Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Utils / ContractUtils.cs / 1305376 / ContractUtils.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Diagnostics; using System.Linq.Expressions; #if SILVERLIGHT using System.Core; #endif namespace System.Dynamic.Utils { // Will be replaced with CLRv4 managed contracts internal static class ContractUtils { internal static Exception Unreachable { get { Debug.Assert(false, "Unreachable"); return new InvalidOperationException("Code supposed to be unreachable"); } } internal static void Requires(bool precondition) { if (!precondition) { throw new ArgumentException(Strings.MethodPreconditionViolated); } } internal static void Requires(bool precondition, string paramName) { Debug.Assert(!string.IsNullOrEmpty(paramName)); if (!precondition) { throw new ArgumentException(Strings.InvalidArgumentValue, paramName); } } internal static void RequiresNotNull(object value, string paramName) { Debug.Assert(!string.IsNullOrEmpty(paramName)); if (value == null) { throw new ArgumentNullException(paramName); } } internal static void RequiresNotEmpty(ICollection collection, string paramName) { RequiresNotNull(collection, paramName); if (collection.Count == 0) { throw new ArgumentException(Strings.NonEmptyCollectionRequired, paramName); } } /// /// Requires the range [offset, offset + count] to be a subset of [0, array.Count]. /// ///Array is ///null .Offset or count are out of range. internal static void RequiresArrayRange(IList array, int offset, int count, string offsetName, string countName) { Debug.Assert(!string.IsNullOrEmpty(offsetName)); Debug.Assert(!string.IsNullOrEmpty(countName)); Debug.Assert(array != null); if (count < 0) throw new ArgumentOutOfRangeException(countName); if (offset < 0 || array.Count - offset < count) throw new ArgumentOutOfRangeException(offsetName); } /// /// Requires the array and all its items to be non-null. /// internal static void RequiresNotNullItems(IList array, string arrayName) { Debug.Assert(arrayName != null); RequiresNotNull(array, arrayName); for (int i = 0; i < array.Count; i++) { if (array[i] == null) { throw new ArgumentNullException(string.Format(System.Globalization.CultureInfo.CurrentCulture, "{0}[{1}]", arrayName, i)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Diagnostics; using System.Linq.Expressions; #if SILVERLIGHT using System.Core; #endif namespace System.Dynamic.Utils { // Will be replaced with CLRv4 managed contracts internal static class ContractUtils { internal static Exception Unreachable { get { Debug.Assert(false, "Unreachable"); return new InvalidOperationException("Code supposed to be unreachable"); } } internal static void Requires(bool precondition) { if (!precondition) { throw new ArgumentException(Strings.MethodPreconditionViolated); } } internal static void Requires(bool precondition, string paramName) { Debug.Assert(!string.IsNullOrEmpty(paramName)); if (!precondition) { throw new ArgumentException(Strings.InvalidArgumentValue, paramName); } } internal static void RequiresNotNull(object value, string paramName) { Debug.Assert(!string.IsNullOrEmpty(paramName)); if (value == null) { throw new ArgumentNullException(paramName); } } internal static void RequiresNotEmpty (ICollection collection, string paramName) { RequiresNotNull(collection, paramName); if (collection.Count == 0) { throw new ArgumentException(Strings.NonEmptyCollectionRequired, paramName); } } /// /// Requires