Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / Script / Services / PageClientProxyGenerator.cs / 1305376 / PageClientProxyGenerator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Script.Services { using System.Web; using System.Web.UI; internal class PageClientProxyGenerator : ClientProxyGenerator { private string _path; internal PageClientProxyGenerator(IPage page, bool debug) : this(VirtualPathUtility.MakeRelative(page.Request.Path, page.Request.FilePath), debug) { // Dev10 Bug 597146: Use VirtualPathUtility to build a relative path from the path to the file. // Previously just Page.Request.FilePath was used, which was for example, /app/foo/page.aspx, // but this breaks with cookieless sessions since the url is /app/foo/(sessionid)/page.aspx. // We need to make a relative path from page.Request.Path (e.g. /app/foo) to page.Request.FilePath // (e.g. /app/foo/page.aspx) rather than just strip off 'page.aspx' with Path.GetFileName, because // the url may include PathInfo, such as "/app/foo/page.aspx/pathinfo1/pathinfo2", and in that case // we need the path to be ../../page.aspx } internal PageClientProxyGenerator(string path, bool debug) { _path = path; _debugMode = debug; } internal static string GetClientProxyScript(HttpContext context, IPage page, bool debug) { // Do nothing during unit tests which have no context or page if (context == null || page == null) return null; WebServiceData webServiceData = WebServiceData.GetWebServiceData(context, page.AppRelativeVirtualPath, false /*failIfNoData*/, true /*pageMethods */); if (webServiceData == null) return null; PageClientProxyGenerator proxyGenerator = new PageClientProxyGenerator(page, debug); return proxyGenerator.GetClientProxyScript(webServiceData); } protected override void GenerateTypeDeclaration(WebServiceData webServiceData, bool genClass) { if (genClass) { _builder.Append("PageMethods.prototype = "); } else { _builder.Append("var PageMethods = "); } } protected override string GetProxyTypeName(WebServiceData data) { return "PageMethods"; } protected override string GetProxyPath() { return _path; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Script.Services { using System.Web; using System.Web.UI; internal class PageClientProxyGenerator : ClientProxyGenerator { private string _path; internal PageClientProxyGenerator(IPage page, bool debug) : this(VirtualPathUtility.MakeRelative(page.Request.Path, page.Request.FilePath), debug) { // Dev10 Bug 597146: Use VirtualPathUtility to build a relative path from the path to the file. // Previously just Page.Request.FilePath was used, which was for example, /app/foo/page.aspx, // but this breaks with cookieless sessions since the url is /app/foo/(sessionid)/page.aspx. // We need to make a relative path from page.Request.Path (e.g. /app/foo) to page.Request.FilePath // (e.g. /app/foo/page.aspx) rather than just strip off 'page.aspx' with Path.GetFileName, because // the url may include PathInfo, such as "/app/foo/page.aspx/pathinfo1/pathinfo2", and in that case // we need the path to be ../../page.aspx } internal PageClientProxyGenerator(string path, bool debug) { _path = path; _debugMode = debug; } internal static string GetClientProxyScript(HttpContext context, IPage page, bool debug) { // Do nothing during