Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / IO / Packaging / RightsManagementInformation.cs / 1305600 / RightsManagementInformation.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class represents the rights-management information stored in an // EncryptedPackageEnvelope. Specifically, it represents the PublishLicense and the // UseLicenses stored in the compound file that embodies the RM protected Package. // // History: // 06/06/2005: LGolding: Initial implementation. // //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Security.RightsManagement; using MS.Internal.IO.Packaging.CompoundFile; namespace System.IO.Packaging { ////// This class represents the rights-management information stored in an /// EncryptedPackageEnvelope. Specifically, it represents the PublishLicense and the /// UseLicenses stored in the compound file that embodies the RM protected Package. /// public class RightsManagementInformation { ////// Internal constructor, called by EncryptedPackageEnvelope.Create or EncryptedPackageEnvelope.Open. /// internal RightsManagementInformation( RightsManagementEncryptionTransform rmet ) { _rmet = rmet; } ////// This property represents the object that determines what operations the current /// user is allowed to perform on the encrypted content. /// public CryptoProvider CryptoProvider { get { return _rmet.CryptoProvider; } set { _rmet.CryptoProvider = value; } } ////// Read the publish license from the RM transform's primary instance data stream. /// ////// The publish license, or null if the compound file does not contain a publish /// license (as it will not, for example, when the compound file is first created). /// ////// If the stream is corrupt, or if the RM instance data in this file cannot be /// read by the current version of this class. /// public PublishLicense LoadPublishLicense() { return _rmet.LoadPublishLicense(); } ////// Save the publish license to the RM transform's instance data stream. /// /// /// The publish licence to be saved. The RM server returns a publish license as a string. /// ////// The stream is rewritten from the beginning, so any existing publish license is /// overwritten. /// ////// If ///is null. /// /// If the existing RM instance data in this file cannot be updated by the current version /// of this class. /// ////// If the transform settings are fixed. /// public void SavePublishLicense(PublishLicense publishLicense) { _rmet.SavePublishLicense(publishLicense); } ////// Load a use license for the specified user from the RM transform's instance data /// storage in the compound file. /// /// /// The user whose use license is desired. /// ////// The use license for the specified user, or null if the compound file does not /// contain a use license for the specified user. /// ////// If ///is null. /// /// If the RM information in this file cannot be read by the current version of /// this class. /// public UseLicense LoadUseLicense(ContentUser userKey) { return _rmet.LoadUseLicense(userKey); } ////// Save a use license for the specified user into the RM transform's instance data /// storage in the compound file. /// /// /// The user to whom the use license was issued. /// /// /// The use license issued to that user. /// ////// Any existing use license for the specified user is removed from the compound /// file before the new use license is saved. /// ////// If ///or is null. /// /// If the RM information in this file cannot be written by the current version of /// this class. /// public void SaveUseLicense(ContentUser userKey, UseLicense useLicense) { _rmet.SaveUseLicense(userKey, useLicense); } ////// Delete the use license for the specified user from the RM transform's instance /// data storage in the compound file. /// /// /// The user whose use license is to be deleted. /// ////// If ///is null. /// /// If the RM information in this file cannot be updated by the current version of /// this class. /// public void DeleteUseLicense(ContentUser userKey) { _rmet.DeleteUseLicense(userKey); } ////// This