Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / Visitors.cs / 1305376 / Visitors.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using pc = System.Data.Query.PlanCompiler; // To be able to use PlanCompiler.Assert instead of Debug.Assert in this class. using System.Data; using System.Globalization; namespace System.Data.Query.InternalTrees { ////// Simple implemenation of the BasicOpVisitor interface. /// internal abstract class BasicOpVisitor { ////// Default constructor. /// internal BasicOpVisitor() { } #region Visitor Helpers ////// Visit the children of this Node /// /// The Node that references the Op protected virtual void VisitChildren(Node n) { foreach (Node chi in n.Children) { VisitNode(chi); } } ////// Visit the children of this Node. but in reverse order /// /// The current node protected virtual void VisitChildrenReverse(Node n) { for (int i = n.Children.Count - 1; i >= 0; i--) { VisitNode(n.Children[i]); } } ////// Visit this node /// /// internal virtual void VisitNode(Node n) { n.Op.Accept(this, n); } ////// Default node visitor /// /// protected virtual void VisitDefault(Node n) { VisitChildren(n); } ////// Default handler for all constantOps /// /// the constant op /// the node protected virtual void VisitConstantOp(ConstantBaseOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Default handler for all TableOps /// /// /// protected virtual void VisitTableOp(ScanTableBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all JoinOps /// /// join op /// protected virtual void VisitJoinOp(JoinBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all ApplyOps /// /// apply op /// protected virtual void VisitApplyOp(ApplyBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all SetOps /// /// set op /// protected virtual void VisitSetOp(SetOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all SortOps /// /// sort op /// protected virtual void VisitSortOp(SortBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all GroupBy ops /// /// sort op /// protected virtual void VisitGroupByOp(GroupByBaseOp op, Node n) { VisitRelOpDefault(op, n); } #endregion #region BasicOpVisitor Members ////// Trap method for unrecognized Op types /// /// The Op being visited /// The Node that references the Op public virtual void Visit(Op op, Node n) { throw new NotSupportedException(System.Data.Entity.Strings.Iqt_General_UnsupportedOp(op.GetType().FullName)); } #region ScalarOps protected virtual void VisitScalarOpDefault(ScalarOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for ConstantOp /// /// The ConstantOp being visited /// The Node that references the Op public virtual void Visit(ConstantOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for NullOp /// /// The NullOp being visited /// The Node that references the Op public virtual void Visit(NullOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for NullSentinelOp /// /// The NullSentinelOp being visited /// The Node that references the Op public virtual void Visit(NullSentinelOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for InternalConstantOp /// /// The InternalConstantOp being visited /// The Node that references the Op public virtual void Visit(InternalConstantOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for ConstantPredicateOp /// /// The ConstantPredicateOp being visited /// The Node that references the Op public virtual void Visit(ConstantPredicateOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for FunctionOp /// /// The FunctionOp being visited /// The Node that references the Op public virtual void Visit(FunctionOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for PropertyOp /// /// The PropertyOp being visited /// The Node that references the Op public virtual void Visit(PropertyOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for RelPropertyOp /// /// The RelPropertyOp being visited /// The Node that references the Op public virtual void Visit(RelPropertyOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for CaseOp /// /// The CaseOp being visited /// The Node that references the Op public virtual void Visit(CaseOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ComparisonOp /// /// The ComparisonOp being visited /// The Node that references the Op public virtual void Visit(ComparisonOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for LikeOp /// /// The LikeOp being visited /// The Node that references the Op public virtual void Visit(LikeOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for AggregateOp /// /// The AggregateOp being visited /// The Node that references the Op public virtual void Visit(AggregateOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewInstanceOp /// /// The NewInstanceOp being visited /// The Node that references the Op public virtual void Visit(NewInstanceOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewEntityOp /// /// The NewEntityOp being visited /// The Node that references the Op public virtual void Visit(NewEntityOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for DiscriminatedNewInstanceOp /// /// The DiscriminatedNewInstanceOp being visited /// The Node that references the Op public virtual void Visit(DiscriminatedNewEntityOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewMultisetOp /// /// The NewMultisetOp being visited /// The Node that references the Op public virtual void Visit(NewMultisetOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewRecordOp /// /// The NewRecordOp being visited /// The Node that references the Op public virtual void Visit(NewRecordOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for RefOp /// /// The RefOp being visited /// The Node that references the Op public virtual void Visit(RefOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for VarRefOp /// /// The VarRefOp being visited /// The Node that references the Op public virtual void Visit(VarRefOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ConditionalOp /// /// The ConditionalOp being visited /// The Node that references the Op public virtual void Visit(ConditionalOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ArithmeticOp /// /// The ArithmeticOp being visited /// The Node that references the Op public virtual void Visit(ArithmeticOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for TreatOp /// /// The TreatOp being visited /// The Node that references the Op public virtual void Visit(TreatOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for CastOp /// /// The CastOp being visited /// The Node that references the Op public virtual void Visit(CastOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for SoftCastOp /// /// The SoftCastOp being visited /// The Node that references the Op public virtual void Visit(SoftCastOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for IsOp /// /// The IsOp being visited /// The Node that references the Op public virtual void Visit(IsOfOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ExistsOp /// /// The ExistsOp being visited /// The Node that references the Op public virtual void Visit(ExistsOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ElementOp /// /// The ElementOp being visited /// The Node that references the Op public