Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Utils / CollectionExtensions.cs / 1305376 / CollectionExtensions.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; #if !MICROSOFT_SCRIPTING_CORE using System.Diagnostics.Contracts; #endif using System.Runtime.CompilerServices; namespace System.Dynamic.Utils { internal static class CollectionExtensions { ////// Wraps the provided enumerable into a ReadOnlyCollection{T} /// /// Copies all of the data into a new array, so the data can't be /// changed after creation. The exception is if the enumerable is /// already a ReadOnlyCollection{T}, in which case we just return it. /// #if !MICROSOFT_SCRIPTING_CORE [Pure] #endif internal static ReadOnlyCollectionToReadOnly (this IEnumerable enumerable) { if (enumerable == null) { return EmptyReadOnlyCollection .Instance; } var troc = enumerable as TrueReadOnlyCollection ; if (troc != null) { return troc; } var builder = enumerable as ReadOnlyCollectionBuilder ; if (builder != null) { return builder.ToReadOnlyCollection(); } var collection = enumerable as ICollection ; if (collection != null) { int count = collection.Count; if (count == 0) { return EmptyReadOnlyCollection .Instance; } T[] clone = new T[count]; collection.CopyTo(clone, 0); return new TrueReadOnlyCollection (clone); } // ToArray trims the excess space and speeds up access return new TrueReadOnlyCollection (new List (enumerable).ToArray()); } // We could probably improve the hashing here internal static int ListHashCode (this IEnumerable list) { var cmp = EqualityComparer .Default; int h = 6551; foreach (T t in list) { h ^= (h << 5) ^ cmp.GetHashCode(t); } return h; } #if !MICROSOFT_SCRIPTING_CORE [Pure] #endif internal static bool ListEquals (this ICollection first, ICollection second) { if (first.Count != second.Count) { return false; } var cmp = EqualityComparer .Default; var f = first.GetEnumerator(); var s = second.GetEnumerator(); while (f.MoveNext()) { s.MoveNext(); if (!cmp.Equals(f.Current, s.Current)) { return false; } } return true; } internal static IEnumerable Select (this IEnumerable enumerable, Func select) { foreach (T t in enumerable) { yield return select(t); } } // Name needs to be different so it doesn't conflict with Enumerable.Select internal static U[] Map (this ICollection collection, Func select) { int count = collection.Count; U[] result = new U[count]; count = 0; foreach (T t in collection) { result[count++] = select(t); } return result; } internal static IEnumerable Where (this IEnumerable enumerable, Func where) { foreach (T t in enumerable) { if (where(t)) { yield return t; } } } internal static bool Any (this IEnumerable source, Func predicate) { foreach (T element in source) { if (predicate(element)) { return true; } } return false; } internal static bool All (this IEnumerable source, Func predicate) { foreach (T element in source) { if (!predicate(element)) { return false; } } return true; } internal static T[] RemoveFirst (this T[] array) { T[] result = new T[array.Length - 1]; Array.Copy(array, 1, result, 0, result.Length); return result; } internal static T[] RemoveLast (this T[] array) { T[] result = new T[array.Length - 1]; Array.Copy(array, 0, result, 0, result.Length); return result; } internal static T[] AddFirst (this IList list, T item) { T[] res = new T[list.Count + 1]; res[0] = item; list.CopyTo(res, 1); return res; } internal static T[] AddLast (this IList list, T item) { T[] res = new T[list.Count + 1]; list.CopyTo(res, 0); res[list.Count] = item; return res; } internal static T First (this IEnumerable source) { var list = source as IList ; if (list != null) { return list[0]; } using (var e = source.GetEnumerator()) { if (e.MoveNext()) return e.Current; } throw new InvalidOperationException(); } internal static T Last (this IList list) { return list[list.Count - 1]; } internal static T[] Copy (this T[] array) { T[] copy = new T[array.Length]; Array.Copy(array, copy, array.Length); return copy; } } internal static class EmptyReadOnlyCollection { internal static ReadOnlyCollection Instance = new TrueReadOnlyCollection (new T[0]); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; #if !MICROSOFT_SCRIPTING_CORE using System.Diagnostics.Contracts; #endif using System.Runtime.CompilerServices; namespace System.Dynamic.Utils { internal static class CollectionExtensions { /// /// Wraps the provided enumerable into a ReadOnlyCollection{T} /// /// Copies all of the data into a new array, so the data can't be /// changed after creation. The exception is if the enumerable is /// already a ReadOnlyCollection{T}, in which case we just return it. /// #if !MICROSOFT_SCRIPTING_CORE [Pure] #endif internal static ReadOnlyCollectionToReadOnly (this IEnumerable enumerable) { if (enumerable == null) { return EmptyReadOnlyCollection .Instance; } var troc = enumerable as TrueReadOnlyCollection ; if (troc != null) { return troc; } var builder = enumerable as ReadOnlyCollectionBuilder ; if (builder != null) { return builder.ToReadOnlyCollection(); } var collection = enumerable as ICollection ; if (collection != null) { int count = collection.Count; if (count == 0) { return EmptyReadOnlyCollection .Instance; } T[] clone = new T[count]; collection.CopyTo(clone, 0); return new TrueReadOnlyCollection (clone); } // ToArray trims the excess space and speeds up access return new TrueReadOnlyCollection (new List (enumerable).ToArray()); } // We could probably improve the hashing here internal static int ListHashCode (this IEnumerable list) { var cmp = EqualityComparer .Default; int h = 6551; foreach (T t in list) { h ^= (h << 5) ^ cmp.GetHashCode(t); } return h; } #if !MICROSOFT_SCRIPTING_CORE [Pure] #endif internal static bool ListEquals (this ICollection first, ICollection second) { if (first.Count != second.Count) { return false; } var cmp = EqualityComparer .Default; var f = first.GetEnumerator(); var s = second.GetEnumerator(); while (f.MoveNext()) { s.MoveNext(); if (!cmp.Equals(f.Current, s.Current)) { return false; } } return true; } internal static IEnumerable Select (this IEnumerable enumerable, Func select) { foreach (T t in enumerable) { yield return select(t); } } // Name needs to be different so it doesn't conflict with Enumerable.Select internal static U[] Map (this ICollection collection, Func select) { int count = collection.Count; U[] result = new U[count]; count = 0; foreach (T t in collection) { result[count++] = select(t); } return result; } internal static IEnumerable Where (this IEnumerable enumerable, Func where) { foreach (T t in enumerable) { if (where(t)) { yield return t; } } } internal static bool Any (this IEnumerable source, Func predicate) { foreach (T element in source) { if (predicate(element)) { return true; } } return false; } internal static bool All (this IEnumerable source, Func predicate) { foreach (T element in source) { if (!predicate(element)) { return false; } } return true; } internal static T[] RemoveFirst (this T[] array) { T[] result = new T[array.Length - 1]; Array.Copy(array, 1, result, 0, result.Length); return result; } internal static T[] RemoveLast (this T[] array) { T[] result = new T[array.Length - 1]; Array.Copy(array, 