Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / NestedContainer.cs / 1305376 / NestedContainer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel; using System.Globalization; using System.IO; using System.Security.Permissions; ////// A nested container is a container that is owned by another component. Nested /// containers can be found by querying a component site's services for NestedConainter. /// Nested containers are a useful tool to establish owner relationships among components. /// All components within a nested container are named with the owning component's name /// as a prefix. /// [HostProtection(SharedState = true)] public class NestedContainer : Container, INestedContainer { private IComponent _owner; ////// Creates a new NestedContainer. /// public NestedContainer(IComponent owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; _owner.Disposed += new EventHandler(OnOwnerDisposed); } ////// The component that owns this nested container. /// public IComponent Owner { get { return _owner; } } ////// Retrieves the name of the owning component. This may be overridden to /// provide a custom owner name. The default searches the owner's site for /// INestedSite and calls FullName, or ISite.Name if there is no nested site. /// If neither is available, this returns null. /// protected virtual string OwnerName { get { string ownerName = null; if (_owner != null && _owner.Site != null) { INestedSite nestedOwnerSite = _owner.Site as INestedSite; if (nestedOwnerSite != null) { ownerName = nestedOwnerSite.FullName; } else { ownerName = _owner.Site.Name; } } return ownerName; } } ////// Creates a site for the component within the container. /// protected override ISite CreateSite(IComponent component, string name) { if (component == null) { throw new ArgumentNullException("component"); } return new Site(component, this, name); } ////// Override of Container's dispose. /// protected override void Dispose(bool disposing) { if (disposing) { _owner.Disposed -= new EventHandler(OnOwnerDisposed); } base.Dispose(disposing); } ////// protected override object GetService(Type service) { if (service == typeof(INestedContainer)) { return this; } else { return base.GetService(service); } } ///[To be supplied.] ////// Called when our owning component is destroyed. /// private void OnOwnerDisposed(object sender, EventArgs e) { Dispose(); } ////// Simple site implementation. We do some special processing to name the site, but /// that's about it. /// private class Site : INestedSite { private IComponent component; private NestedContainer container; private string name; internal Site(IComponent component, NestedContainer container, string name) { this.component = component; this.container = container; this.name = name; } // The component sited by this component site. public IComponent Component { get { return component; } } // The container in which the component is sited. public IContainer Container { get { return container; } } public Object GetService(Type service) { return((service == typeof(ISite)) ? this : container.GetService(service)); } // Indicates whether the component is in design mode. public bool DesignMode { get { IComponent owner = container.Owner; if (owner != null && owner.Site != null) { return owner.Site.DesignMode; } return false; } } public string FullName { get { if (name != null) { string ownerName = container.OwnerName; string childName = name; if (ownerName != null) { childName = string.Format(CultureInfo.InvariantCulture, "{0}.{1}", ownerName, childName); } return childName; } return name; } } // The name of the component. // public String Name { get { return name; } set { if (value == null || name == null || !value.Equals(name)) { container.ValidateName(component, value); name = value; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel; using System.Globalization; using System.IO; using System.Security.Permissions; ////// A nested container is a container that is owned by another component. Nested /// containers can be found by querying a component site's services for NestedConainter. /// Nested containers are a useful tool to establish owner relationships among components. /// All components within a nested container are named with the owning component's name /// as a prefix. /// [HostProtection(SharedState = true)] public class NestedContainer : Container, INestedContainer { private IComponent _owner; ////// Creates a new NestedContainer. /// public NestedContainer(IComponent owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; _owner.Disposed += new EventHandler(OnOwnerDisposed); } ////// The component that owns this nested container. /// public IComponent Owner { get { return _owner; } } ////// Retrieves the name of the owning component. This may be overridden to /// provide a custom owner name. The default searches the owner's site for /// INestedSite and calls FullName, or ISite.Name if there is no nested site. /// If neither is available, this returns