virtual void Visit(ElementOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for GetEntityRefOp /// /// The GetEntityRefOp being visited /// The Node that references the Op public virtual void Visit(GetEntityRefOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for GetRefKeyOp /// /// The GetRefKeyOp being visited /// The Node that references the Op public virtual void Visit(GetRefKeyOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NestOp /// /// The NestOp being visited /// The Node that references the Op public virtual void Visit(CollectOp op, Node n) { VisitScalarOpDefault(op, n); } public virtual void Visit(DerefOp op, Node n) { VisitScalarOpDefault(op, n); } public virtual void Visit(NavigateOp op, Node n) { VisitScalarOpDefault(op, n); } #endregion #region AncillaryOps protected virtual void VisitAncillaryOpDefault(AncillaryOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for VarDefOp /// /// The VarDefOp being visited /// The Node that references the Op public virtual void Visit(VarDefOp op, Node n) { VisitAncillaryOpDefault(op, n); } ////// Visitor pattern method for VarDefListOp /// /// The VarDefListOp being visited /// The Node that references the Op public virtual void Visit(VarDefListOp op, Node n) { VisitAncillaryOpDefault(op, n); } #endregion #region RelOps protected virtual void VisitRelOpDefault(RelOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for ScanTableOp /// /// The ScanTableOp being visited /// The Node that references the Op public virtual void Visit(ScanTableOp op, Node n) { VisitTableOp(op, n); } ////// Visitor pattern method for ScanViewOp /// /// The ScanViewOp being visited /// The Node that references the Op public virtual void Visit(ScanViewOp op, Node n) { VisitTableOp(op, n); } ////// Visitor pattern method for UnnestOp /// /// The UnnestOp being visited /// The Node that references the Op public virtual void Visit(UnnestOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for ProjectOp /// /// The ProjectOp being visited /// The Node that references the Op public virtual void Visit(ProjectOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for FilterOp /// /// The FilterOp being visited /// The Node that references the Op public virtual void Visit(FilterOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for SortOp /// /// The SortOp being visited /// The Node that references the Op public virtual void Visit(SortOp op, Node n) { VisitSortOp(op, n); } ////// Visitor pattern method for ConstrainedSortOp /// /// The ConstrainedSortOp being visited /// The Node that references the Op public virtual void Visit(ConstrainedSortOp op, Node n) { VisitSortOp(op, n); } ////// Visitor pattern method for GroupByOp /// /// The GroupByOp being visited /// The Node that references the Op public virtual void Visit(GroupByOp op, Node n) { VisitGroupByOp(op, n); } ////// Visitor pattern method for GroupByIntoOp /// /// The GroupByIntoOp being visited /// The Node that references the Op public virtual void Visit(GroupByIntoOp op, Node n) { VisitGroupByOp(op, n); } ////// Visitor pattern method for CrossJoinOp /// /// The CrossJoinOp being visited /// The Node that references the Op public virtual void Visit(CrossJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for InnerJoinOp /// /// The InnerJoinOp being visited /// The Node that references the Op public virtual void Visit(InnerJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for LeftOuterJoinOp /// /// The LeftOuterJoinOp being visited /// The Node that references the Op public virtual void Visit(LeftOuterJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for FullOuterJoinOp /// /// The FullOuterJoinOp being visited /// The Node that references the Op public virtual void Visit(FullOuterJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for CrossApplyOp /// /// The CrossApplyOp being visited /// The Node that references the Op public virtual void Visit(CrossApplyOp op, Node n) { VisitApplyOp(op, n); } ////// Visitor pattern method for OuterApplyOp /// /// The OuterApplyOp being visited /// The Node that references the Op public virtual void Visit(OuterApplyOp op, Node n) { VisitApplyOp(op, n); } ////// Visitor pattern method for UnionAllOp /// /// The UnionAllOp being visited /// The Node that references the Op public virtual void Visit(UnionAllOp op, Node n) { VisitSetOp(op, n); } ////// Visitor pattern method for IntersectOp /// /// The IntersectOp being visited /// The Node that references the Op public virtual void Visit(IntersectOp op, Node n) { VisitSetOp(op, n); } ////// Visitor pattern method for ExceptOp /// /// The ExceptOp being visited /// The Node that references the Op public virtual void Visit(ExceptOp op, Node n) { VisitSetOp(op, n); } ////// Visitor pattern method for DistinctOp /// /// The DistinctOp being visited /// The Node that references the Op public virtual void Visit(DistinctOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for SingleRowOp /// /// The SingleRowOp being visited /// The Node that references the Op public virtual void Visit(SingleRowOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for SingleRowTableOp /// /// The SingleRowTableOp being visited /// The Node that references the Op public virtual void Visit(SingleRowTableOp op, Node n) { VisitRelOpDefault(op, n); } #endregion #region PhysicalOps protected virtual void VisitPhysicalOpDefault(PhysicalOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for PhysicalProjectOp /// /// The op being visited /// The Node that references the Op public virtual void Visit(PhysicalProjectOp op, Node n) { VisitPhysicalOpDefault(op, n); } #region NestOps ////// Common handling for all nestOps /// /// nest op /// protected virtual void VisitNestOp(NestBaseOp op, Node n) { VisitPhysicalOpDefault(op, n); } ////// Visitor pattern method for SingleStreamNestOp /// /// The op being visited /// The Node that references the Op public virtual void Visit(SingleStreamNestOp op, Node n) { VisitNestOp(op, n); } ////// Visitor pattern method for MultistreamNestOp /// /// The op being visited /// The Node that references the Op public virtual void Visit(MultiStreamNestOp op, Node n) { VisitNestOp(op, n); } #endregion #endregion #endregion } ////// Simple implementation of the BasicOpVisitorOfT interface"/> /// ///type parameter internal abstract class BasicOpVisitorOfT{ #region visitor helpers /// /// Simply iterates over all children, and manages any updates /// /// The current node protected virtual void VisitChildren(Node n) { for (int i = 0; i < n.Children.Count; i++) { VisitNode(n.Children[i]); } } ////// Simply iterates over all children, and manages any updates, but in reverse order /// /// The current node protected virtual void VisitChildrenReverse(Node n) { for (int i = n.Children.Count - 1; i >= 0; i--) { VisitNode(n.Children[i]); } } ////// Simple wrapper to invoke the appropriate action on a node /// /// the node to process ///internal TResultType VisitNode(Node n) { // Invoke the visitor return n.Op.Accept (this, n); } /// /// A default processor for any node. Visits the children and returns itself unmodified. /// /// the node to process ///a potentially new node protected virtual TResultType