Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Dom / DomNameTable.cs / 1305376 / DomNameTable.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Schema; namespace System.Xml { internal class DomNameTable { XmlName[] entries; int count; int mask; XmlDocument ownerDocument; XmlNameTable nameTable; const int InitialSize = 64; // must be a power of two public DomNameTable( XmlDocument document ) { ownerDocument = document; nameTable = document.NameTable; entries = new XmlName[InitialSize]; mask = InitialSize - 1; Debug.Assert( ( entries.Length & mask ) == 0 ); // entries.Length must be a power of two } public XmlName GetName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } return null; } public XmlName AddName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } prefix = nameTable.Add(prefix); localName = nameTable.Add(localName); ns = nameTable.Add(ns); int index = hashCode & mask; XmlName name = XmlName.Create(prefix, localName, ns, hashCode, ownerDocument, entries[index], schemaInfo); entries[index] = name; if (count++ == mask) { Grow(); } return name; } private void Grow() { int newMask = mask * 2 + 1; XmlName[] oldEntries = entries; XmlName[] newEntries = new XmlName[newMask+1]; // use oldEntries.Length to eliminate the rangecheck for ( int i = 0; i < oldEntries.Length; i++ ) { XmlName name = oldEntries[i]; while ( name != null ) { int newIndex = name.HashCode & newMask; XmlName tmp = name.next; name.next = newEntries[newIndex]; newEntries[newIndex] = name; name = tmp; } } entries = newEntries; mask = newMask; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Schema; namespace System.Xml { internal class DomNameTable { XmlName[] entries; int count; int mask; XmlDocument ownerDocument; XmlNameTable nameTable; const int InitialSize = 64; // must be a power of two public DomNameTable( XmlDocument document ) { ownerDocument = document; nameTable = document.NameTable; entries = new XmlName[InitialSize]; mask = InitialSize - 1; Debug.Assert( ( entries.Length & mask ) == 0 ); // entries.Length must be a power of two } public XmlName GetName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } return null; } public XmlName AddName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } prefix = nameTable.Add(prefix); localName = nameTable.Add(localName); ns = nameTable.Add(ns); int index = hashCode & mask; XmlName name = XmlName.Create(prefix, localName, ns, hashCode, ownerDocument, entries[index], schemaInfo); entries[index] = name; if (count++ == mask) { Grow(); } return name; } private void Grow() { int newMask = mask * 2 + 1; XmlName[] oldEntries = entries; XmlName[] newEntries = new XmlName[newMask+1]; // use oldEntries.Length to eliminate the rangecheck for ( int i = 0; i < oldEntries.Length; i++ ) { XmlName name = oldEntries[i]; while ( name != null ) { int newIndex = name.HashCode & newMask; XmlName tmp = name.next; name.next = newEntries[newIndex]; newEntries[newIndex] = name; name = tmp; } } entries = newEntries; mask = newMask; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
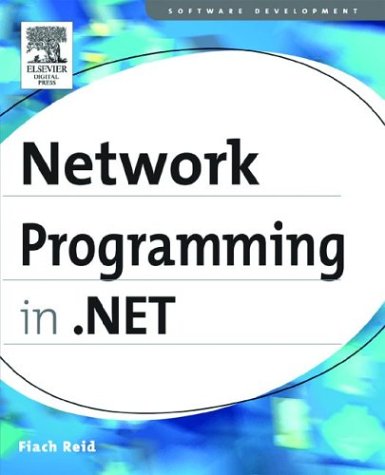
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeObject.cs
- XmlSignificantWhitespace.cs
- Action.cs
- DivideByZeroException.cs
- NativeMethodsOther.cs
- DiscoveryReference.cs
- ProtocolsConfiguration.cs
- HttpProfileGroupBase.cs
- PermissionSetEnumerator.cs
- ISCIIEncoding.cs
- RoleServiceManager.cs
- BitmapDecoder.cs
- SettingsBase.cs
- StylusPointCollection.cs
- FormatterConverter.cs
- Animatable.cs
- QualifierSet.cs
- SqlCacheDependency.cs
- PrePostDescendentsWalker.cs
- DataGridViewRowsRemovedEventArgs.cs
- Pair.cs
- ObjectToken.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- input.cs
- DynamicDiscoSearcher.cs
- DrawingGroupDrawingContext.cs
- ProgressBar.cs
- FormCollection.cs
- JsonEncodingStreamWrapper.cs
- NotificationContext.cs
- ExitEventArgs.cs
- FormatterConverter.cs
- NodeInfo.cs
- ResponseBodyWriter.cs
- BitmapDecoder.cs
- BindToObject.cs
- CLRBindingWorker.cs
- ProbeDuplexAsyncResult.cs
- WebRequestModuleElementCollection.cs
- WebHeaderCollection.cs
- InnerItemCollectionView.cs
- ImageListStreamer.cs
- TextCharacters.cs
- Roles.cs
- TableItemPatternIdentifiers.cs
- WebPartConnectionsConfigureVerb.cs
- DetailsViewUpdatedEventArgs.cs
- StylusButtonEventArgs.cs
- Accessors.cs
- InvalidCastException.cs
- InputLanguageSource.cs
- TextDecorations.cs
- DurableInstancingOptions.cs
- SqlErrorCollection.cs
- CellPartitioner.cs
- IdentifierCollection.cs
- QuarticEase.cs
- BigInt.cs
- MultiSelectRootGridEntry.cs
- TreeWalker.cs
- controlskin.cs
- UpdatePanelTrigger.cs
- WmlMobileTextWriter.cs
- ListDataHelper.cs
- DataControlExtensions.cs
- AutomationFocusChangedEventArgs.cs
- SetterBase.cs
- BookmarkOptionsHelper.cs
- DetailsViewDeletedEventArgs.cs
- DataGridViewCellStyleConverter.cs
- Assembly.cs
- RowSpanVector.cs
- Triangle.cs
- ITextView.cs
- QilLiteral.cs
- TableDetailsRow.cs
- ExpressionBindings.cs
- EntityDesignerBuildProvider.cs
- SpeechAudioFormatInfo.cs
- SafeSecurityHandles.cs
- RepeaterItem.cs
- RegularExpressionValidator.cs
- ReadOnlyTernaryTree.cs
- MethodCallConverter.cs
- KnownColorTable.cs
- EndOfStreamException.cs
- DeploymentExceptionMapper.cs
- FormViewInsertedEventArgs.cs
- CTreeGenerator.cs
- SHA256.cs
- WebDisplayNameAttribute.cs
- TextElementEnumerator.cs
- ListViewItem.cs
- SamlAuthenticationClaimResource.cs
- MultiPropertyDescriptorGridEntry.cs
- TransformerConfigurationWizardBase.cs
- UpDownBase.cs
- InputBuffer.cs
- HostedTcpTransportManager.cs
- ContentTypeSettingDispatchMessageFormatter.cs