unit tests which have no context or page if (context == null || page == null) return null; WebServiceData webServiceData = WebServiceData.GetWebServiceData(context, page.AppRelativeVirtualPath, false /*failIfNoData*/, true /*pageMethods */); if (webServiceData == null) return null; PageClientProxyGenerator proxyGenerator = new PageClientProxyGenerator(page, debug); return proxyGenerator.GetClientProxyScript(webServiceData); } protected override void GenerateTypeDeclaration(WebServiceData webServiceData, bool genClass) { if (genClass) { _builder.Append("PageMethods.prototype = "); } else { _builder.Append("var PageMethods = "); } } protected override string GetProxyTypeName(WebServiceData data) { return "PageMethods"; } protected override string GetProxyPath() { return _path; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
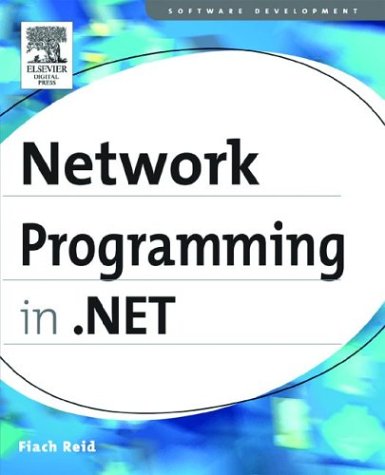
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FamilyMap.cs
- MessageBodyDescription.cs
- CredentialCache.cs
- CompiledQueryCacheKey.cs
- NameObjectCollectionBase.cs
- ExecutionTracker.cs
- MenuAdapter.cs
- TextSelectionProcessor.cs
- InvalidCardException.cs
- Geometry.cs
- AutoResizedEvent.cs
- TableLayoutStyleCollection.cs
- ListView.cs
- DocumentPageView.cs
- ButtonBaseAutomationPeer.cs
- hresults.cs
- SoapSchemaMember.cs
- glyphs.cs
- ipaddressinformationcollection.cs
- DataGridColumnDropSeparator.cs
- SystemIPInterfaceProperties.cs
- BuildProvider.cs
- TypeInitializationException.cs
- WorkerRequest.cs
- TreeNodeMouseHoverEvent.cs
- SystemFonts.cs
- WsatServiceCertificate.cs
- ButtonColumn.cs
- ListControlBuilder.cs
- ConfigXmlText.cs
- DisplayInformation.cs
- RuleAction.cs
- CursorConverter.cs
- PermissionSet.cs
- InterleavedZipPartStream.cs
- ThreadAbortException.cs
- DynamicPropertyHolder.cs
- Vector3DValueSerializer.cs
- GenericAuthenticationEventArgs.cs
- MainMenu.cs
- DbModificationClause.cs
- FixedPageAutomationPeer.cs
- GifBitmapEncoder.cs
- DbParameterCollectionHelper.cs
- ServiceXNameTypeConverter.cs
- XmlSchemaFacet.cs
- DataGridViewHeaderCell.cs
- ISessionStateStore.cs
- TreeIterators.cs
- TableLayoutRowStyleCollection.cs
- SmtpLoginAuthenticationModule.cs
- XmlDocumentFragment.cs
- QilScopedVisitor.cs
- BulletDecorator.cs
- UnhandledExceptionEventArgs.cs
- MouseActionValueSerializer.cs
- QueryExtender.cs
- FtpWebRequest.cs
- CustomExpression.cs
- DataGridAddNewRow.cs
- XmlILAnnotation.cs
- TransformGroup.cs
- DataGridViewToolTip.cs
- TreeWalker.cs
- CommandValueSerializer.cs
- ExcludeFromCodeCoverageAttribute.cs
- DisplayToken.cs
- ObjectDataSourceSelectingEventArgs.cs
- ProgressChangedEventArgs.cs
- Encoding.cs
- Padding.cs
- GroupQuery.cs
- WmlValidatorAdapter.cs
- TextCompositionEventArgs.cs
- IntegrationExceptionEventArgs.cs
- IpcClientChannel.cs
- UriSectionData.cs
- COAUTHINFO.cs
- DesignerToolStripControlHost.cs
- ChtmlTextWriter.cs
- LoginUtil.cs
- ListParagraph.cs
- Type.cs
- ValidationRule.cs
- QueryInterceptorAttribute.cs
- PresentationSource.cs
- SoapElementAttribute.cs
- ComEventsInfo.cs
- Image.cs
- WebPartsSection.cs
- ISAPIRuntime.cs
- DictionaryContent.cs
- Evidence.cs
- LinqDataView.cs
- PreservationFileReader.cs
- EventLog.cs
- _FtpControlStream.cs
- XmlAttributeAttribute.cs
- UrlPropertyAttribute.cs
- Quaternion.cs