VisitDefault(Node n) { VisitChildren(n); return default(TResultType); } #endregion ////// No processing yet for this node - raises an exception /// /// internal virtual TResultType Unimplemented(Node n) { pc.PlanCompiler.Assert(false, "Not implemented op type"); return default(TResultType); } ////// Catch-all processor - raises an exception /// /// /// ///public virtual TResultType Visit(Op op, Node n) { return Unimplemented(n); } #region AncillaryOp Visitors /// /// A default processor for all AncillaryOps. /// /// Allows new visitors to just override this to handle all AncillaryOps /// /// the AncillaryOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitAncillaryOpDefault(AncillaryOp op, Node n) { return VisitDefault(n); } ////// VarDefOp /// /// /// ///public virtual TResultType Visit(VarDefOp op, Node n) { return VisitAncillaryOpDefault(op, n); } /// /// VarDefListOp /// /// /// ///public virtual TResultType Visit(VarDefListOp op, Node n) { return VisitAncillaryOpDefault(op, n); } #endregion #region PhysicalOp Visitors /// /// A default processor for all PhysicalOps. /// /// Allows new visitors to just override this to handle all PhysicalOps /// /// the PhysicalOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitPhysicalOpDefault(PhysicalOp op, Node n) { return VisitDefault(n); } ////// PhysicalProjectOp /// /// /// ///public virtual TResultType Visit(PhysicalProjectOp op, Node n) { return VisitPhysicalOpDefault(op, n); } #region NestOp Visitors /// /// A default processor for all NestOps. /// /// Allows new visitors to just override this to handle all NestOps /// /// the NestOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitNestOp(NestBaseOp op, Node n) { return VisitPhysicalOpDefault(op, n); } ////// SingleStreamNestOp /// /// /// ///public virtual TResultType Visit(SingleStreamNestOp op, Node n) { return VisitNestOp(op, n); } /// /// MultiStreamNestOp /// /// /// ///public virtual TResultType Visit(MultiStreamNestOp op, Node n) { return VisitNestOp(op, n); } #endregion #endregion #region RelOp Visitors /// /// A default processor for all RelOps. /// /// Allows new visitors to just override this to handle all RelOps /// /// the RelOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitRelOpDefault(RelOp op, Node n) { return VisitDefault(n); } #region ApplyOp Visitors ////// Common handling for all ApplyOps /// /// the ApplyOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitApplyOp(ApplyBaseOp op, Node n) { return VisitRelOpDefault(op, n); } ////// CrossApply /// /// /// ///public virtual TResultType Visit(CrossApplyOp op, Node n) { return VisitApplyOp(op, n); } /// /// OuterApply /// /// /// ///public virtual TResultType Visit(OuterApplyOp op, Node n) { return VisitApplyOp(op, n); } #endregion #region JoinOp Visitors /// /// A default processor for all JoinOps. /// /// Allows new visitors to just override this to handle all JoinOps. /// /// the JoinOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitJoinOp(JoinBaseOp op, Node n) { return VisitRelOpDefault(op, n); } ////// CrossJoin /// /// /// ///public virtual TResultType Visit(CrossJoinOp op, Node n) { return VisitJoinOp(op, n); } /// /// FullOuterJoin /// /// /// ///public virtual TResultType Visit(FullOuterJoinOp op, Node n) { return VisitJoinOp(op, n); } /// /// LeftOuterJoin /// /// /// ///public virtual TResultType Visit(LeftOuterJoinOp op, Node n) { return VisitJoinOp(op, n); } /// /// InnerJoin /// /// /// ///public virtual TResultType Visit(InnerJoinOp op, Node n) { return VisitJoinOp(op, n); } #endregion #region SetOp Visitors /// /// A default processor for all SetOps. /// /// Allows new visitors to just override this to handle all SetOps. /// /// the SetOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitSetOp(SetOp op, Node n) { return VisitRelOpDefault(op, n); } ////// Except /// /// /// ///public virtual TResultType Visit(ExceptOp op, Node n) { return VisitSetOp(op, n); } /// /// Intersect /// /// /// ///public virtual TResultType Visit(IntersectOp op, Node n) { return VisitSetOp(op, n); } /// /// UnionAll /// /// /// ///public virtual TResultType Visit(UnionAllOp op, Node n) { return VisitSetOp(op, n); } #endregion /// /// Distinct /// /// /// ///public virtual TResultType Visit(DistinctOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// FilterOp /// /// /// ///public virtual TResultType Visit(FilterOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// GroupByBaseOp /// /// /// ///protected virtual TResultType VisitGroupByOp(GroupByBaseOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// GroupByOp /// /// /// ///public virtual TResultType Visit(GroupByOp op, Node n) { return VisitGroupByOp(op, n); } /// /// GroupByIntoOp /// /// /// ///public virtual TResultType Visit(GroupByIntoOp op, Node n) { return VisitGroupByOp(op, n); } /// /// ProjectOp /// /// /// ///public virtual TResultType Visit(ProjectOp op, Node n) { return VisitRelOpDefault(op, n); } #region TableOps /// /// Default handler for all TableOps /// /// /// ///protected virtual TResultType VisitTableOp(ScanTableBaseOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// ScanTableOp /// /// /// ///public virtual TResultType Visit(ScanTableOp op, Node n) { return VisitTableOp(op, n); } /// /// ScanViewOp /// /// /// ///public virtual TResultType Visit(ScanViewOp op, Node n) { return VisitTableOp(op, n); } #endregion /// /// Visitor pattern method for SingleRowOp /// /// The SingleRowOp being visited /// The Node that references the Op ///public virtual TResultType Visit(SingleRowOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// Visitor pattern method for SingleRowTableOp /// /// The SingleRowTableOp being visited /// The Node that references the Op ///public virtual TResultType Visit(SingleRowTableOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// A default processor for all SortOps. /// /// Allows new visitors to just override this to handle ConstrainedSortOp/SortOp. /// /// the SetOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitSortOp(SortBaseOp op, Node n) { return VisitRelOpDefault(op, n); } ////// SortOp /// /// /// ///public virtual TResultType Visit(SortOp op, Node n) { return VisitSortOp(op, n); } /// /// ConstrainedSortOp /// /// /// ///public virtual TResultType Visit(ConstrainedSortOp op, Node n) { return VisitSortOp(op, n); } /// /// UnnestOp /// /// /// ///public virtual TResultType Visit(UnnestOp op, Node n) { return VisitRelOpDefault(op, n); } #endregion #region ScalarOp Visitors /// /// A default processor for all ScalarOps. /// /// Allows new visitors to just override this to handle all ScalarOps /// /// the ScalarOp /// the node to process ///a potentially new node protected virtual TResultType VisitScalarOpDefault(ScalarOp op, Node n) { return VisitDefault(n); } ////// Default handler for all constant Ops /// /// /// ///protected virtual TResultType VisitConstantOp(ConstantBaseOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// AggregateOp /// /// /// ///public virtual TResultType Visit(AggregateOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ArithmeticOp /// /// /// ///public virtual TResultType Visit(ArithmeticOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// CaseOp /// /// /// ///public virtual TResultType Visit(CaseOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// CastOp /// /// /// ///public virtual TResultType Visit(CastOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// SoftCastOp /// /// /// ///public virtual TResultType Visit(SoftCastOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NestOp /// /// /// ///public virtual TResultType Visit(CollectOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ComparisonOp /// /// /// ///public virtual TResultType Visit(ComparisonOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ConditionalOp /// /// /// ///public virtual TResultType Visit(ConditionalOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ConstantOp /// /// /// ///public virtual TResultType Visit(ConstantOp op, Node n) { return VisitConstantOp(op, n); } /// /// ConstantPredicateOp /// /// /// ///public virtual TResultType Visit(ConstantPredicateOp op, Node n) { return VisitConstantOp(op, n); } /// /// ElementOp /// /// /// ///public virtual TResultType Visit(ElementOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ExistsOp /// /// /// ///public virtual TResultType Visit(ExistsOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// FunctionOp /// /// /// ///public virtual TResultType Visit(FunctionOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// GetEntityRefOp /// /// /// ///public virtual TResultType Visit(GetEntityRefOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// GetRefKeyOp /// /// /// ///public virtual TResultType Visit(GetRefKeyOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// InternalConstantOp /// /// /// ///public virtual TResultType Visit(InternalConstantOp op, Node n) { return VisitConstantOp(op, n); } /// /// IsOfOp /// /// /// ///public virtual TResultType Visit(IsOfOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// LikeOp /// /// /// ///public virtual TResultType Visit(LikeOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewEntityOp /// /// /// ///public virtual TResultType Visit(NewEntityOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewInstanceOp /// /// /// ///public virtual TResultType Visit(NewInstanceOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// DiscriminatedNewInstanceOp /// /// /// ///public virtual TResultType Visit(DiscriminatedNewEntityOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewMultisetOp /// /// /// ///public virtual TResultType Visit(NewMultisetOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewRecordOp /// /// /// ///public virtual TResultType Visit(NewRecordOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NullOp /// /// /// ///public virtual TResultType Visit(NullOp op, Node n) { return VisitConstantOp(op, n); } /// /// NullSentinelOp /// /// /// ///public virtual TResultType Visit(NullSentinelOp op, Node n) { return VisitConstantOp(op, n); } /// /// PropertyOp /// /// /// ///public virtual TResultType Visit(PropertyOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// RelPropertyOp /// /// /// ///public virtual TResultType Visit(RelPropertyOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// RefOp /// /// /// ///public virtual TResultType Visit(RefOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// TreatOp /// /// /// ///public virtual TResultType Visit(TreatOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// VarRefOp /// /// /// ///public virtual TResultType Visit(VarRefOp op, Node n) { return VisitScalarOpDefault(op, n); } public virtual TResultType Visit(DerefOp op, Node n) { return VisitScalarOpDefault(op, n); } public virtual TResultType Visit(NavigateOp op, Node n) { return VisitScalarOpDefault(op, n); } #endregion } /// /// A visitor implementation that allows subtrees to be modified (in a bottom-up /// fashion) /// internal abstract class BasicOpVisitorOfNode : BasicOpVisitorOfT{ #region visitor helpers /// /// Simply iterates over all children, and manages any updates /// /// The current node protected override void VisitChildren(Node n) { for (int i = 0; i < n.Children.Count; i++) { n.Children[i] = VisitNode(n.Children[i]); } } ////// Simply iterates over all children, and manages any updates, but in reverse order /// /// The current node protected override void VisitChildrenReverse(Node n) { for (int i = n.Children.Count - 1; i >= 0; i--) { n.Children[i] = VisitNode(n.Children[i]); } } ////// A default processor for any node. Visits the children and returns itself unmodified. /// /// the node to process ///a potentially new node protected override Node VisitDefault(Node n) { VisitChildren(n); return n; } #endregion #region AncillaryOp Visitors ////// A default processor for all AncillaryOps. /// /// Allows new visitors to just override this to handle all AncillaryOps /// /// the AncillaryOp /// the node to process ///a potentially modified subtree protected override Node VisitAncillaryOpDefault(AncillaryOp op, Node n) { return VisitDefault(n); } #endregion #region PhysicalOp Visitors ////// A default processor for all PhysicalOps. /// /// Allows new visitors to just override this to handle all PhysicalOps /// /// the PhysicalOp /// the node to process ///a potentially modified subtree protected override Node VisitPhysicalOpDefault(PhysicalOp op, Node n) { return VisitDefault(n); } #endregion #region RelOp Visitors ////// A default processor for all RelOps. /// /// Allows new visitors to just override this to handle all RelOps /// /// the RelOp /// the node to process ///a potentially modified subtree protected override Node VisitRelOpDefault(RelOp op, Node n) { return VisitDefault(n); } #endregion #region ScalarOp Visitors ////// A default processor for all ScalarOps. /// /// Allows new visitors to just override this to handle all ScalarOps /// /// the ScalarOp /// the node to process ///a potentially new node protected override Node VisitScalarOpDefault(ScalarOp op, Node n) { return VisitDefault(n); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using pc = System.Data.Query.PlanCompiler; // To be able to use PlanCompiler.Assert instead of Debug.Assert in this class. using System.Data; using System.Globalization; namespace System.Data.Query.InternalTrees { ////// Simple implemenation of the BasicOpVisitor interface. /// internal abstract class BasicOpVisitor { ////// Default constructor. /// internal BasicOpVisitor() { } #region Visitor Helpers ////// Visit the children of this Node /// /// The Node that references the Op protected virtual void VisitChildren(Node n) { foreach (Node chi in n.Children) { VisitNode(chi); } } ////// Visit the children of this Node. but in reverse order /// /// The current node protected virtual void VisitChildrenReverse(Node n) { for (int i = n.Children.Count - 1; i >= 0; i--) { VisitNode(n.Children[i]); } } ////// Visit this node /// /// internal virtual void VisitNode(Node n) { n.Op.Accept(this, n); } ////// Default node visitor /// /// protected virtual void VisitDefault(Node n) { VisitChildren(n); } ////// Default handler for all constantOps /// /// the constant op /// the node protected virtual void VisitConstantOp(ConstantBaseOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Default handler for all TableOps /// /// /// protected virtual void