Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / Verify.cs / 1305600 / Verify.cs
/**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace MS.Internal { using System; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Threading; using System.IO; using MS.Internal.WindowsBase; ////// A static class for retail validated assertions. /// Instead of breaking into the debugger an exception is thrown. /// internal static class Verify { ////// Ensure that the current thread's apartment state is what's expected. /// /// /// The required apartment state for the current thread. /// /// /// The message string for the exception to be thrown if the state is invalid. /// ////// Thrown if the calling thread's apartment state is not the same as the requiredState. /// public static void IsApartmentState(ApartmentState requiredState) { if (Thread.CurrentThread.GetApartmentState() != requiredState) { throw new InvalidOperationException(SR.Get(SRID.Verify_ApartmentState, requiredState)); } } ////// Ensure that an argument is neither null nor empty. /// /// The string to validate. /// The name of the parameter that will be presented if an exception is thrown. public static void IsNeitherNullNorEmpty(string value, string name) { // catch caller errors, mixing up the parameters. Name should never be empty. Debug.Assert(!string.IsNullOrEmpty(name)); // Notice that ArgumentNullException and ArgumentException take the parameters in opposite order :P if (value == null) { throw new ArgumentNullException(name, SR.Get(SRID.Verify_NeitherNullNorEmpty)); } if (value == "") { throw new ArgumentException(SR.Get(SRID.Verify_NeitherNullNorEmpty), name); } } ///Verifies that an argument is not null. ///Type of the object to validate. Must be a class. /// The object to validate. /// The name of the parameter that will be presented if an exception is thrown. public static void IsNotNull(T obj, string name) where T : class { if (obj == null) { throw new ArgumentNullException(name); } } /// /// Verifies the specified expression is true. Throws an ArgumentException if it's not. /// /// The expression to be verified as true. /// Name of the parameter to include in the ArgumentException. /// The message to include in the ArgumentException. public static void IsTrue(bool expression, string name, string message) { if (!expression) { throw new ArgumentException(message, name); } } ////// Verifies two values are not equal to each other. Throws an ArgumentException if they are. /// /// The actual value. /// The value that 'actual' should not be. /// The name to display for 'actual' in the exception if this test fails. /// The message to include in the ArgumentException. public static void AreNotEqual(T actual, T notExpected, string parameterName, string message) { if (notExpected == null) { // Two nulls are considered equal, regardless of type semantics. if (actual == null || actual.Equals(notExpected)) { throw new ArgumentException(SR.Get(SRID.Verify_AreNotEqual, notExpected), parameterName); } } else if (notExpected.Equals(actual)) { throw new ArgumentException(SR.Get(SRID.Verify_AreNotEqual, notExpected), parameterName); } } /// /// Verifies the specified file exists. Throws an ArgumentException if it doesn't. /// /// The file path to check for existence. /// Name of the parameter to include in the ArgumentException. ///This method demands FileIOPermission(FileIOPermissionAccess.PathDiscovery) public static void FileExists(string filePath, string parameterName) { Verify.IsNeitherNullNorEmpty(filePath, parameterName); if (!File.Exists(filePath)) { throw new ArgumentException(SR.Get(SRID.Verify_FileExists, filePath), parameterName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace MS.Internal { using System; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Threading; using System.IO; using MS.Internal.WindowsBase; ////// A static class for retail validated assertions. /// Instead of breaking into the debugger an exception is thrown. /// internal static class Verify { ////// Ensure that the current thread's apartment state is what's expected. /// /// /// The required apartment state for the current thread. /// /// /// The message string for the exception to be thrown if the state is invalid. /// ////// Thrown if the calling thread's apartment state is not the same as the requiredState. /// public static void IsApartmentState(ApartmentState requiredState) { if (Thread.CurrentThread.GetApartmentState() != requiredState) { throw new InvalidOperationException(SR.Get(SRID.Verify_ApartmentState, requiredState)); } } ////// Ensure that an argument is neither null nor empty. /// /// The string to validate. /// The name of the parameter that will be presented if an exception is thrown. public static void IsNeitherNullNorEmpty(string value, string name) { // catch caller errors, mixing up the parameters. Name should never be