Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / LinearGradientBrush.cs / 1305376 / LinearGradientBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; using System.Runtime.InteropServices; using System.Drawing; using System.Drawing.Internal; using System.Runtime.Versioning; /** * Represent a LinearGradient brush object */ ////// /// public sealed class LinearGradientBrush : Brush { private bool interpolationColorsWasSet; /** * Create a new rectangle gradient brush object */ ////// Encapsulates a ///with a linear gradient. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(PointF point1, PointF point2, Color color1, Color color2) { IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateLineBrush(new GPPOINTF(point1), new GPPOINTF(point2), color1.ToArgb(), color2.ToArgb(), (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ///class with the specified points and /// colors. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Point point1, Point point2, Color color1, Color color2) { IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateLineBrushI(new GPPOINT(point1), new GPPOINT(point2), color1.ToArgb(), color2.ToArgb(), (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Initializes a new instance of the ///class with the /// specified points and colors. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(RectangleF rect, Color color1, Color color2, LinearGradientMode linearGradientMode) { //validate the LinearGradientMode enum //valid values are 0x0 to 0x3 if (!ClientUtils.IsEnumValid(linearGradientMode, (int)linearGradientMode, (int)LinearGradientMode.Horizontal, (int)LinearGradientMode.BackwardDiagonal)){ throw new InvalidEnumArgumentException("linearGradientMode", (int)linearGradientMode, typeof(LinearGradientMode)); } //validate the rect if (rect.Width == 0.0 || rect.Height == 0.0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } IntPtr brush = IntPtr.Zero; GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRect(ref gprectf, color1.ToArgb(), color2.ToArgb(), (int) linearGradientMode, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with /// the specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Rectangle rect, Color color1, Color color2, LinearGradientMode linearGradientMode) { //validate the LinearGradientMode enum //valid values are 0x0 to 0x3 if (!ClientUtils.IsEnumValid(linearGradientMode, (int)linearGradientMode, (int)LinearGradientMode.Horizontal, (int)LinearGradientMode.BackwardDiagonal)) { throw new InvalidEnumArgumentException("linearGradientMode", (int)linearGradientMode, typeof(LinearGradientMode)); } //validate the rect if (rect.Width == 0 || rect.Height == 0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } IntPtr brush = IntPtr.Zero; GPRECT gpRect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRectI(ref gpRect, color1.ToArgb(), color2.ToArgb(), (int) linearGradientMode, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(RectangleF rect, Color color1, Color color2, float angle) : this(rect, color1, color2, angle, false) {} ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(RectangleF rect, Color color1, Color color2, float angle, bool isAngleScaleable) { IntPtr brush = IntPtr.Zero; //validate the rect if (rect.Width == 0.0 || rect.Height == 0.0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRectWithAngle(ref gprectf, color1.ToArgb(), color2.ToArgb(), angle, isAngleScaleable, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Rectangle rect, Color color1, Color color2, float angle) : this(rect, color1, color2, angle, false) { } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Rectangle rect, Color color1, Color color2, float angle, bool isAngleScaleable) { IntPtr brush = IntPtr.Zero; //validate the rect if (rect.Width == 0 || rect.Height == 0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRectWithAngleI(ref gprect, color1.ToArgb(), color2.ToArgb(), angle, isAngleScaleable, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// Constructor to initialized this object to be owned by GDI+. /// internal LinearGradientBrush(IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrushInternal( nativeBrush ); } ////// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new LinearGradientBrush(cloneBrush); } /** * Get/set colors */ private void _SetLinearColors(Color color1, Color color2) { int status = SafeNativeMethods.Gdip.GdipSetLineColors(new HandleRef(this, this.NativeBrush), color1.ToArgb(), color2.ToArgb()); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private Color[] _GetLinearColors() { int[] colors = new int[] { 0, 0 }; int status = SafeNativeMethods.Gdip.GdipGetLineColors(new HandleRef(this, this.NativeBrush), colors); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); Color[] lineColor = new Color[2]; lineColor[0] = Color.FromArgb(colors[0]); lineColor[1] = Color.FromArgb(colors[1]); return lineColor; } ///. /// /// /// Gets or sets the starting and ending colors of the /// gradient. /// public Color[] LinearColors { get { return _GetLinearColors();} set { _SetLinearColors(value[0], value[1]);} } /** * Get source rectangle */ private RectangleF _GetRectangle() { GPRECTF rect = new GPRECTF(); int status = SafeNativeMethods.Gdip.GdipGetLineRect(new HandleRef(this, this.NativeBrush), ref rect); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return rect.ToRectangleF(); } ////// /// public RectangleF Rectangle { get { return _GetRectangle(); } } ////// Gets a rectangular region that defines the /// starting and ending points of the gradient. /// ////// /// public bool GammaCorrection { get { bool useGammaCorrection; int status = SafeNativeMethods.Gdip.GdipGetLineGammaCorrection(new HandleRef(this, this.NativeBrush), out useGammaCorrection); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return useGammaCorrection; } set { int status = SafeNativeMethods.Gdip.GdipSetLineGammaCorrection(new HandleRef(this, this.NativeBrush), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } /** * Get/set blend factors * * @notes If the blendFactors.Length = 1, then it's treated * as the falloff parameter. Otherwise, it's the array * of blend factors. */ private Blend _GetBlend() { // VSWHidbey 518309 - interpolation colors and blends don't get along. Just getting // the Blend when InterpolationColors was set puts the Brush into an unusable state afterwards. // so to avoid that (mostly the problem of having Blend pop up in the debugger window and cause this problem) // we just bail here. // if (interpolationColorsWasSet) { return null; } Blend blend; // Figure out the size of blend factor array int retval = 0; int status = SafeNativeMethods.Gdip.GdipGetLineBlendCount(new HandleRef(this, this.NativeBrush), out retval); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } if (retval <= 0) { return null; } // Allocate temporary native memory buffer int count = retval; IntPtr factors = IntPtr.Zero; IntPtr positions = IntPtr.Zero; try { factors = Marshal.AllocHGlobal(4*count); positions = Marshal.AllocHGlobal(4*count); // Retrieve horizontal blend factors status = SafeNativeMethods.Gdip.GdipGetLineBlend(new HandleRef(this, this.NativeBrush), factors, positions, count); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } // Return the result in a managed array blend = new Blend(count); Marshal.Copy(factors, blend.Factors, 0, count); Marshal.Copy(positions, blend.Positions, 0, count); } finally { if( factors != IntPtr.Zero ){ Marshal.FreeHGlobal(factors); } if( positions != IntPtr.Zero ){ Marshal.FreeHGlobal(positions); } } return blend; } private void _SetBlend(Blend blend) { // Allocate temporary native memory buffer // and copy input blend factors into it. int count = blend.Factors.Length; IntPtr factors = IntPtr.Zero; IntPtr positions = IntPtr.Zero; try { factors = Marshal.AllocHGlobal(4*count); positions = Marshal.AllocHGlobal(4*count); Marshal.Copy(blend.Factors, 0, factors, count); Marshal.Copy(blend.Positions, 0, positions, count); // Set blend factors int status = SafeNativeMethods.Gdip.GdipSetLineBlend(new HandleRef(this, this.NativeBrush), new HandleRef(null, factors), new HandleRef(null, positions), count); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } finally{ if( factors != IntPtr.Zero ) { Marshal.FreeHGlobal(factors); } if( positions != IntPtr.Zero ) { Marshal.FreeHGlobal(positions); } } } ////// Gets or sets a value indicating whether /// gamma correction is enabled for this ///. /// /// /// Gets or sets a public Blend Blend { get { return _GetBlend();} set { _SetBlend(value);} } /* * SigmaBlend & LinearBlend not yet implemented */ ///that specifies /// positions and factors that define a custom falloff for the gradient. /// /// /// Creates a gradient falloff based on a /// bell-shaped curve. /// public void SetSigmaBellShape(float focus) { SetSigmaBellShape(focus, (float)1.0); } ////// /// Creates a gradient falloff based on a /// bell-shaped curve. /// public void SetSigmaBellShape(float focus, float scale) { int status = SafeNativeMethods.Gdip.GdipSetLineSigmaBlend(new HandleRef(this, this.NativeBrush), focus, scale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// /// public void SetBlendTriangularShape(float focus) { SetBlendTriangularShape(focus, (float)1.0); } ////// Creates a triangular gradient. /// ////// /// public void SetBlendTriangularShape(float focus, float scale) { int status = SafeNativeMethods.Gdip.GdipSetLineLinearBlend(new HandleRef(this, this.NativeBrush), focus, scale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } /* * Preset Color Blend */ private ColorBlend _GetInterpolationColors() { ColorBlend blend; if (!interpolationColorsWasSet) { throw new ArgumentException(SR.GetString(SR.InterpolationColorsCommon, SR.GetString(SR.InterpolationColorsColorBlendNotSet),"")); } // Figure out the size of blend factor array int retval = 0; int status = SafeNativeMethods.Gdip.GdipGetLinePresetBlendCount(new HandleRef(this, this.NativeBrush), out retval); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } // Allocate temporary native memory buffer int count = retval; IntPtr colors = IntPtr.Zero; IntPtr positions = IntPtr.Zero; try { colors = Marshal.AllocHGlobal(4*count); positions = Marshal.AllocHGlobal(4*count); // Retrieve horizontal blend factors status = SafeNativeMethods.Gdip.GdipGetLinePresetBlend(new HandleRef(this, this.NativeBrush), colors, positions, count); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } // Return the result in a managed array blend = new ColorBlend(count); int[] argb = new int[count]; Marshal.Copy(colors, argb, 0, count); Marshal.Copy(positions, blend.Positions, 0, count); // copy ARGB values into Color array of ColorBlend blend.Colors = new Color[argb.Length]; for (int i=0; i/// Creates a triangular gradient. /// ////// /// public ColorBlend InterpolationColors { get { return _GetInterpolationColors();} set { _SetInterpolationColors(value);} } /** * Set/get brush wrapping mode */ private void _SetWrapMode(WrapMode wrapMode) { int status = SafeNativeMethods.Gdip.GdipSetLineWrapMode(new HandleRef(this, this.NativeBrush), (int) wrapMode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private WrapMode _GetWrapMode() { int mode = 0; int status = SafeNativeMethods.Gdip.GdipGetLineWrapMode(new HandleRef(this, this.NativeBrush), out mode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return (WrapMode) mode; } ////// Gets or sets a ///that defines a multi-color linear /// gradient. /// /// /// public WrapMode WrapMode { get { return _GetWrapMode(); } set { //validate the WrapMode enum //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(value, (int)value, (int)WrapMode.Tile, (int)WrapMode.Clamp)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(WrapMode)); } _SetWrapMode(value); } } /** * Set/get brush transform */ private void _SetTransform(Matrix matrix) { if (matrix == null) throw new ArgumentNullException("matrix"); int status = SafeNativeMethods.Gdip.GdipSetLineTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private Matrix _GetTransform() { Matrix matrix = new Matrix(); // NOTE: new Matrix() will throw an exception if matrix == null. int status = SafeNativeMethods.Gdip.GdipGetLineTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return matrix; } ////// Gets or sets a ///that indicates the wrap mode for this . /// /// /// public Matrix Transform { get { return _GetTransform();} set { _SetTransform(value);} } ////// Gets or sets a ///that defines a local geometrical transform for /// this . /// /// /// Resets the public void ResetTransform() { int status = SafeNativeMethods.Gdip.GdipResetLineTransform(new HandleRef(this, this.NativeBrush)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///property to identity. /// /// /// public void MultiplyTransform(Matrix matrix) { MultiplyTransform(matrix, MatrixOrder.Prepend); } ////// Multiplies the ///that represents the local geometrical /// transform of this by the specified by prepending the specified . /// /// /// public void MultiplyTransform(Matrix matrix, MatrixOrder order) { if (matrix == null) { throw new ArgumentNullException("matrix"); } int status = SafeNativeMethods.Gdip.GdipMultiplyLineTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix), order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Multiplies the ///that represents the local geometrical /// transform of this by the specified in the specified order. /// /// /// Translates the local geometrical transform /// by the specified dimmensions. This method prepends the translation to the /// transform. /// public void TranslateTransform(float dx, float dy) { TranslateTransform(dx, dy, MatrixOrder.Prepend); } ////// /// Translates the local geometrical transform /// by the specified dimmensions in the specified order. /// public void TranslateTransform(float dx, float dy, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipTranslateLineTransform(new HandleRef(this, this.NativeBrush), dx, dy, order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// /// Scales the local geometric transform by the /// specified amounts. This method prepends the scaling matrix to the transform. /// public void ScaleTransform(float sx, float sy) { ScaleTransform(sx, sy, MatrixOrder.Prepend); } ////// /// public void ScaleTransform(float sx, float sy, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipScaleLineTransform(new HandleRef(this, this.NativeBrush), sx, sy, order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Scales the local geometric transform by the /// specified amounts in the specified order. /// ////// /// Rotates the local geometric transform by the /// specified amount. This method prepends the rotation to the transform. /// public void RotateTransform(float angle) { RotateTransform(angle, MatrixOrder.Prepend); } ////// /// public void RotateTransform(float angle, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipRotateLineTransform(new HandleRef(this, this.NativeBrush), angle, order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Rotates the local geometric transform by the specified /// amount in the specified order. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; using System.Runtime.InteropServices; using System.Drawing; using System.Drawing.Internal; using System.Runtime.Versioning; /** * Represent a LinearGradient brush object */ ////// /// public sealed class LinearGradientBrush : Brush { private bool interpolationColorsWasSet; /** * Create a new rectangle gradient brush object */ ////// Encapsulates a ///with a linear gradient. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(PointF point1, PointF point2, Color color1, Color color2) { IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateLineBrush(new GPPOINTF(point1), new GPPOINTF(point2), color1.ToArgb(), color2.ToArgb(), (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ///class with the specified points and /// colors. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Point point1, Point point2, Color color1, Color color2) { IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateLineBrushI(new GPPOINT(point1), new GPPOINT(point2), color1.ToArgb(), color2.ToArgb(), (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Initializes a new instance of the ///class with the /// specified points and colors. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(RectangleF rect, Color color1, Color color2, LinearGradientMode linearGradientMode) { //validate the LinearGradientMode enum //valid values are 0x0 to 0x3 if (!ClientUtils.IsEnumValid(linearGradientMode, (int)linearGradientMode, (int)LinearGradientMode.Horizontal, (int)LinearGradientMode.BackwardDiagonal)){ throw new InvalidEnumArgumentException("linearGradientMode", (int)linearGradientMode, typeof(LinearGradientMode)); } //validate the rect if (rect.Width == 0.0 || rect.Height == 0.0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } IntPtr brush = IntPtr.Zero; GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRect(ref gprectf, color1.ToArgb(), color2.ToArgb(), (int) linearGradientMode, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with /// the specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Rectangle rect, Color color1, Color color2, LinearGradientMode linearGradientMode) { //validate the LinearGradientMode enum //valid values are 0x0 to 0x3 if (!ClientUtils.IsEnumValid(linearGradientMode, (int)linearGradientMode, (int)LinearGradientMode.Horizontal, (int)LinearGradientMode.BackwardDiagonal)) { throw new InvalidEnumArgumentException("linearGradientMode", (int)linearGradientMode, typeof(LinearGradientMode)); } //validate the rect if (rect.Width == 0 || rect.Height == 0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } IntPtr brush = IntPtr.Zero; GPRECT gpRect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRectI(ref gpRect, color1.ToArgb(), color2.ToArgb(), (int) linearGradientMode, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(RectangleF rect, Color color1, Color color2, float angle) : this(rect, color1, color2, angle, false) {} ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(RectangleF rect, Color color1, Color color2, float angle, bool isAngleScaleable) { IntPtr brush = IntPtr.Zero; //validate the rect if (rect.Width == 0.0 || rect.Height == 0.0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRectWithAngle(ref gprectf, color1.ToArgb(), color2.ToArgb(), angle, isAngleScaleable, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Rectangle rect, Color color1, Color color2, float angle) : this(rect, color1, color2, angle, false) { } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public LinearGradientBrush(Rectangle rect, Color color1, Color color2, float angle, bool isAngleScaleable) { IntPtr brush = IntPtr.Zero; //validate the rect if (rect.Width == 0 || rect.Height == 0) { throw new ArgumentException(SR.GetString(SR.GdiplusInvalidRectangle, rect.ToString())); } GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCreateLineBrushFromRectWithAngleI(ref gprect, color1.ToArgb(), color2.ToArgb(), angle, isAngleScaleable, (int)WrapMode.Tile, out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Encapsulates a new instance of the ///class with the /// specified points, colors, and orientation. /// /// Constructor to initialized this object to be owned by GDI+. /// internal LinearGradientBrush(IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrushInternal( nativeBrush ); } ////// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new LinearGradientBrush(cloneBrush); } /** * Get/set colors */ private void _SetLinearColors(Color color1, Color color2) { int status = SafeNativeMethods.Gdip.GdipSetLineColors(new HandleRef(this, this.NativeBrush), color1.ToArgb(), color2.ToArgb()); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private Color[] _GetLinearColors() { int[] colors = new int[] { 0, 0 }; int status = SafeNativeMethods.Gdip.GdipGetLineColors(new HandleRef(this, this.NativeBrush), colors); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); Color[] lineColor = new Color[2]; lineColor[0] = Color.FromArgb(colors[0]); lineColor[1] = Color.FromArgb(colors[1]); return lineColor; } ///. /// /// /// Gets or sets the starting and ending colors of the /// gradient. /// public Color[] LinearColors { get { return _GetLinearColors();} set { _SetLinearColors(value[0], value[1]);} } /** * Get source rectangle */ private RectangleF _GetRectangle() { GPRECTF rect = new GPRECTF(); int status = SafeNativeMethods.Gdip.GdipGetLineRect(new HandleRef(this, this.NativeBrush), ref rect); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return