the range [offset, offset + count] to be a subset of [0, array.Count]. /// ///Array is ///null .Offset or count are out of range. internal static void RequiresArrayRange(IList array, int offset, int count, string offsetName, string countName) { Debug.Assert(!string.IsNullOrEmpty(offsetName)); Debug.Assert(!string.IsNullOrEmpty(countName)); Debug.Assert(array != null); if (count < 0) throw new ArgumentOutOfRangeException(countName); if (offset < 0 || array.Count - offset < count) throw new ArgumentOutOfRangeException(offsetName); } /// /// Requires the array and all its items to be non-null. /// internal static void RequiresNotNullItems(IList array, string arrayName) { Debug.Assert(arrayName != null); RequiresNotNull(array, arrayName); for (int i = 0; i < array.Count; i++) { if (array[i] == null) { throw new ArgumentNullException(string.Format(System.Globalization.CultureInfo.CurrentCulture, "{0}[{1}]", arrayName, i)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
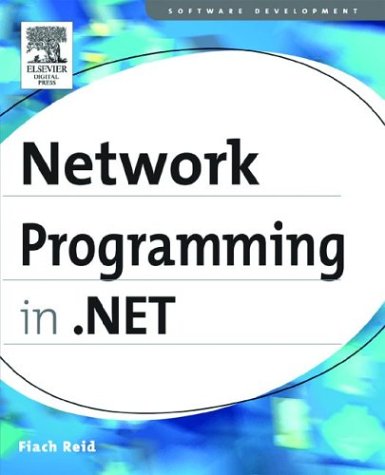
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- cookiecontainer.cs
- DataTableTypeConverter.cs
- Vector.cs
- XPathAxisIterator.cs
- Variant.cs
- SimpleExpression.cs
- CalendarAutoFormatDialog.cs
- VerificationException.cs
- DesignColumn.cs
- PenThreadPool.cs
- IsolatedStorageException.cs
- RealizationDrawingContextWalker.cs
- HtmlWindow.cs
- Bits.cs
- HttpWebResponse.cs
- XmlElementAttribute.cs
- EncryptedReference.cs
- ResourceProviderFactory.cs
- PlainXmlSerializer.cs
- EncodingInfo.cs
- CodeComment.cs
- filewebresponse.cs
- StatusBarItem.cs
- PipeSecurity.cs
- Repeater.cs
- IPAddressCollection.cs
- mda.cs
- codemethodreferenceexpression.cs
- RadioButtonDesigner.cs
- SelectingProviderEventArgs.cs
- ClientBuildManager.cs
- CombinedGeometry.cs
- ScaleTransform.cs
- CommonXSendMessage.cs
- CounterSample.cs
- DataGridViewCheckBoxCell.cs
- TemplateControlParser.cs
- CodeNamespace.cs
- HwndHost.cs
- CodePageUtils.cs
- AnimationClockResource.cs
- FileLogRecordEnumerator.cs
- ExtentKey.cs
- CodeCatchClauseCollection.cs
- WebServiceMethodData.cs
- CodePageEncoding.cs
- SettingsBase.cs
- NetNamedPipeBindingCollectionElement.cs
- PropertyBuilder.cs
- BinaryCommonClasses.cs
- BypassElementCollection.cs
- OdbcUtils.cs
- StringExpressionSet.cs
- WindowsFormsLinkLabel.cs
- DataGridPageChangedEventArgs.cs
- MessageQueueConverter.cs
- ModelTreeManager.cs
- PermissionToken.cs
- TemplateBuilder.cs
- DataBinding.cs
- RuleSetDialog.cs
- Base64Stream.cs
- RestClientProxyHandler.cs
- CollectionChangeEventArgs.cs
- ControlType.cs
- ClientScriptManager.cs
- PropertyGrid.cs
- Wildcard.cs
- BrowserTree.cs
- DeferredBinaryDeserializerExtension.cs
- Point4D.cs
- ToolTipService.cs
- InstancePersistenceException.cs
- BitmapCache.cs
- XAMLParseException.cs
- UnknownBitmapDecoder.cs
- FontFamily.cs
- FileDialogCustomPlacesCollection.cs
- SharedStatics.cs
- CompoundFileReference.cs
- DataGridViewButtonColumn.cs
- EtwProvider.cs
- QilXmlWriter.cs
- DropShadowEffect.cs
- RawStylusInputReport.cs
- PathSegment.cs
- IPEndPoint.cs
- CaseCqlBlock.cs
- ReflectTypeDescriptionProvider.cs
- BaseDataBoundControl.cs
- DictionaryBase.cs
- SecondaryViewProvider.cs
- InstalledFontCollection.cs
- ListComponentEditor.cs
- WriteStateInfoBase.cs
- TripleDESCryptoServiceProvider.cs
- IntegerValidator.cs
- WebConfigurationManager.cs
- SecurityPermission.cs
- TrackingExtract.cs