VisitTableOp(ScanTableBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all JoinOps /// /// join op /// protected virtual void VisitJoinOp(JoinBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all ApplyOps /// /// apply op /// protected virtual void VisitApplyOp(ApplyBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all SetOps /// /// set op /// protected virtual void VisitSetOp(SetOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all SortOps /// /// sort op /// protected virtual void VisitSortOp(SortBaseOp op, Node n) { VisitRelOpDefault(op, n); } ////// Default handler for all GroupBy ops /// /// sort op /// protected virtual void VisitGroupByOp(GroupByBaseOp op, Node n) { VisitRelOpDefault(op, n); } #endregion #region BasicOpVisitor Members ////// Trap method for unrecognized Op types /// /// The Op being visited /// The Node that references the Op public virtual void Visit(Op op, Node n) { throw new NotSupportedException(System.Data.Entity.Strings.Iqt_General_UnsupportedOp(op.GetType().FullName)); } #region ScalarOps protected virtual void VisitScalarOpDefault(ScalarOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for ConstantOp /// /// The ConstantOp being visited /// The Node that references the Op public virtual void Visit(ConstantOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for NullOp /// /// The NullOp being visited /// The Node that references the Op public virtual void Visit(NullOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for NullSentinelOp /// /// The NullSentinelOp being visited /// The Node that references the Op public virtual void Visit(NullSentinelOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for InternalConstantOp /// /// The InternalConstantOp being visited /// The Node that references the Op public virtual void Visit(InternalConstantOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for ConstantPredicateOp /// /// The ConstantPredicateOp being visited /// The Node that references the Op public virtual void Visit(ConstantPredicateOp op, Node n) { VisitConstantOp(op, n); } ////// Visitor pattern method for FunctionOp /// /// The FunctionOp being visited /// The Node that references the Op public virtual void Visit(FunctionOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for PropertyOp /// /// The PropertyOp being visited /// The Node that references the Op public virtual void Visit(PropertyOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for RelPropertyOp /// /// The RelPropertyOp being visited /// The Node that references the Op public virtual void Visit(RelPropertyOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for CaseOp /// /// The CaseOp being visited /// The Node that references the Op public virtual void Visit(CaseOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ComparisonOp /// /// The ComparisonOp being visited /// The Node that references the Op public virtual void Visit(ComparisonOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for LikeOp /// /// The LikeOp being visited /// The Node that references the Op public virtual void Visit(LikeOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for AggregateOp /// /// The AggregateOp being visited /// The Node that references the Op public virtual void Visit(AggregateOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewInstanceOp /// /// The NewInstanceOp being visited /// The Node that references the Op public virtual void Visit(NewInstanceOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewEntityOp /// /// The NewEntityOp being visited /// The Node that references the Op public virtual void Visit(NewEntityOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for DiscriminatedNewInstanceOp /// /// The DiscriminatedNewInstanceOp being visited /// The Node that references the Op public virtual void Visit(DiscriminatedNewEntityOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewMultisetOp /// /// The NewMultisetOp being visited /// The Node that references the Op public virtual void Visit(NewMultisetOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NewRecordOp /// /// The NewRecordOp being visited /// The Node that references the Op public virtual void Visit(NewRecordOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for RefOp /// /// The RefOp being visited /// The Node that references the Op public virtual void Visit(RefOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for VarRefOp /// /// The VarRefOp being visited /// The Node that references the Op public virtual void Visit(VarRefOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ConditionalOp /// /// The ConditionalOp being visited /// The Node that references the Op public virtual void Visit(ConditionalOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ArithmeticOp /// /// The ArithmeticOp being visited /// The Node that references the Op public virtual void Visit(ArithmeticOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for TreatOp /// /// The TreatOp being visited /// The Node that references the Op public virtual void Visit(TreatOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for CastOp /// /// The CastOp being visited /// The Node that references the Op public virtual void Visit(CastOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for SoftCastOp /// /// The SoftCastOp being visited /// The Node that references the Op public virtual void Visit(SoftCastOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for IsOp /// /// The IsOp being visited /// The Node that references the Op public virtual void Visit(IsOfOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ExistsOp /// /// The ExistsOp being visited /// The Node that references the Op public virtual void Visit(ExistsOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for ElementOp /// /// The ElementOp being visited /// The Node that references the Op public virtual void Visit(ElementOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for GetEntityRefOp /// /// The GetEntityRefOp being visited /// The Node that references the Op public virtual void Visit(GetEntityRefOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for GetRefKeyOp /// /// The GetRefKeyOp being visited /// The Node that references the Op public virtual void Visit(GetRefKeyOp op, Node n) { VisitScalarOpDefault(op, n); } ////// Visitor pattern method for NestOp /// /// The NestOp being visited /// The Node that references the Op public virtual void Visit(CollectOp op, Node n) { VisitScalarOpDefault(op, n); } public virtual void Visit(DerefOp op, Node n) { VisitScalarOpDefault(op, n); } public virtual void Visit(NavigateOp op, Node n) { VisitScalarOpDefault(op, n); } #endregion #region AncillaryOps protected virtual void VisitAncillaryOpDefault(AncillaryOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for VarDefOp /// /// The VarDefOp being visited /// The Node that references the Op public virtual void Visit(VarDefOp op, Node n) { VisitAncillaryOpDefault(op, n); } ////// Visitor pattern method for VarDefListOp /// /// The VarDefListOp being visited /// The Node that references the Op public virtual void Visit(VarDefListOp op, Node n) { VisitAncillaryOpDefault(op, n); } #endregion #region RelOps protected virtual void