empty. Debug.Assert(!string.IsNullOrEmpty(name)); // Notice that ArgumentNullException and ArgumentException take the parameters in opposite order :P if (value == null) { throw new ArgumentNullException(name, SR.Get(SRID.Verify_NeitherNullNorEmpty)); } if (value == "") { throw new ArgumentException(SR.Get(SRID.Verify_NeitherNullNorEmpty), name); } } ///Verifies that an argument is not null. ///Type of the object to validate. Must be a class. /// The object to validate. /// The name of the parameter that will be presented if an exception is thrown. public static void IsNotNull(T obj, string name) where T : class { if (obj == null) { throw new ArgumentNullException(name); } } /// /// Verifies the specified expression is true. Throws an ArgumentException if it's not. /// /// The expression to be verified as true. /// Name of the parameter to include in the ArgumentException. /// The message to include in the ArgumentException. public static void IsTrue(bool expression, string name, string message) { if (!expression) { throw new ArgumentException(message, name); } } ////// Verifies two values are not equal to each other. Throws an ArgumentException if they are. /// /// The actual value. /// The value that 'actual' should not be. /// The name to display for 'actual' in the exception if this test fails. /// The message to include in the ArgumentException. public static void AreNotEqual(T actual, T notExpected, string parameterName, string message) { if (notExpected == null) { // Two nulls are considered equal, regardless of type semantics. if (actual == null || actual.Equals(notExpected)) { throw new ArgumentException(SR.Get(SRID.Verify_AreNotEqual, notExpected), parameterName); } } else if (notExpected.Equals(actual)) { throw new ArgumentException(SR.Get(SRID.Verify_AreNotEqual, notExpected), parameterName); } } /// /// Verifies the specified file exists. Throws an ArgumentException if it doesn't. /// /// The file path to check for existence. /// Name of the parameter to include in the ArgumentException. ///This method demands FileIOPermission(FileIOPermissionAccess.PathDiscovery) public static void FileExists(string filePath, string parameterName) { Verify.IsNeitherNullNorEmpty(filePath, parameterName); if (!File.Exists(filePath)) { throw new ArgumentException(SR.Get(SRID.Verify_FileExists, filePath), parameterName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
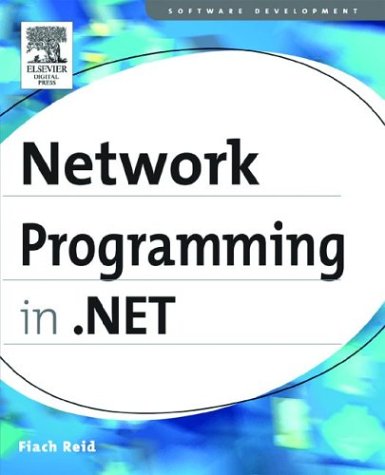
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MultiDataTrigger.cs
- HtmlInputReset.cs
- IconConverter.cs
- ManagementEventWatcher.cs
- HttpCacheVary.cs
- RectangleGeometry.cs
- ObjectDataSourceChooseMethodsPanel.cs
- SmiGettersStream.cs
- ChannelOptions.cs
- ColumnBinding.cs
- CapabilitiesAssignment.cs
- InstanceData.cs
- ProfilePropertySettings.cs
- Brush.cs
- ApplicationDirectoryMembershipCondition.cs
- _Win32.cs
- DataGridViewDataErrorEventArgs.cs
- FlowLayout.cs
- TextBoxView.cs
- MimeTypeMapper.cs
- ListItemsCollectionEditor.cs
- ValidationHelpers.cs
- AssemblyInfo.cs
- Delegate.cs
- SrgsGrammarCompiler.cs
- ConfigurationValidatorBase.cs
- TextElementEnumerator.cs
- ReadOnlyCollectionBase.cs
- IisTraceWebEventProvider.cs
- WebPartsPersonalization.cs
- ListBoxAutomationPeer.cs
- Intellisense.cs
- _CommandStream.cs
- MenuCommandService.cs
- RepeatBehaviorConverter.cs
- SHA1CryptoServiceProvider.cs
- PropertyGridEditorPart.cs
- DataGridCellClipboardEventArgs.cs
- AsymmetricSignatureDeformatter.cs
- PropertyValue.cs
- TraceSwitch.cs
- Attributes.cs
- SafeNativeMethodsCLR.cs
- UInt16Converter.cs
- SessionStateModule.cs
- JavaScriptSerializer.cs
- ManagementDateTime.cs
- TextTreeUndo.cs
- CodeBinaryOperatorExpression.cs
- ColorConverter.cs
- AuthenticationException.cs
- TextTreeTextNode.cs
- SiteMapNodeCollection.cs
- Hyperlink.cs
- RadioButtonAutomationPeer.cs
- DummyDataSource.cs
- InfoCardMetadataExchangeClient.cs
- StringFreezingAttribute.cs
- EventTrigger.cs
- AuthenticateEventArgs.cs
- TextAnchor.cs
- XmlSchemaAppInfo.cs
- _TimerThread.cs
- GroupBoxAutomationPeer.cs
- RelOps.cs
- IdentityNotMappedException.cs
- UriTemplateMatch.cs
- BaseComponentEditor.cs
- ProxyWebPartManager.cs
- ItemsPresenter.cs
- SettingsPropertyIsReadOnlyException.cs
- BaseTypeViewSchema.cs
- EncoderFallback.cs
- ValidatingReaderNodeData.cs
- IDataContractSurrogate.cs
- OdbcCommandBuilder.cs
- SecurityVerifiedMessage.cs
- DBCommandBuilder.cs
- TemplateFactory.cs
- DataTemplateSelector.cs
- SafeHandles.cs
- HostUtils.cs
- RetrieveVirtualItemEventArgs.cs
- Thumb.cs
- WorkflowItemsPresenter.cs
- XmlReflectionImporter.cs
- DockingAttribute.cs
- TypeExtensionSerializer.cs
- HtmlInputSubmit.cs
- EventTask.cs
- DaylightTime.cs
- DateTimeFormat.cs
- ConfigsHelper.cs
- TextRangeSerialization.cs
- ResourcePart.cs
- SplitContainer.cs
- FloaterParagraph.cs
- LifetimeManager.cs
- HtmlElementEventArgs.cs
- EncoderNLS.cs