method retrieves a reference to a dictionary with keys of type User and values /// of type UseLicense, containing one entry for each use license embedded in the compound /// file for this particular transform instance. The collection is a snapshot of the use /// licenses in the compound file at the time of the call. The term "Embedded" in the method /// name emphasizes that the dictionary returned by this method only includes those use /// licenses that are embedded in the compound file. It does not include any other use /// licenses that the application might have acquired from an RM server but not yet embedded /// into the compound file. /// public IDictionaryGetEmbeddedUseLicenses() { return _rmet.GetEmbeddedUseLicenses(); } private RightsManagementEncryptionTransform _rmet; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class represents the rights-management information stored in an // EncryptedPackageEnvelope. Specifically, it represents the PublishLicense and the // UseLicenses stored in the compound file that embodies the RM protected Package. // // History: // 06/06/2005: LGolding: Initial implementation. // //----------------------------------------------------------------------------- using System.Collections.Generic; using System.Security.RightsManagement; using MS.Internal.IO.Packaging.CompoundFile; namespace System.IO.Packaging { ////// This class represents the rights-management information stored in an /// EncryptedPackageEnvelope. Specifically, it represents the PublishLicense and the /// UseLicenses stored in the compound file that embodies the RM protected Package. /// public class RightsManagementInformation { ////// Internal constructor, called by EncryptedPackageEnvelope.Create or EncryptedPackageEnvelope.Open. /// internal RightsManagementInformation( RightsManagementEncryptionTransform rmet ) { _rmet = rmet; } ////// This property represents the object that determines what operations the current /// user is allowed to perform on the encrypted content. /// public CryptoProvider CryptoProvider { get { return _rmet.CryptoProvider; } set { _rmet.CryptoProvider = value; } } ////// Read the publish license from the RM transform's primary instance data stream. /// ////// The publish license, or null if the compound file does not contain a publish /// license (as it will not, for example, when the compound file is first created). /// ////// If the stream is corrupt, or if the RM instance data in this file cannot be /// read by the current version of this class. /// public PublishLicense LoadPublishLicense() { return _rmet.LoadPublishLicense(); } ////// Save the publish license to the RM transform's instance data stream. /// /// /// The publish licence to be saved. The RM server returns a publish license as a string. /// ////// The stream is rewritten from the beginning, so any existing publish license is /// overwritten. /// ////// If ///is null. /// /// If the existing RM instance data in this file cannot be updated by the current version /// of this class. /// ////// If the transform settings are fixed. /// public void SavePublishLicense(PublishLicense publishLicense) { _rmet.SavePublishLicense(publishLicense); } ////// Load a use license for the specified user from the RM transform's instance data /// storage in the compound file. /// /// /// The user whose use license is desired. /// ////// The use license for the specified user, or null if the compound file does not /// contain a use license for the specified user. /// ////// If ///is null. /// /// If the RM information in this file cannot be read by the current version of /// this class. /// public UseLicense LoadUseLicense(ContentUser userKey) { return _rmet.LoadUseLicense(userKey); } ////// Save a use license for the specified user into the RM transform's instance data /// storage in the compound file. /// /// /// The user to whom the use license was issued. /// /// /// The use license issued to that user. /// ////// Any existing use license for the specified user is removed from the