VisitRelOpDefault(RelOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for ScanTableOp /// /// The ScanTableOp being visited /// The Node that references the Op public virtual void Visit(ScanTableOp op, Node n) { VisitTableOp(op, n); } ////// Visitor pattern method for ScanViewOp /// /// The ScanViewOp being visited /// The Node that references the Op public virtual void Visit(ScanViewOp op, Node n) { VisitTableOp(op, n); } ////// Visitor pattern method for UnnestOp /// /// The UnnestOp being visited /// The Node that references the Op public virtual void Visit(UnnestOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for ProjectOp /// /// The ProjectOp being visited /// The Node that references the Op public virtual void Visit(ProjectOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for FilterOp /// /// The FilterOp being visited /// The Node that references the Op public virtual void Visit(FilterOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for SortOp /// /// The SortOp being visited /// The Node that references the Op public virtual void Visit(SortOp op, Node n) { VisitSortOp(op, n); } ////// Visitor pattern method for ConstrainedSortOp /// /// The ConstrainedSortOp being visited /// The Node that references the Op public virtual void Visit(ConstrainedSortOp op, Node n) { VisitSortOp(op, n); } ////// Visitor pattern method for GroupByOp /// /// The GroupByOp being visited /// The Node that references the Op public virtual void Visit(GroupByOp op, Node n) { VisitGroupByOp(op, n); } ////// Visitor pattern method for GroupByIntoOp /// /// The GroupByIntoOp being visited /// The Node that references the Op public virtual void Visit(GroupByIntoOp op, Node n) { VisitGroupByOp(op, n); } ////// Visitor pattern method for CrossJoinOp /// /// The CrossJoinOp being visited /// The Node that references the Op public virtual void Visit(CrossJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for InnerJoinOp /// /// The InnerJoinOp being visited /// The Node that references the Op public virtual void Visit(InnerJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for LeftOuterJoinOp /// /// The LeftOuterJoinOp being visited /// The Node that references the Op public virtual void Visit(LeftOuterJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for FullOuterJoinOp /// /// The FullOuterJoinOp being visited /// The Node that references the Op public virtual void Visit(FullOuterJoinOp op, Node n) { VisitJoinOp(op, n); } ////// Visitor pattern method for CrossApplyOp /// /// The CrossApplyOp being visited /// The Node that references the Op public virtual void Visit(CrossApplyOp op, Node n) { VisitApplyOp(op, n); } ////// Visitor pattern method for OuterApplyOp /// /// The OuterApplyOp being visited /// The Node that references the Op public virtual void Visit(OuterApplyOp op, Node n) { VisitApplyOp(op, n); } ////// Visitor pattern method for UnionAllOp /// /// The UnionAllOp being visited /// The Node that references the Op public virtual void Visit(UnionAllOp op, Node n) { VisitSetOp(op, n); } ////// Visitor pattern method for IntersectOp /// /// The IntersectOp being visited /// The Node that references the Op public virtual void Visit(IntersectOp op, Node n) { VisitSetOp(op, n); } ////// Visitor pattern method for ExceptOp /// /// The ExceptOp being visited /// The Node that references the Op public virtual void Visit(ExceptOp op, Node n) { VisitSetOp(op, n); } ////// Visitor pattern method for DistinctOp /// /// The DistinctOp being visited /// The Node that references the Op public virtual void Visit(DistinctOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for SingleRowOp /// /// The SingleRowOp being visited /// The Node that references the Op public virtual void Visit(SingleRowOp op, Node n) { VisitRelOpDefault(op, n); } ////// Visitor pattern method for SingleRowTableOp /// /// The SingleRowTableOp being visited /// The Node that references the Op public virtual void Visit(SingleRowTableOp op, Node n) { VisitRelOpDefault(op, n); } #endregion #region PhysicalOps protected virtual void VisitPhysicalOpDefault(PhysicalOp op, Node n) { VisitDefault(n); } ////// Visitor pattern method for PhysicalProjectOp /// /// The op being visited /// The Node that references the Op public virtual void Visit(PhysicalProjectOp op, Node n) { VisitPhysicalOpDefault(op, n); } #region NestOps ////// Common handling for all nestOps /// /// nest op /// protected virtual void VisitNestOp(NestBaseOp op, Node n) { VisitPhysicalOpDefault(op, n); } ////// Visitor pattern method for SingleStreamNestOp /// /// The op being visited /// The Node that references the Op public virtual void Visit(SingleStreamNestOp op, Node n) { VisitNestOp(op, n); } ////// Visitor pattern method for MultistreamNestOp /// /// The op being visited /// The Node that references the Op public virtual void Visit(MultiStreamNestOp op, Node n) { VisitNestOp(op, n); } #endregion #endregion #endregion } ////// Simple implementation of the BasicOpVisitorOfT interface"/> /// ///type parameter internal abstract class BasicOpVisitorOfT{ #region visitor helpers /// /// Simply iterates over all children, and manages any updates /// /// The current node protected virtual void VisitChildren(Node n) { for (int i = 0; i < n.Children.Count; i++) { VisitNode(n.Children[i]); } } ////// Simply iterates over all children, and manages any updates, but in reverse order /// /// The current node protected virtual void VisitChildrenReverse(Node n) { for (int i = n.Children.Count - 1; i >= 0; i--) { VisitNode(n.Children[i]); } } ////// Simple wrapper to invoke the appropriate action on a node /// /// the node to process ///internal TResultType VisitNode(Node n) { // Invoke the visitor return n.Op.Accept (this, n); } /// /// A default processor for any node. Visits the children and returns itself unmodified. /// /// the node to process ///a potentially new node protected virtual TResultType VisitDefault(Node n) { VisitChildren(n); return default(TResultType); } #endregion ////// No processing yet for this node - raises an exception /// /// internal virtual TResultType Unimplemented(Node n) { pc.PlanCompiler.Assert(false, "Not implemented op type"); return default(TResultType); } ////// Catch-all processor - raises an exception /// /// /// ///public virtual TResultType Visit(Op op, Node n) { return Unimplemented(n); } #region AncillaryOp Visitors /// /// A default processor for all AncillaryOps. /// /// Allows new visitors to just override this to handle all AncillaryOps /// /// the AncillaryOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitAncillaryOpDefault(AncillaryOp op, Node n) { return VisitDefault(n); } ////// VarDefOp /// /// /// ///public virtual TResultType Visit(VarDefOp op, Node n) { return VisitAncillaryOpDefault(op, n); } /// /// VarDefListOp /// /// /// ///public virtual TResultType Visit(VarDefListOp op, Node n) { return VisitAncillaryOpDefault(op, n); } #endregion #region PhysicalOp Visitors /// /// A default processor for all PhysicalOps. /// /// Allows new visitors to just override this to handle all PhysicalOps /// /// the PhysicalOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitPhysicalOpDefault(PhysicalOp op, Node n) { return