Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / MouseActionConverter.cs / 1305600 / MouseActionConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: MouseActionConverter - Converts a MouseAction string // to the *Type* that the string represents // // // History: // 05/01/2003 : Chandrasekhar Rentachintala - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using MS.Internal; using System.Windows; using System.Windows.Input; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// MouseAction - Converter class for converting between a string and the Type of a MouseAction /// public class MouseActionConverter : TypeConverter { ////// CanConvertFrom - Used to check whether we can convert a string into a MouseAction /// ///ITypeDescriptorContext ///type to convert from ///true if the given type can be converted, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } else { return false; } } //////TypeConverter method override. /// ///ITypeDescriptorContext ///Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for known type if (context != null && context.Instance != null) { return (MouseActionConverter.IsDefinedMouseAction((MouseAction)context.Instance)); } } return false; } ////// ConvertFrom() /// /// Parser Context /// Culture Info /// MouseAction String ///public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { if (source != null && source is string) { string mouseActionToken = ((string)source).Trim(); mouseActionToken = mouseActionToken.ToUpper(CultureInfo.InvariantCulture); if (mouseActionToken == String.Empty) return MouseAction.None; MouseAction mouseAction = MouseAction.None; switch (mouseActionToken) { case "LEFTCLICK" : mouseAction = MouseAction.LeftClick; break; case "RIGHTCLICK" : mouseAction = MouseAction.RightClick; break; case "MIDDLECLICK" : mouseAction = MouseAction.MiddleClick; break; case "WHEELCLICK" : mouseAction = MouseAction.WheelClick; break; case "LEFTDOUBLECLICK" : mouseAction = MouseAction.LeftDoubleClick; break; case "RIGHTDOUBLECLICK" : mouseAction = MouseAction.RightDoubleClick; break; case "MIDDLEDOUBLECLICK": mouseAction = MouseAction.MiddleDoubleClick; break; default : throw new NotSupportedException(SR.Get(SRID.Unsupported_MouseAction, mouseActionToken)); } return mouseAction; } throw GetConvertFromException(source); } /// /// ConvertTo() /// /// Serialization Context /// Culture Info /// MouseAction value /// Type to Convert ///string if parameter is a MouseAction public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) throw new ArgumentNullException("destinationType"); if (destinationType == typeof(string) && value != null) { MouseAction mouseActionValue = (MouseAction)value ; if (MouseActionConverter.IsDefinedMouseAction(mouseActionValue)) { string mouseAction = null; switch (mouseActionValue) { case MouseAction.None : mouseAction=String.Empty; break; case MouseAction.LeftClick : mouseAction="LeftClick"; break; case MouseAction.RightClick : mouseAction="RightClick"; break; case MouseAction.MiddleClick : mouseAction="MiddleClick"; break; case MouseAction.WheelClick : mouseAction="WheelClick"; break; case MouseAction.LeftDoubleClick : mouseAction="LeftDoubleClick"; break; case MouseAction.RightDoubleClick : mouseAction="RightDoubleClick"; break; case MouseAction.MiddleDoubleClick: mouseAction="MiddleDoubleClick"; break; } if (mouseAction != null) return mouseAction; } throw new InvalidEnumArgumentException("value", (int)mouseActionValue, typeof(MouseAction)); } throw GetConvertToException(value,destinationType); } // Helper like Enum.IsDefined, for MouseAction. internal static bool IsDefinedMouseAction(MouseAction mouseAction) { return (mouseAction >= MouseAction.None && mouseAction <= MouseAction.MiddleDoubleClick); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: MouseActionConverter - Converts a MouseAction string // to the *Type* that the string represents // // // History: // 05/01/2003 : Chandrasekhar Rentachintala - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using MS.Internal; using System.Windows; using System.Windows.Input; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// MouseAction - Converter class for converting between a string and the Type of a MouseAction /// public class MouseActionConverter : TypeConverter { ////// CanConvertFrom - Used to check whether we can convert a string into a MouseAction /// ///ITypeDescriptorContext ///type to convert from ///true if the given type can be converted, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } else { return false; } } //////TypeConverter method override. /// ///ITypeDescriptorContext ///Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for known type if (context != null && context.Instance != null) { return (MouseActionConverter.IsDefinedMouseAction((MouseAction)context.Instance)); } } return false; } ////// ConvertFrom() /// /// Parser Context /// Culture Info /// MouseAction String ///public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { if (source != null && source is string) { string mouseActionToken = ((string)source).Trim(); mouseActionToken = mouseActionToken.ToUpper(CultureInfo.InvariantCulture); if (mouseActionToken == String.Empty) return MouseAction.None; MouseAction mouseAction = MouseAction.None; switch (mouseActionToken) { case "LEFTCLICK" : mouseAction = MouseAction.LeftClick; break; case "RIGHTCLICK" : mouseAction = MouseAction.RightClick; break; case "MIDDLECLICK" : mouseAction = MouseAction.MiddleClick; break; case "WHEELCLICK" : mouseAction = MouseAction.WheelClick; break; case "LEFTDOUBLECLICK" : mouseAction = MouseAction.LeftDoubleClick; break; case "RIGHTDOUBLECLICK" : mouseAction = MouseAction.RightDoubleClick; break; case "MIDDLEDOUBLECLICK": mouseAction = MouseAction.MiddleDoubleClick; break; default : throw new NotSupportedException(SR.Get(SRID.Unsupported_MouseAction, mouseActionToken)); } return mouseAction; } throw GetConvertFromException(source); } /// /// ConvertTo() /// /// Serialization Context /// Culture Info /// MouseAction value /// Type to Convert ///string if parameter is a MouseAction public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) throw new ArgumentNullException("destinationType"); if (destinationType == typeof(string) && value != null) { MouseAction mouseActionValue = (MouseAction)value ; if (MouseActionConverter.IsDefinedMouseAction(mouseActionValue)) { string mouseAction = null; switch (mouseActionValue) { case MouseAction.None : mouseAction=String.Empty; break; case MouseAction.LeftClick : mouseAction="LeftClick"; break; case MouseAction.RightClick : mouseAction="RightClick"; break; case MouseAction.MiddleClick : mouseAction="MiddleClick"; break; case MouseAction.WheelClick : mouseAction="WheelClick"; break; case MouseAction.LeftDoubleClick : mouseAction="LeftDoubleClick"; break; case MouseAction.RightDoubleClick : mouseAction="RightDoubleClick"; break; case MouseAction.MiddleDoubleClick: mouseAction="MiddleDoubleClick"; break; } if (mouseAction != null) return mouseAction; } throw new InvalidEnumArgumentException("value", (int)mouseActionValue, typeof(MouseAction)); } throw GetConvertToException(value,destinationType); } // Helper like Enum.IsDefined, for MouseAction. internal static bool IsDefinedMouseAction(MouseAction mouseAction) { return (mouseAction >= MouseAction.None && mouseAction <= MouseAction.MiddleDoubleClick); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
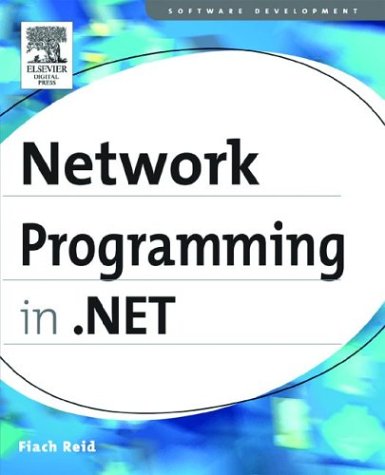
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebBrowserEvent.cs
- UnsafeNativeMethods.cs
- DbDeleteCommandTree.cs
- FullTextBreakpoint.cs
- Util.cs
- FrameworkContentElement.cs
- TrackingStringDictionary.cs
- ThreadSafeMessageFilterTable.cs
- HttpCacheVary.cs
- Adorner.cs
- DiscriminatorMap.cs
- SqlInternalConnectionTds.cs
- Membership.cs
- EntityWithChangeTrackerStrategy.cs
- TemplateBamlRecordReader.cs
- AssociationEndMember.cs
- ClientSession.cs
- Ray3DHitTestResult.cs
- Image.cs
- Int64Animation.cs
- TextDecorationLocationValidation.cs
- ISCIIEncoding.cs
- DictionaryContent.cs
- StandardCommands.cs
- DateTimeSerializationSection.cs
- CreateParams.cs
- DataRowComparer.cs
- TrackingCondition.cs
- GcSettings.cs
- CreateInstanceBinder.cs
- SqlPersonalizationProvider.cs
- TypeUtils.cs
- Stream.cs
- DeclaredTypeValidatorAttribute.cs
- EmptyReadOnlyDictionaryInternal.cs
- SafeCryptContextHandle.cs
- ScrollChrome.cs
- ComponentGuaranteesAttribute.cs
- KeyTime.cs
- TableDetailsRow.cs
- InkPresenterAutomationPeer.cs
- WorkflowTimerService.cs
- SchemaTypeEmitter.cs
- GeometryGroup.cs
- ApplicationHost.cs
- CompilationUtil.cs
- OdbcParameter.cs
- dataprotectionpermission.cs
- Coordinator.cs
- RSAPKCS1SignatureDeformatter.cs
- SpecularMaterial.cs
- TreeWalker.cs
- SerialPinChanges.cs
- PasswordTextNavigator.cs
- SchemaAttDef.cs
- HttpAsyncResult.cs
- IChannel.cs
- XamlGridLengthSerializer.cs
- Slider.cs
- InternalMappingException.cs
- TextTreeExtractElementUndoUnit.cs
- TextEndOfParagraph.cs
- ManifestSignedXml.cs
- CharacterHit.cs
- ToolTipAutomationPeer.cs
- x509utils.cs
- StyleXamlParser.cs
- RoleService.cs
- DataContractAttribute.cs
- TextEffect.cs
- SwitchAttribute.cs
- ByteRangeDownloader.cs
- CharConverter.cs
- ClientSideQueueItem.cs
- MailMessage.cs
- VirtualPathProvider.cs
- EncoderBestFitFallback.cs
- ConsumerConnectionPoint.cs
- MdiWindowListItemConverter.cs
- DataList.cs
- AuthenticateEventArgs.cs
- AttributeCollection.cs
- MexBindingElement.cs
- Button.cs
- GorillaCodec.cs
- Image.cs
- MethodBody.cs
- ClientSideQueueItem.cs
- CommandEventArgs.cs
- SymbolMethod.cs
- ClientRolePrincipal.cs
- InheritablePropertyChangeInfo.cs
- IBuiltInEvidence.cs
- Image.cs
- formatstringdialog.cs
- VerifyHashRequest.cs
- FormatStringEditor.cs
- AdRotator.cs
- X509Chain.cs
- DeploymentSectionCache.cs