compound /// file before the new use license is saved. /// ////// If ///or is null. /// /// If the RM information in this file cannot be written by the current version of /// this class. /// public void SaveUseLicense(ContentUser userKey, UseLicense useLicense) { _rmet.SaveUseLicense(userKey, useLicense); } ////// Delete the use license for the specified user from the RM transform's instance /// data storage in the compound file. /// /// /// The user whose use license is to be deleted. /// ////// If ///is null. /// /// If the RM information in this file cannot be updated by the current version of /// this class. /// public void DeleteUseLicense(ContentUser userKey) { _rmet.DeleteUseLicense(userKey); } ////// This method retrieves a reference to a dictionary with keys of type User and values /// of type UseLicense, containing one entry for each use license embedded in the compound /// file for this particular transform instance. The collection is a snapshot of the use /// licenses in the compound file at the time of the call. The term "Embedded" in the method /// name emphasizes that the dictionary returned by this method only includes those use /// licenses that are embedded in the compound file. It does not include any other use /// licenses that the application might have acquired from an RM server but not yet embedded /// into the compound file. /// public IDictionaryGetEmbeddedUseLicenses() { return _rmet.GetEmbeddedUseLicenses(); } private RightsManagementEncryptionTransform _rmet; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
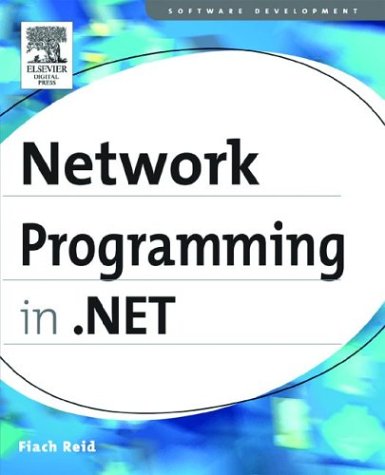
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DoubleAnimationClockResource.cs
- ConnectionStringsExpressionEditor.cs
- FilteredDataSetHelper.cs
- ConfigurationManagerHelper.cs
- InternalControlCollection.cs
- SingleStorage.cs
- _SslState.cs
- BitmapEffectGeneralTransform.cs
- SelfIssuedTokenFactoryCredential.cs
- DataSourceProvider.cs
- HtmlLabelAdapter.cs
- BaseCodePageEncoding.cs
- ZoneIdentityPermission.cs
- BitmapEffectInput.cs
- IntegrationExceptionEventArgs.cs
- Parser.cs
- BitmapSizeOptions.cs
- Int32Converter.cs
- WriteableBitmap.cs
- EdgeModeValidation.cs
- DateTimeFormatInfo.cs
- DataTrigger.cs
- Int32EqualityComparer.cs
- ErrorTableItemStyle.cs
- XmlUtilWriter.cs
- MetadataArtifactLoaderCompositeFile.cs
- ProcessStartInfo.cs
- rsa.cs
- HtmlInputReset.cs
- Pkcs7Recipient.cs
- InlineCollection.cs
- AutomationElement.cs
- KoreanLunisolarCalendar.cs
- SchemaElementDecl.cs
- PersonalizableAttribute.cs
- DbProviderSpecificTypePropertyAttribute.cs
- InputReportEventArgs.cs
- XmlElementElement.cs
- TimeZoneInfo.cs
- DataGridCell.cs
- ConsoleTraceListener.cs
- EnumMember.cs
- TraceListeners.cs
- WebPartHelpVerb.cs
- CornerRadius.cs
- SortQueryOperator.cs
- DataPointer.cs
- ZipIORawDataFileBlock.cs
- SaveFileDialogDesigner.cs
- LayoutTable.cs
- Graph.cs
- SmtpReplyReaderFactory.cs
- CodeDelegateInvokeExpression.cs
- Tag.cs
- RemoteTokenFactory.cs
- AttributeAction.cs
- FreezableCollection.cs
- WindowsAuthenticationEventArgs.cs
- UnsafePeerToPeerMethods.cs
- AssemblySettingAttributes.cs
- PrimitiveXmlSerializers.cs
- ExtendedProperty.cs
- ScriptingWebServicesSectionGroup.cs
- SHA1.cs
- ListViewItem.cs
- DbParameterHelper.cs
- GuidConverter.cs
- InsufficientMemoryException.cs
- WebHttpSecurityModeHelper.cs
- PerspectiveCamera.cs
- CultureTableRecord.cs
- SqlServer2KCompatibilityCheck.cs
- CustomPopupPlacement.cs
- DrawingState.cs
- HttpStreams.cs
- safelinkcollection.cs
- ValidatingPropertiesEventArgs.cs
- ChangeDirector.cs
- XhtmlBasicPanelAdapter.cs
- PagedDataSource.cs
- Stroke.cs
- ConnectionInterfaceCollection.cs
- TableRowCollection.cs
- Convert.cs
- MultipleCopiesCollection.cs
- DataSet.cs
- ManagementNamedValueCollection.cs
- SerializationEventsCache.cs
- Soap.cs
- MethodBody.cs
- SecUtil.cs
- HtmlTernaryTree.cs
- ReturnEventArgs.cs
- TabRenderer.cs
- PlatformCulture.cs
- XmlSchemaAttributeGroup.cs
- NamespaceInfo.cs
- XmlCompatibilityReader.cs
- SecureUICommand.cs
- Crc32.cs