null. /// protected virtual string OwnerName { get { string ownerName = null; if (_owner != null && _owner.Site != null) { INestedSite nestedOwnerSite = _owner.Site as INestedSite; if (nestedOwnerSite != null) { ownerName = nestedOwnerSite.FullName; } else { ownerName = _owner.Site.Name; } } return ownerName; } } ////// Creates a site for the component within the container. /// protected override ISite CreateSite(IComponent component, string name) { if (component == null) { throw new ArgumentNullException("component"); } return new Site(component, this, name); } ////// Override of Container's dispose. /// protected override void Dispose(bool disposing) { if (disposing) { _owner.Disposed -= new EventHandler(OnOwnerDisposed); } base.Dispose(disposing); } ////// protected override object GetService(Type service) { if (service == typeof(INestedContainer)) { return this; } else { return base.GetService(service); } } ///[To be supplied.] ////// Called when our owning component is destroyed. /// private void OnOwnerDisposed(object sender, EventArgs e) { Dispose(); } ////// Simple site implementation. We do some special processing to name the site, but /// that's about it. /// private class Site : INestedSite { private IComponent component; private NestedContainer container; private string name; internal Site(IComponent component, NestedContainer container, string name) { this.component = component; this.container = container; this.name = name; } // The component sited by this component site. public IComponent Component { get { return component; } } // The container in which the component is sited. public IContainer Container { get { return container; } } public Object GetService(Type service) { return((service == typeof(ISite)) ? this : container.GetService(service)); } // Indicates whether the component is in design mode. public bool DesignMode { get { IComponent owner = container.Owner; if (owner != null && owner.Site != null) { return owner.Site.DesignMode; } return false; } } public string FullName { get { if (name != null) { string ownerName = container.OwnerName; string childName = name; if (ownerName != null) { childName = string.Format(CultureInfo.InvariantCulture, "{0}.{1}", ownerName, childName); } return childName; } return name; } } // The name of the component. // public String Name { get { return name; } set { if (value == null || name == null || !value.Equals(name)) { container.ValidateName(component, value); name = value; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
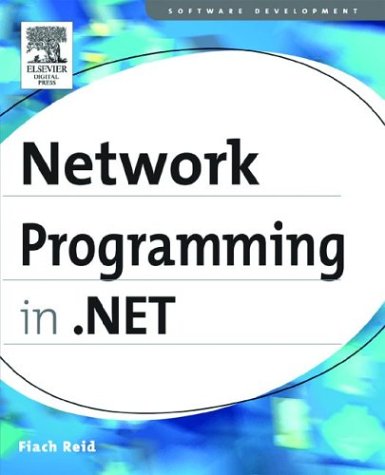
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CacheHelper.cs
- Signature.cs
- SafeHandles.cs
- SmtpAuthenticationManager.cs
- Sentence.cs
- XmlCharType.cs
- Page.cs
- TableParaClient.cs
- XmlBinaryReaderSession.cs
- AdvancedBindingPropertyDescriptor.cs
- SelectedGridItemChangedEvent.cs
- TracingConnectionInitiator.cs
- HttpModuleActionCollection.cs
- ContentElement.cs
- ByteStorage.cs
- ColorMatrix.cs
- GlyphsSerializer.cs
- EditorPartCollection.cs
- SpeechRecognitionEngine.cs
- ContourSegment.cs
- XmlUtil.cs
- SvcMapFile.cs
- WindowsAuthenticationEventArgs.cs
- SQLDecimal.cs
- ConditionalAttribute.cs
- Exceptions.cs
- HttpHandlersSection.cs
- PlacementWorkspace.cs
- DiagnosticsConfigurationHandler.cs
- HttpWriter.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- SimpleFieldTemplateUserControl.cs
- DefaultAsyncDataDispatcher.cs
- MethodRental.cs
- PropertyChangedEventArgs.cs
- HtmlShimManager.cs
- CoreSwitches.cs
- FontSizeConverter.cs
- UntrustedRecipientException.cs
- TextWriterTraceListener.cs
- DashStyle.cs
- StaticExtension.cs
- GlyphingCache.cs
- SystemIPAddressInformation.cs
- AddressHeader.cs
- CompilerWrapper.cs
- ToolStripItemCollection.cs
- ListControl.cs
- DataErrorValidationRule.cs
- Listbox.cs
- Encoder.cs
- RealProxy.cs
- PointCollection.cs
- VideoDrawing.cs
- WindowsFormsSectionHandler.cs
- DeviceContext2.cs
- QilGenerator.cs
- StringReader.cs
- ModelItemDictionaryImpl.cs
- DataGridLinkButton.cs
- LineServicesCallbacks.cs
- DbProviderFactories.cs
- Number.cs
- Size.cs
- ThicknessKeyFrameCollection.cs
- KeyboardEventArgs.cs
- PackWebResponse.cs
- SmtpClient.cs
- EntityCommandExecutionException.cs
- SafeReversePInvokeHandle.cs
- EntryIndex.cs
- WebPartHelpVerb.cs
- ADMembershipUser.cs
- OptimizedTemplateContent.cs
- ExtentCqlBlock.cs
- TableProviderWrapper.cs
- HttpFormatExtensions.cs
- PerformanceCounterNameAttribute.cs
- CodeTypeConstructor.cs
- BindMarkupExtensionSerializer.cs
- HighlightComponent.cs
- DropShadowEffect.cs
- XmlSchemaExporter.cs
- ScrollableControl.cs
- CompareValidator.cs
- DoubleKeyFrameCollection.cs
- SoapSchemaMember.cs
- ToolStripItemRenderEventArgs.cs
- ClientApiGenerator.cs
- ControlEvent.cs
- HttpAsyncResult.cs
- StandardCommands.cs
- WebPartEditorCancelVerb.cs
- OrderedParallelQuery.cs
- SmtpSection.cs
- HandleExceptionArgs.cs
- mediapermission.cs
- InvalidCommandTreeException.cs
- DataGridViewDataErrorEventArgs.cs
- ApplicationException.cs