Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / security / system / security / authentication / ExtendedProtection / ServiceNameCollection.cs / 1305376 / ServiceNameCollection.cs
using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; namespace System.Security.Authentication.ExtendedProtection { // derived from ReadOnlyCollectionBase because it needs to be back ported to .Net 1.x [SuppressMessage("Microsoft.Design","CA1058:TypesShouldNotExtendCertainBaseTypes", Justification="changing this would be a breaking change; this code has already shipped")] [Serializable] public class ServiceNameCollection : ReadOnlyCollectionBase { public ServiceNameCollection(ICollection items) { if (items == null) { throw new ArgumentNullException("items"); } InnerList.AddRange(items); } public ServiceNameCollection Merge(string serviceName) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); AddIfNew(newServiceNames, serviceName); ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } public ServiceNameCollection Merge(IEnumerable serviceNames) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); // we have a pretty bad performance here: O(n^2), but since service name lists should // be small (<<50) and Merge() should not be called frequently, this shouldn't be an issue foreach (object item in serviceNames) { AddIfNew(newServiceNames, item as string); } ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } private void AddIfNew(ArrayList newServiceNames, string serviceName) { if (String.IsNullOrEmpty(serviceName)) { throw new ArgumentException(SR.GetString(SR.security_ServiceNameCollection_EmptyServiceName)); } if (!Contains(serviceName, newServiceNames)) { newServiceNames.Add(serviceName); } } private bool Contains(string searchServiceName, ICollection serviceNames) { Debug.Assert(serviceNames != null); Debug.Assert(!String.IsNullOrEmpty(searchServiceName)); bool found = false; foreach (string serviceName in serviceNames) { if (String.Compare(serviceName, searchServiceName, StringComparison.OrdinalIgnoreCase) == 0) { found = true; break; } } return found; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; namespace System.Security.Authentication.ExtendedProtection { // derived from ReadOnlyCollectionBase because it needs to be back ported to .Net 1.x [SuppressMessage("Microsoft.Design","CA1058:TypesShouldNotExtendCertainBaseTypes", Justification="changing this would be a breaking change; this code has already shipped")] [Serializable] public class ServiceNameCollection : ReadOnlyCollectionBase { public ServiceNameCollection(ICollection items) { if (items == null) { throw new ArgumentNullException("items"); } InnerList.AddRange(items); } public ServiceNameCollection Merge(string serviceName) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); AddIfNew(newServiceNames, serviceName); ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } public ServiceNameCollection Merge(IEnumerable serviceNames) { ArrayList newServiceNames = new ArrayList(); // be compatible with .Net 1.x; no generics newServiceNames.AddRange(this.InnerList); // we have a pretty bad performance here: O(n^2), but since service name lists should // be small (<<50) and Merge() should not be called frequently, this shouldn't be an issue foreach (object item in serviceNames) { AddIfNew(newServiceNames, item as string); } ServiceNameCollection newCollection = new ServiceNameCollection(newServiceNames); return newCollection; } private void AddIfNew(ArrayList newServiceNames, string serviceName) { if (String.IsNullOrEmpty(serviceName)) { throw new ArgumentException(SR.GetString(SR.security_ServiceNameCollection_EmptyServiceName)); } if (!Contains(serviceName, newServiceNames)) { newServiceNames.Add(serviceName); } } private bool Contains(string searchServiceName, ICollection serviceNames) { Debug.Assert(serviceNames != null); Debug.Assert(!String.IsNullOrEmpty(searchServiceName)); bool found = false; foreach (string serviceName in serviceNames) { if (String.Compare(serviceName, searchServiceName, StringComparison.OrdinalIgnoreCase) == 0) { found = true; break; } } return found; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
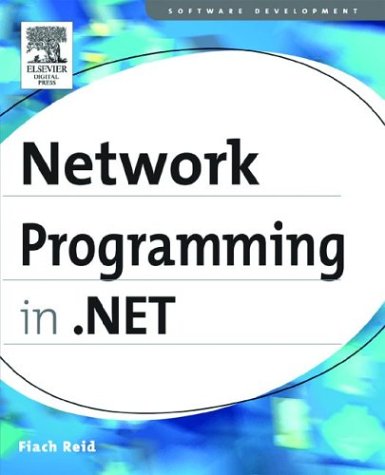
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpApplicationFactory.cs
- CompilationAssemblyInstallComponent.cs
- XmlQueryOutput.cs
- Column.cs
- RepeaterItemEventArgs.cs
- WizardSideBarListControlItem.cs
- ProxyElement.cs
- MutexSecurity.cs
- AcceleratedTokenProviderState.cs
- _OSSOCK.cs
- ParsedAttributeCollection.cs
- NodeInfo.cs
- BuilderPropertyEntry.cs
- CodeTypeReference.cs
- UpdatePanelTriggerCollection.cs
- FloatUtil.cs
- SqlClientWrapperSmiStream.cs
- CompModSwitches.cs
- RowBinding.cs
- DataGrid.cs
- System.Data.OracleClient_BID.cs
- ProfileService.cs
- UInt16Converter.cs
- NativeMethods.cs
- X509Chain.cs
- ItemList.cs
- RSATokenProvider.cs
- streamingZipPartStream.cs
- SingleAnimationBase.cs
- SafeLocalMemHandle.cs
- DataServiceContext.cs
- WebReferencesBuildProvider.cs
- Point.cs
- InkCanvasAutomationPeer.cs
- HttpServerVarsCollection.cs
- RegularExpressionValidator.cs
- TextAction.cs
- Walker.cs
- ContainerVisual.cs
- KoreanCalendar.cs
- GeneralTransform3DGroup.cs
- GroupItem.cs
- Model3DGroup.cs
- LocalBuilder.cs
- WorkflowRuntimeServiceElement.cs
- path.cs
- MemberAssignment.cs
- CodeExpressionCollection.cs
- Image.cs
- AssemblyCache.cs
- StatusStrip.cs
- Mutex.cs
- InertiaTranslationBehavior.cs
- CodeAttributeDeclaration.cs
- DataServices.cs
- AdapterDictionary.cs
- ReplacementText.cs
- ListViewItem.cs
- WebPartDescriptionCollection.cs
- ExceptionRoutedEventArgs.cs
- SQLRoleProvider.cs
- TabItem.cs
- OleDbMetaDataFactory.cs
- ProviderMetadataCachedInformation.cs
- DetailsViewDeleteEventArgs.cs
- SynchronizedInputAdaptor.cs
- AssociationType.cs
- Pair.cs
- DBBindings.cs
- CapabilitiesRule.cs
- ChannelSinkStacks.cs
- DashStyle.cs
- ShapingWorkspace.cs
- UrlMapping.cs
- XmlRawWriterWrapper.cs
- SamlSecurityToken.cs
- Win32Native.cs
- Classification.cs
- SystemColors.cs
- TypeForwardedFromAttribute.cs
- SettingsSection.cs
- Header.cs
- UTF8Encoding.cs
- MethodExecutor.cs
- StructuredTypeEmitter.cs
- DataTableTypeConverter.cs
- VectorCollectionValueSerializer.cs
- TransformerInfo.cs
- OrCondition.cs
- DateTimePickerDesigner.cs
- OleDbConnectionInternal.cs
- Decimal.cs
- SafeCertificateContext.cs
- PreloadedPackages.cs
- DataServiceKeyAttribute.cs
- HttpDictionary.cs
- SymbolPair.cs
- RectangleConverter.cs
- XmlMapping.cs
- AddInContractAttribute.cs