Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Utils / CacheDict.cs / 1305376 / CacheDict.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; namespace System.Dynamic.Utils { ////// Provides a dictionary-like object used for caches which holds onto a maximum /// number of elements specified at construction time. /// /// This class is not thread safe. /// internal class CacheDict{ private readonly Dictionary _dict = new Dictionary (); private readonly LinkedList _list = new LinkedList (); private readonly int _maxSize; /// /// Creates a dictionary-like object used for caches. /// /// The maximum number of elements to store. internal CacheDict(int maxSize) { _maxSize = maxSize; } ////// Tries to get the value associated with 'key', returning true if it's found and /// false if it's not present. /// internal bool TryGetValue(TKey key, out TValue value) { KeyInfo storedValue; if (_dict.TryGetValue(key, out storedValue)) { LinkedListNodenode = storedValue.List; if (node.Previous != null) { // move us to the head of the list... _list.Remove(node); _list.AddFirst(node); } value = storedValue.Value; return true; } value = default(TValue); return false; } /// /// Adds a new element to the cache, replacing and moving it to the front if the /// element is already present. /// internal void Add(TKey key, TValue value) { KeyInfo keyInfo; if (_dict.TryGetValue(key, out keyInfo)) { // remove original entry from the linked list _list.Remove(keyInfo.List); } else if (_list.Count == _maxSize) { // we've reached capacity, remove the last used element... LinkedListNodenode = _list.Last; _list.RemoveLast(); bool res = _dict.Remove(node.Value); Debug.Assert(res); } // add the new entry to the head of the list and into the dictionary LinkedListNode listNode = new LinkedListNode (key); _list.AddFirst(listNode); _dict[key] = new CacheDict .KeyInfo(value, listNode); } /// /// Returns the value associated with the given key, or throws KeyNotFoundException /// if the key is not present. /// internal TValue this[TKey key] { get { TValue res; if (TryGetValue(key, out res)) { return res; } throw new KeyNotFoundException(); } set { Add(key, value); } } private struct KeyInfo { internal readonly TValue Value; internal readonly LinkedListNodeList; internal KeyInfo(TValue value, LinkedListNode list) { Value = value; List = list; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; namespace System.Dynamic.Utils { /// /// Provides a dictionary-like object used for caches which holds onto a maximum /// number of elements specified at construction time. /// /// This class is not thread safe. /// internal class CacheDict{ private readonly Dictionary _dict = new Dictionary (); private readonly LinkedList _list = new LinkedList (); private readonly int _maxSize; /// /// Creates a dictionary-like object used for caches. /// /// The maximum number of elements to store. internal CacheDict(int maxSize) { _maxSize = maxSize; } ////// Tries to get the value associated with 'key', returning true if it's found and /// false if it's not present. /// internal bool TryGetValue(TKey key, out TValue value) { KeyInfo storedValue; if (_dict.TryGetValue(key, out storedValue)) { LinkedListNodenode = storedValue.List; if (node.Previous != null) { // move us to the head of the list... _list.Remove(node); _list.AddFirst(node); } value = storedValue.Value; return true; } value = default(TValue); return false; } /// /// Adds a new element to the cache, replacing and moving it to the front if the /// element is already present. /// internal void Add(TKey key, TValue value) { KeyInfo keyInfo; if (_dict.TryGetValue(key, out keyInfo)) { // remove original entry from the linked list _list.Remove(keyInfo.List); } else if (_list.Count == _maxSize) { // we've reached capacity, remove the last used element... LinkedListNodenode = _list.Last; _list.RemoveLast(); bool res = _dict.Remove(node.Value); Debug.Assert(res); } // add the new entry to the head of the list and into the dictionary LinkedListNode listNode = new LinkedListNode (key); _list.AddFirst(listNode); _dict[key] = new CacheDict .KeyInfo(value, listNode); } /// /// Returns the value associated with the given key, or throws KeyNotFoundException /// if the key is not present. /// internal TValue this[TKey key] { get { TValue res; if (TryGetValue(key, out res)) { return res; } throw new KeyNotFoundException(); } set { Add(key, value); } } private struct KeyInfo { internal readonly TValue Value; internal readonly LinkedListNodeList; internal KeyInfo(TValue value, LinkedListNode list) { Value = value; List = list; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
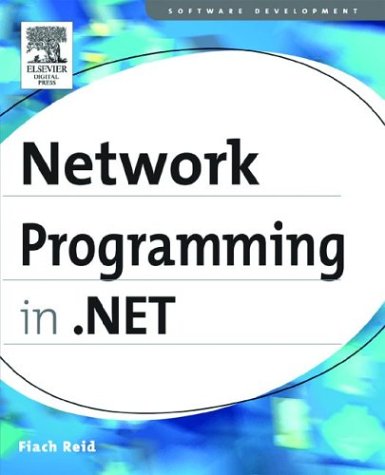
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmiConnection.cs
- File.cs
- InstanceDataCollectionCollection.cs
- IdentifierCollection.cs
- BindingNavigator.cs
- GridViewRowCollection.cs
- ProgressBarAutomationPeer.cs
- ProcessHostServerConfig.cs
- NameTable.cs
- OSEnvironmentHelper.cs
- RadioButton.cs
- CompiledELinqQueryState.cs
- WindowsHyperlink.cs
- ValidatorCompatibilityHelper.cs
- XmlChildEnumerator.cs
- UiaCoreApi.cs
- ComponentRenameEvent.cs
- RectangleHotSpot.cs
- ScrollProviderWrapper.cs
- SqlCacheDependency.cs
- DesignSurfaceCollection.cs
- PreviewPageInfo.cs
- SqlUnionizer.cs
- SchemaTypeEmitter.cs
- TiffBitmapEncoder.cs
- BitmapEffectRenderDataResource.cs
- IsolatedStorage.cs
- XmlSchemaChoice.cs
- Group.cs
- AuthStoreRoleProvider.cs
- SrgsElementFactoryCompiler.cs
- TagNameToTypeMapper.cs
- InvokeHandlers.cs
- SecurityCriticalDataForSet.cs
- SendMessageContent.cs
- PeerChannelFactory.cs
- SqlTrackingQuery.cs
- Label.cs
- UnsafeNativeMethods.cs
- ToggleButton.cs
- CacheEntry.cs
- SchemaCollectionPreprocessor.cs
- WindowsRichEditRange.cs
- HasCopySemanticsAttribute.cs
- PublisherIdentityPermission.cs
- webclient.cs
- EventLog.cs
- DynamicRendererThreadManager.cs
- ModelItemDictionary.cs
- GridViewPageEventArgs.cs
- DataGridViewCellCancelEventArgs.cs
- PinnedBufferMemoryStream.cs
- CodeGenerator.cs
- MetaData.cs
- CfgParser.cs
- FrameworkTemplate.cs
- AmbientValueAttribute.cs
- CommunicationObjectAbortedException.cs
- _ChunkParse.cs
- RootNamespaceAttribute.cs
- HtmlShimManager.cs
- DataTableTypeConverter.cs
- ChannelAcceptor.cs
- ColorMatrix.cs
- DBDataPermissionAttribute.cs
- AppSettingsExpressionBuilder.cs
- DoubleUtil.cs
- EditBehavior.cs
- BuildProviderCollection.cs
- iisPickupDirectory.cs
- ScriptReferenceBase.cs
- SmiRecordBuffer.cs
- DateTimeFormatInfoScanner.cs
- HtmlMeta.cs
- ConfigurationConverterBase.cs
- PropertyValueChangedEvent.cs
- CallTemplateAction.cs
- DataGridViewCellCollection.cs
- DetailsViewDeletedEventArgs.cs
- EncoderParameters.cs
- SmtpNetworkElement.cs
- NoResizeHandleGlyph.cs
- ContextMarshalException.cs
- DataGridViewCellCollection.cs
- SchemaMapping.cs
- SynchronizationLockException.cs
- WebPartDeleteVerb.cs
- DPAPIProtectedConfigurationProvider.cs
- PathFigure.cs
- SafeNativeMethods.cs
- SecurityPermission.cs
- DropAnimation.xaml.cs
- ConsoleTraceListener.cs
- PolicyManager.cs
- SEHException.cs
- FilteredReadOnlyMetadataCollection.cs
- CounterSample.cs
- PasswordDeriveBytes.cs
- EntityDataSourceDesigner.cs
- RepeatBehavior.cs