0, result, 0, result.Length); return result; } internal static T[] AddFirst (this IList list, T item) { T[] res = new T[list.Count + 1]; res[0] = item; list.CopyTo(res, 1); return res; } internal static T[] AddLast (this IList list, T item) { T[] res = new T[list.Count + 1]; list.CopyTo(res, 0); res[list.Count] = item; return res; } internal static T First (this IEnumerable source) { var list = source as IList ; if (list != null) { return list[0]; } using (var e = source.GetEnumerator()) { if (e.MoveNext()) return e.Current; } throw new InvalidOperationException(); } internal static T Last (this IList list) { return list[list.Count - 1]; } internal static T[] Copy (this T[] array) { T[] copy = new T[array.Length]; Array.Copy(array, copy, array.Length); return copy; } } internal static class EmptyReadOnlyCollection { internal static ReadOnlyCollection Instance = new TrueReadOnlyCollection (new T[0]); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
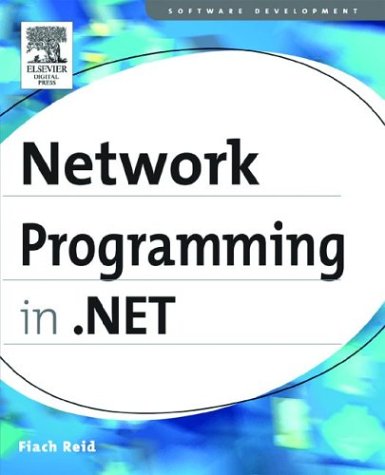
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProofTokenCryptoHandle.cs
- MemberJoinTreeNode.cs
- TrackingMemoryStreamFactory.cs
- PageStatePersister.cs
- XsdDataContractExporter.cs
- HttpPostServerProtocol.cs
- FrameworkContentElement.cs
- ClassImporter.cs
- SqlFacetAttribute.cs
- ToolStripTextBox.cs
- CodeCompileUnit.cs
- ContainerFilterService.cs
- latinshape.cs
- _ChunkParse.cs
- RtfControlWordInfo.cs
- Command.cs
- DebuggerAttributes.cs
- Input.cs
- CompletedAsyncResult.cs
- DefaultMemberAttribute.cs
- PageMediaType.cs
- DataServiceContext.cs
- ObjectDataSourceDisposingEventArgs.cs
- MexHttpsBindingElement.cs
- IODescriptionAttribute.cs
- TypeSystem.cs
- XmlConverter.cs
- FtpWebResponse.cs
- InternalDuplexChannelListener.cs
- SQLRoleProvider.cs
- CustomAttributeFormatException.cs
- MessagingDescriptionAttribute.cs
- CLRBindingWorker.cs
- SqlBooleanizer.cs
- ImageIndexConverter.cs
- LayoutInformation.cs
- OleAutBinder.cs
- HtmlInputText.cs
- ProfileGroupSettings.cs
- ProviderSettingsCollection.cs
- CopyAttributesAction.cs
- TouchEventArgs.cs
- ScaleTransform3D.cs
- ScrollProperties.cs
- OleDbConnectionInternal.cs
- InvalidCommandTreeException.cs
- ServicePerformanceCounters.cs
- WebDisplayNameAttribute.cs
- DeferredElementTreeState.cs
- BaseUriHelper.cs
- PointCollectionConverter.cs
- RuntimeHelpers.cs
- FlowDocumentReader.cs
- WorkflowTransactionService.cs
- DataGridSortCommandEventArgs.cs
- DbParameterHelper.cs
- NamedPipeDuplicateContext.cs
- AbstractExpressions.cs
- SoapEnumAttribute.cs
- NGCSerializer.cs
- ObjectListDesigner.cs
- NameValuePair.cs
- FaultCode.cs
- CodeCommentStatementCollection.cs
- DesignerEventService.cs
- DbParameterCollection.cs
- RuleDefinitions.cs
- URI.cs
- MenuItemStyleCollection.cs
- SelectorItemAutomationPeer.cs
- HTTP_SERVICE_CONFIG_URLACL_PARAM.cs
- ControlParser.cs
- SortedList.cs
- ICspAsymmetricAlgorithm.cs
- FormViewDeletedEventArgs.cs
- UserControlCodeDomTreeGenerator.cs
- ClrProviderManifest.cs
- MarkupExtensionReturnTypeAttribute.cs
- RetrieveVirtualItemEventArgs.cs
- BindingGroup.cs
- documentsequencetextcontainer.cs
- StylusButtonCollection.cs
- SQLMoney.cs
- ColorContext.cs
- CommonXSendMessage.cs
- ResourceDescriptionAttribute.cs
- SplineKeyFrames.cs
- NumericUpDownAcceleration.cs
- codemethodreferenceexpression.cs
- BCLDebug.cs
- Point3DCollectionValueSerializer.cs
- PathParser.cs
- TdsValueSetter.cs
- HttpResponseHeader.cs
- DataGridViewColumnCollectionEditor.cs
- DebugView.cs
- FormsAuthenticationUserCollection.cs
- ConfigXmlComment.cs
- XmlSchemaSequence.cs
- ArgIterator.cs