rect.ToRectangleF(); } ////// /// public RectangleF Rectangle { get { return _GetRectangle(); } } ////// Gets a rectangular region that defines the /// starting and ending points of the gradient. /// ////// /// public bool GammaCorrection { get { bool useGammaCorrection; int status = SafeNativeMethods.Gdip.GdipGetLineGammaCorrection(new HandleRef(this, this.NativeBrush), out useGammaCorrection); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return useGammaCorrection; } set { int status = SafeNativeMethods.Gdip.GdipSetLineGammaCorrection(new HandleRef(this, this.NativeBrush), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } /** * Get/set blend factors * * @notes If the blendFactors.Length = 1, then it's treated * as the falloff parameter. Otherwise, it's the array * of blend factors. */ private Blend _GetBlend() { // VSWHidbey 518309 - interpolation colors and blends don't get along. Just getting // the Blend when InterpolationColors was set puts the Brush into an unusable state afterwards. // so to avoid that (mostly the problem of having Blend pop up in the debugger window and cause this problem) // we just bail here. // if (interpolationColorsWasSet) { return null; } Blend blend; // Figure out the size of blend factor array int retval = 0; int status = SafeNativeMethods.Gdip.GdipGetLineBlendCount(new HandleRef(this, this.NativeBrush), out retval); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } if (retval <= 0) { return null; } // Allocate temporary native memory buffer int count = retval; IntPtr factors = IntPtr.Zero; IntPtr positions = IntPtr.Zero; try { factors = Marshal.AllocHGlobal(4*count); positions = Marshal.AllocHGlobal(4*count); // Retrieve horizontal blend factors status = SafeNativeMethods.Gdip.GdipGetLineBlend(new HandleRef(this, this.NativeBrush), factors, positions, count); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } // Return the result in a managed array blend = new Blend(count); Marshal.Copy(factors, blend.Factors, 0, count); Marshal.Copy(positions, blend.Positions, 0, count); } finally { if( factors != IntPtr.Zero ){ Marshal.FreeHGlobal(factors); } if( positions != IntPtr.Zero ){ Marshal.FreeHGlobal(positions); } } return blend; } private void _SetBlend(Blend blend) { // Allocate temporary native memory buffer // and copy input blend factors into it. int count = blend.Factors.Length; IntPtr factors = IntPtr.Zero; IntPtr positions = IntPtr.Zero; try { factors = Marshal.AllocHGlobal(4*count); positions = Marshal.AllocHGlobal(4*count); Marshal.Copy(blend.Factors, 0, factors, count); Marshal.Copy(blend.Positions, 0, positions, count); // Set blend factors int status = SafeNativeMethods.Gdip.GdipSetLineBlend(new HandleRef(this, this.NativeBrush), new HandleRef(null, factors), new HandleRef(null, positions), count); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } } finally{ if( factors != IntPtr.Zero ) { Marshal.FreeHGlobal(factors); } if( positions != IntPtr.Zero ) { Marshal.FreeHGlobal(positions); } } } ////// Gets or sets a value indicating whether /// gamma correction is enabled for this ///. /// /// /// Gets or sets a public Blend Blend { get { return _GetBlend();} set { _SetBlend(value);} } /* * SigmaBlend & LinearBlend not yet implemented */ ///that specifies /// positions and factors that define a custom falloff for the gradient. /// /// /// Creates a gradient falloff based on a /// bell-shaped curve. /// public void SetSigmaBellShape(float focus) { SetSigmaBellShape(focus, (float)1.0); } ////// /// Creates a gradient falloff based on a /// bell-shaped curve. /// public void SetSigmaBellShape(float focus, float scale) { int status = SafeNativeMethods.Gdip.GdipSetLineSigmaBlend(new HandleRef(this, this.NativeBrush), focus, scale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// /// public void SetBlendTriangularShape(float focus) { SetBlendTriangularShape(focus, (float)1.0); } ////// Creates a triangular gradient. /// ////// /// public void SetBlendTriangularShape(float focus, float scale) { int status = SafeNativeMethods.Gdip.GdipSetLineLinearBlend(new HandleRef(this, this.NativeBrush), focus, scale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } /* * Preset