VisitDefault(n); } ////// PhysicalProjectOp /// /// /// ///public virtual TResultType Visit(PhysicalProjectOp op, Node n) { return VisitPhysicalOpDefault(op, n); } #region NestOp Visitors /// /// A default processor for all NestOps. /// /// Allows new visitors to just override this to handle all NestOps /// /// the NestOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitNestOp(NestBaseOp op, Node n) { return VisitPhysicalOpDefault(op, n); } ////// SingleStreamNestOp /// /// /// ///public virtual TResultType Visit(SingleStreamNestOp op, Node n) { return VisitNestOp(op, n); } /// /// MultiStreamNestOp /// /// /// ///public virtual TResultType Visit(MultiStreamNestOp op, Node n) { return VisitNestOp(op, n); } #endregion #endregion #region RelOp Visitors /// /// A default processor for all RelOps. /// /// Allows new visitors to just override this to handle all RelOps /// /// the RelOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitRelOpDefault(RelOp op, Node n) { return VisitDefault(n); } #region ApplyOp Visitors ////// Common handling for all ApplyOps /// /// the ApplyOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitApplyOp(ApplyBaseOp op, Node n) { return VisitRelOpDefault(op, n); } ////// CrossApply /// /// /// ///public virtual TResultType Visit(CrossApplyOp op, Node n) { return VisitApplyOp(op, n); } /// /// OuterApply /// /// /// ///public virtual TResultType Visit(OuterApplyOp op, Node n) { return VisitApplyOp(op, n); } #endregion #region JoinOp Visitors /// /// A default processor for all JoinOps. /// /// Allows new visitors to just override this to handle all JoinOps. /// /// the JoinOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitJoinOp(JoinBaseOp op, Node n) { return VisitRelOpDefault(op, n); } ////// CrossJoin /// /// /// ///public virtual TResultType Visit(CrossJoinOp op, Node n) { return VisitJoinOp(op, n); } /// /// FullOuterJoin /// /// /// ///public virtual TResultType Visit(FullOuterJoinOp op, Node n) { return VisitJoinOp(op, n); } /// /// LeftOuterJoin /// /// /// ///public virtual TResultType Visit(LeftOuterJoinOp op, Node n) { return VisitJoinOp(op, n); } /// /// InnerJoin /// /// /// ///public virtual TResultType Visit(InnerJoinOp op, Node n) { return VisitJoinOp(op, n); } #endregion #region SetOp Visitors /// /// A default processor for all SetOps. /// /// Allows new visitors to just override this to handle all SetOps. /// /// the SetOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitSetOp(SetOp op, Node n) { return VisitRelOpDefault(op, n); } ////// Except /// /// /// ///public virtual TResultType Visit(ExceptOp op, Node n) { return VisitSetOp(op, n); } /// /// Intersect /// /// /// ///public virtual TResultType Visit(IntersectOp op, Node n) { return VisitSetOp(op, n); } /// /// UnionAll /// /// /// ///public virtual TResultType Visit(UnionAllOp op, Node n) { return VisitSetOp(op, n); } #endregion /// /// Distinct /// /// /// ///public virtual TResultType Visit(DistinctOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// FilterOp /// /// /// ///public virtual TResultType Visit(FilterOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// GroupByBaseOp /// /// /// ///protected virtual TResultType VisitGroupByOp(GroupByBaseOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// GroupByOp /// /// /// ///public virtual TResultType Visit(GroupByOp op, Node n) { return VisitGroupByOp(op, n); } /// /// GroupByIntoOp /// /// /// ///public virtual TResultType Visit(GroupByIntoOp op, Node n) { return VisitGroupByOp(op, n); } /// /// ProjectOp /// /// /// ///public virtual TResultType Visit(ProjectOp op, Node n) { return VisitRelOpDefault(op, n); } #region TableOps /// /// Default handler for all TableOps /// /// /// ///protected virtual TResultType VisitTableOp(ScanTableBaseOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// ScanTableOp /// /// /// ///public virtual TResultType Visit(ScanTableOp op, Node n) { return VisitTableOp(op, n); } /// /// ScanViewOp /// /// /// ///public virtual TResultType Visit(ScanViewOp op, Node n) { return VisitTableOp(op, n); } #endregion /// /// Visitor pattern method for SingleRowOp /// /// The SingleRowOp being visited /// The Node that references the Op ///public virtual TResultType Visit(SingleRowOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// Visitor pattern method for SingleRowTableOp /// /// The SingleRowTableOp being visited /// The Node that references the Op ///public virtual TResultType Visit(SingleRowTableOp op, Node n) { return VisitRelOpDefault(op, n); } /// /// A default processor for all SortOps. /// /// Allows new visitors to just override this to handle ConstrainedSortOp/SortOp. /// /// the SetOp /// the node to process ///a potentially modified subtree protected virtual TResultType VisitSortOp(SortBaseOp op, Node n) { return VisitRelOpDefault(op, n); } ////// SortOp /// /// /// ///public virtual TResultType Visit(SortOp op, Node n) { return VisitSortOp(op, n); } /// /// ConstrainedSortOp /// /// /// ///public virtual TResultType Visit(ConstrainedSortOp op, Node n) { return VisitSortOp(op, n); } /// /// UnnestOp /// /// /// ///public virtual TResultType Visit(UnnestOp op, Node n) { return VisitRelOpDefault(op, n); } #endregion #region ScalarOp Visitors /// /// A default processor for all ScalarOps. /// /// Allows new visitors to just override this to handle all ScalarOps /// /// the ScalarOp /// the node to process ///a potentially new node protected virtual TResultType VisitScalarOpDefault(ScalarOp op, Node n) { return VisitDefault(n); } ////// Default handler for all constant Ops /// /// /// ///protected virtual TResultType VisitConstantOp(ConstantBaseOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// AggregateOp /// /// /// ///public virtual TResultType Visit(AggregateOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ArithmeticOp /// /// /// ///public virtual TResultType Visit(ArithmeticOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// CaseOp /// /// /// ///public virtual TResultType Visit(CaseOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// CastOp /// /// /// ///public virtual TResultType Visit(CastOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// SoftCastOp /// /// /// ///public virtual TResultType Visit(SoftCastOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NestOp /// /// /// ///public virtual TResultType Visit(CollectOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ComparisonOp /// /// /// ///public virtual TResultType Visit(ComparisonOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ConditionalOp /// /// /// ///public virtual TResultType Visit(ConditionalOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ConstantOp /// /// /// ///public virtual TResultType Visit(ConstantOp op, Node n) { return VisitConstantOp(op, n); } /// /// ConstantPredicateOp /// /// /// ///public virtual TResultType Visit(ConstantPredicateOp op, Node n) { return VisitConstantOp(op, n); } /// /// ElementOp /// /// /// ///public virtual TResultType Visit(ElementOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// ExistsOp /// /// /// ///public virtual TResultType Visit(ExistsOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// FunctionOp /// /// /// ///public virtual TResultType Visit(FunctionOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// GetEntityRefOp /// /// /// ///public virtual TResultType Visit(GetEntityRefOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// GetRefKeyOp /// /// /// ///public virtual TResultType Visit(GetRefKeyOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// InternalConstantOp /// /// /// ///public virtual TResultType Visit(InternalConstantOp op, Node n) { return VisitConstantOp(op, n); } /// /// IsOfOp /// /// /// ///public virtual TResultType Visit(IsOfOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// LikeOp /// /// /// ///public virtual TResultType Visit(LikeOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewEntityOp /// /// /// ///public virtual TResultType Visit(NewEntityOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewInstanceOp /// /// /// ///public virtual TResultType Visit(NewInstanceOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// DiscriminatedNewInstanceOp /// /// /// ///public virtual TResultType Visit(DiscriminatedNewEntityOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewMultisetOp /// /// /// ///public virtual TResultType Visit(NewMultisetOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NewRecordOp /// /// /// ///public virtual TResultType Visit(NewRecordOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// NullOp /// /// /// ///public virtual TResultType Visit(NullOp op, Node n) { return VisitConstantOp(op, n); } /// /// NullSentinelOp /// /// /// ///public virtual TResultType Visit(NullSentinelOp op, Node n) { return VisitConstantOp(op, n); } /// /// PropertyOp /// /// /// ///public virtual TResultType Visit(PropertyOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// RelPropertyOp /// /// /// ///public virtual TResultType Visit(RelPropertyOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// RefOp /// /// /// ///public virtual TResultType Visit(RefOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// TreatOp /// /// /// ///public virtual TResultType Visit(TreatOp op, Node n) { return VisitScalarOpDefault(op, n); } /// /// VarRefOp /// /// /// ///public virtual TResultType Visit(VarRefOp op, Node n) { return VisitScalarOpDefault(op, n); } public virtual TResultType Visit(DerefOp op, Node n) { return VisitScalarOpDefault(op, n); } public virtual TResultType Visit(NavigateOp op, Node n) { return VisitScalarOpDefault(op, n); } #endregion } /// /// A visitor implementation that allows subtrees to be modified (in a bottom-up /// fashion) /// internal abstract class BasicOpVisitorOfNode : BasicOpVisitorOfT{ #region visitor helpers /// /// Simply iterates over all children, and manages any updates /// /// The current node protected override void VisitChildren(Node n) { for (int i = 0; i < n.Children.Count; i++) { n.Children[i] = VisitNode(n.Children[i]); } } ////// Simply iterates over all children, and manages any updates, but in reverse order /// /// The current node protected override void VisitChildrenReverse(Node n) { for (int i = n.Children.Count - 1; i >= 0; i--) { n.Children[i] = VisitNode(n.Children[i]); } } ////// A default processor for any node. Visits the children and returns itself unmodified. /// /// the node to process ///a potentially new node protected override Node VisitDefault(Node n) { VisitChildren(n); return n; } #endregion #region AncillaryOp Visitors ////// A default processor for all AncillaryOps. /// /// Allows new visitors to just override this to handle all AncillaryOps /// /// the AncillaryOp /// the node to process ///a potentially modified subtree protected override Node VisitAncillaryOpDefault(AncillaryOp op, Node n) { return VisitDefault(n); } #endregion #region PhysicalOp Visitors ////// A default processor for all PhysicalOps. /// /// Allows new visitors to just override this to handle all PhysicalOps /// /// the PhysicalOp /// the node to process ///a potentially modified subtree protected override Node VisitPhysicalOpDefault(PhysicalOp op, Node n) { return VisitDefault(n); } #endregion #region RelOp Visitors ////// A default processor for all RelOps. /// /// Allows new visitors to just override this to handle all RelOps /// /// the RelOp /// the node to process ///a potentially modified subtree protected override Node VisitRelOpDefault(RelOp op, Node n) { return VisitDefault(n); } #endregion #region ScalarOp Visitors ////// A default processor for all ScalarOps. /// /// Allows new visitors to just override this to handle all ScalarOps /// /// the ScalarOp /// the node to process ///a potentially new node protected override Node VisitScalarOpDefault(ScalarOp op, Node n) { return VisitDefault(n); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
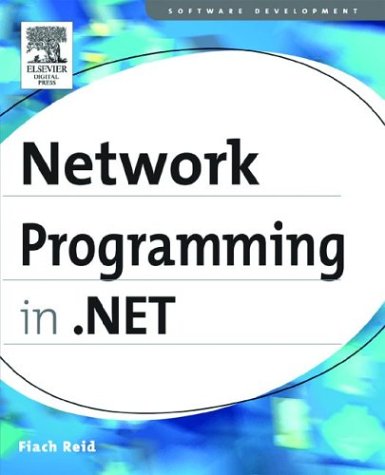
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpApplication.cs
- SplitterEvent.cs
- LayoutTableCell.cs
- KeyValueInternalCollection.cs
- PersistenceTypeAttribute.cs
- SByteStorage.cs
- EDesignUtil.cs
- AccessedThroughPropertyAttribute.cs
- StylusPointPropertyUnit.cs
- XmlSchemaImport.cs
- TextParagraph.cs
- RuleSetBrowserDialog.cs
- SoapHeaderException.cs
- ActiveXMessageFormatter.cs
- NumericPagerField.cs
- ComAdminInterfaces.cs
- SiteMapDataSource.cs
- dsa.cs
- Button.cs
- BasePattern.cs
- GridViewHeaderRowPresenter.cs
- DocumentApplicationState.cs
- DynamicRendererThreadManager.cs
- TypeBuilder.cs
- UpdatePanel.cs
- DropShadowEffect.cs
- SortDescription.cs
- PropertyKey.cs
- Graph.cs
- SqlBuilder.cs
- PeerNameRecordCollection.cs
- CannotUnloadAppDomainException.cs
- DirectoryInfo.cs
- ActionFrame.cs
- DictionaryItemsCollection.cs
- SymmetricKey.cs
- NCryptSafeHandles.cs
- EdmMember.cs
- XmlAnyElementAttributes.cs
- XmlWellformedWriter.cs
- DataRowCollection.cs
- HtmlSelect.cs
- EmptyImpersonationContext.cs
- Win32Interop.cs
- WebPartsPersonalization.cs
- PersonalizationProviderHelper.cs
- CodeDirectionExpression.cs
- CompoundFileIOPermission.cs
- StrokeNodeData.cs
- EmptyStringExpandableObjectConverter.cs
- DataExchangeServiceBinder.cs
- PtsContext.cs
- RedirectionProxy.cs
- SQLInt64Storage.cs
- WebPartVerbCollection.cs
- COSERVERINFO.cs
- TextServicesDisplayAttributePropertyRanges.cs
- DefaultMemberAttribute.cs
- PKCS1MaskGenerationMethod.cs
- SettingsPropertyIsReadOnlyException.cs
- ObjectDataSourceFilteringEventArgs.cs
- COMException.cs
- MissingMemberException.cs
- CodeDOMUtility.cs
- IPEndPoint.cs
- XmlAttributeAttribute.cs
- CharacterHit.cs
- NativeRightsManagementAPIsStructures.cs
- mongolianshape.cs
- MetaTable.cs
- StrongNameUtility.cs
- _DomainName.cs
- DbConnectionInternal.cs
- ScriptMethodAttribute.cs
- ControlPersister.cs
- RuleRefElement.cs
- FixedNode.cs
- XmlSchemaComplexContentRestriction.cs
- ComponentResourceManager.cs
- ChangeInterceptorAttribute.cs
- XPathBuilder.cs
- PermissionSetTriple.cs
- ReverseQueryOperator.cs
- LogRecordSequence.cs
- DataServiceBehavior.cs
- EntityTypeBase.cs
- HyperLinkColumn.cs
- EmptyQuery.cs
- sqlcontext.cs
- KoreanCalendar.cs
- ExpressionCopier.cs
- ServiceTimeoutsBehavior.cs
- RSAPKCS1KeyExchangeFormatter.cs
- BindingMAnagerBase.cs
- DesignerValidationSummaryAdapter.cs
- login.cs
- Bidi.cs
- PrimitiveXmlSerializers.cs
- Trace.cs
- SafeReadContext.cs