cs
- CodeAccessPermission.cs
- FileEnumerator.cs
- BinaryReader.cs
- HttpCachePolicyWrapper.cs
- AlgoModule.cs
- InkCanvas.cs
- FileStream.cs
- MenuItemBinding.cs
- RepeaterCommandEventArgs.cs
- BuildProvider.cs
- XmlImplementation.cs
- sqlcontext.cs
- ErrorProvider.cs
- RuntimeWrappedException.cs
- QueueProcessor.cs
- WebPartsSection.cs
- ConfigsHelper.cs
- ObjectCloneHelper.cs
- XmlDataDocument.cs
- ServiceModelReg.cs
- ConfigurationCollectionAttribute.cs
- SiteOfOriginContainer.cs
- StreamingContext.cs
- ServiceNameElement.cs
- VBCodeProvider.cs
- HttpProfileGroupBase.cs
- MemoryMappedFileSecurity.cs
- CategoryAttribute.cs
- FileUpload.cs
- XmlMapping.cs
- DisplayNameAttribute.cs
- RangeValidator.cs
- DoubleKeyFrameCollection.cs
- Decimal.cs
- WindowsListViewItem.cs
- OrderPreservingPipeliningMergeHelper.cs
- NavigationProgressEventArgs.cs
- ContentWrapperAttribute.cs
- NativeMethods.cs
- ExceptionHandlers.cs
- EventsTab.cs
- RouteParameter.cs
- LocalizabilityAttribute.cs
- As.cs
- Point.cs
- Geometry3D.cs
- RotateTransform3D.cs
- SecurityState.cs
- ModuleElement.cs
- SoapObjectWriter.cs
- EventMappingSettingsCollection.cs
- ProtectedConfigurationSection.cs
- SByteStorage.cs
- Model3DGroup.cs
- webproxy.cs
- StylusTip.cs
- SQLDoubleStorage.cs