Color Blend */ private ColorBlend _GetInterpolationColors() { ColorBlend blend; if (!interpolationColorsWasSet) { throw new ArgumentException(SR.GetString(SR.InterpolationColorsCommon, SR.GetString(SR.InterpolationColorsColorBlendNotSet),"")); } // Figure out the size of blend factor array int retval = 0; int status = SafeNativeMethods.Gdip.GdipGetLinePresetBlendCount(new HandleRef(this, this.NativeBrush), out retval); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } // Allocate temporary native memory buffer int count = retval; IntPtr colors = IntPtr.Zero; IntPtr positions = IntPtr.Zero; try { colors = Marshal.AllocHGlobal(4*count); positions = Marshal.AllocHGlobal(4*count); // Retrieve horizontal blend factors status = SafeNativeMethods.Gdip.GdipGetLinePresetBlend(new HandleRef(this, this.NativeBrush), colors, positions, count); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } // Return the result in a managed array blend = new ColorBlend(count); int[] argb = new int[count]; Marshal.Copy(colors, argb, 0, count); Marshal.Copy(positions, blend.Positions, 0, count); // copy ARGB values into Color array of ColorBlend blend.Colors = new Color[argb.Length]; for (int i=0; i/// Creates a triangular gradient. /// ////// /// public ColorBlend InterpolationColors { get { return _GetInterpolationColors();} set { _SetInterpolationColors(value);} } /** * Set/get brush wrapping mode */ private void _SetWrapMode(WrapMode wrapMode) { int status = SafeNativeMethods.Gdip.GdipSetLineWrapMode(new HandleRef(this, this.NativeBrush), (int) wrapMode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private WrapMode _GetWrapMode() { int mode = 0; int status = SafeNativeMethods.Gdip.GdipGetLineWrapMode(new HandleRef(this, this.NativeBrush), out mode); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return (WrapMode) mode; } ////// Gets or sets a ///that defines a multi-color linear /// gradient. /// /// /// public WrapMode WrapMode { get { return _GetWrapMode(); } set { //validate the WrapMode enum //valid values are 0x0 to 0x4 if (!ClientUtils.IsEnumValid(value, (int)value, (int)WrapMode.Tile, (int)WrapMode.Clamp)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(WrapMode)); } _SetWrapMode(value); } } /** * Set/get brush transform */ private void _SetTransform(Matrix matrix) { if (matrix == null) throw new ArgumentNullException("matrix"); int status = SafeNativeMethods.Gdip.GdipSetLineTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private Matrix _GetTransform() { Matrix matrix = new Matrix(); // NOTE: new Matrix() will throw an exception if matrix == null. int status = SafeNativeMethods.Gdip.GdipGetLineTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return matrix; } ////// Gets or sets a ///that indicates the wrap mode for this . /// /// /// public Matrix Transform { get { return _GetTransform();} set { _SetTransform(value);} } ////// Gets or sets a ///that defines a local geometrical transform for /// this . /// /// /// Resets the public void ResetTransform() { int status = SafeNativeMethods.Gdip.GdipResetLineTransform(new HandleRef(this, this.NativeBrush)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///property to identity. /// /// /// public void MultiplyTransform(Matrix matrix) { MultiplyTransform(matrix, MatrixOrder.Prepend); } ////// Multiplies the ///that represents the local geometrical /// transform of this by the specified by prepending the specified . /// /// /// public void MultiplyTransform(Matrix matrix, MatrixOrder order) { if (matrix == null) { throw new ArgumentNullException("matrix"); } int status = SafeNativeMethods.Gdip.GdipMultiplyLineTransform(new HandleRef(this, this.NativeBrush), new HandleRef(matrix, matrix.nativeMatrix), order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Multiplies the ///that represents the local geometrical /// transform of this by the specified in the specified order. /// /// /// Translates the local geometrical transform /// by the specified dimmensions. This method prepends the translation to the /// transform. /// public void TranslateTransform(float dx, float dy) { TranslateTransform(dx, dy, MatrixOrder.Prepend); } ////// /// Translates the local geometrical transform /// by the specified dimmensions in the specified order. /// public void TranslateTransform(float dx, float dy, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipTranslateLineTransform(new HandleRef(this, this.NativeBrush), dx, dy, order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// /// Scales the local geometric transform by the /// specified amounts. This method prepends the scaling matrix to the transform. /// public void ScaleTransform(float sx, float sy) { ScaleTransform(sx, sy, MatrixOrder.Prepend); } ////// /// public void ScaleTransform(float sx, float sy, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipScaleLineTransform(new HandleRef(this, this.NativeBrush), sx, sy, order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Scales the local geometric transform by the /// specified amounts in the specified order. /// ////// /// Rotates the local geometric transform by the /// specified amount. This method prepends the rotation to the transform. /// public void RotateTransform(float angle) { RotateTransform(angle, MatrixOrder.Prepend); } ////// /// public void RotateTransform(float angle, MatrixOrder order) { int status = SafeNativeMethods.Gdip.GdipRotateLineTransform(new HandleRef(this, this.NativeBrush), angle, order); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Rotates the local geometric transform by the specified /// amount in the specified order. /// ///
Link Menu
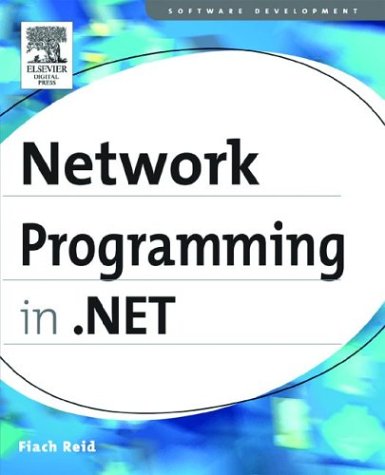
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Rule.cs
- IteratorAsyncResult.cs
- HttpCapabilitiesBase.cs
- ToolStripTextBox.cs
- SemaphoreFullException.cs
- UnsignedPublishLicense.cs
- DoubleLinkListEnumerator.cs
- HebrewNumber.cs
- IndexerNameAttribute.cs
- TypeNameConverter.cs
- MissingMemberException.cs
- StylusCaptureWithinProperty.cs
- MetabaseReader.cs
- Int16.cs
- ParameterBuilder.cs
- MissingManifestResourceException.cs
- MeasurementDCInfo.cs
- AdPostCacheSubstitution.cs
- MinimizableAttributeTypeConverter.cs
- ComNativeDescriptor.cs
- DBParameter.cs
- TdsParser.cs
- ToolboxItemLoader.cs
- CompModSwitches.cs
- DataGridViewCellStyleConverter.cs
- ParserExtension.cs
- XmlCDATASection.cs
- DataControlFieldCell.cs
- KerberosTicketHashIdentifierClause.cs
- TerminatorSinks.cs
- DateTime.cs
- WindowsSolidBrush.cs
- MultiDataTrigger.cs
- GZipDecoder.cs
- EventLogPermissionEntry.cs
- _TransmitFileOverlappedAsyncResult.cs
- ProtectedConfigurationProviderCollection.cs
- UTF7Encoding.cs
- ClientRolePrincipal.cs
- OdbcEnvironmentHandle.cs
- AuthorizationRule.cs
- MultipartContentParser.cs
- DataGridState.cs
- VisualCollection.cs
- MultilineStringConverter.cs
- SystemShuttingDownException.cs
- NumericUpDownAccelerationCollection.cs
- SqlIdentifier.cs
- MediaTimeline.cs
- SmtpCommands.cs
- ByeMessageCD1.cs
- BaseCAMarshaler.cs
- FileDataSource.cs
- TextEditorParagraphs.cs
- DocumentAutomationPeer.cs
- DataGridViewCellEventArgs.cs
- PlaceHolder.cs
- WebControlsSection.cs
- ProxyElement.cs
- StylusTip.cs
- AuthenticationException.cs
- DtrList.cs
- SafeThemeHandle.cs
- DataBinder.cs
- SiteMapNodeItemEventArgs.cs
- RtfControlWordInfo.cs
- FontResourceCache.cs
- HtmlInputImage.cs
- ReferenceSchema.cs
- CookieHandler.cs
- FormCollection.cs
- TextStore.cs
- ToolStripScrollButton.cs
- SimpleFileLog.cs
- NonVisualControlAttribute.cs
- PropertyDescriptorComparer.cs
- SessionState.cs
- PageFunction.cs
- ConfigXmlSignificantWhitespace.cs
- TimeoutValidationAttribute.cs
- EventLogTraceListener.cs
- DependencyPropertyAttribute.cs
- PolyBezierSegmentFigureLogic.cs
- ControlHelper.cs
- SecurityHeaderElementInferenceEngine.cs
- SystemTcpConnection.cs
- SqlXmlStorage.cs
- KerberosReceiverSecurityToken.cs
- Documentation.cs
- QilIterator.cs
- ButtonFlatAdapter.cs
- ScriptIgnoreAttribute.cs
- SizeAnimationUsingKeyFrames.cs
- ManifestBasedResourceGroveler.cs
- Context.cs
- XmlnsCompatibleWithAttribute.cs
- XPathNavigator.cs
- StreamWithDictionary.cs
- AuthenticatedStream.cs
- TargetPerspective.cs