Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Runtime / CompilerServices / MethodImplAttribute.cs / 1305376 / MethodImplAttribute.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Runtime.CompilerServices { using System; using System.Reflection; // This Enum matchs the miImpl flags defined in corhdr.h. It is used to specify // certain method properties. [Serializable] [Flags] [System.Runtime.InteropServices.ComVisible(true)] public enum MethodImplOptions { Unmanaged = System.Reflection.MethodImplAttributes.Unmanaged, ForwardRef = System.Reflection.MethodImplAttributes.ForwardRef, PreserveSig = System.Reflection.MethodImplAttributes.PreserveSig, InternalCall = System.Reflection.MethodImplAttributes.InternalCall, Synchronized = System.Reflection.MethodImplAttributes.Synchronized, NoInlining = System.Reflection.MethodImplAttributes.NoInlining, NoOptimization = System.Reflection.MethodImplAttributes.NoOptimization, // **** If you add something, update internal MethodImplAttribute(MethodImplAttributes methodImplAttributes)! **** } [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public enum MethodCodeType { IL = System.Reflection.MethodImplAttributes.IL, Native = System.Reflection.MethodImplAttributes.Native, ///OPTIL = System.Reflection.MethodImplAttributes.OPTIL, Runtime = System.Reflection.MethodImplAttributes.Runtime } // Custom attribute to specify additional method properties. [Serializable] [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor, Inherited = false)] [System.Runtime.InteropServices.ComVisible(true)] sealed public class MethodImplAttribute : Attribute { internal MethodImplOptions _val; public MethodCodeType MethodCodeType; internal MethodImplAttribute(MethodImplAttributes methodImplAttributes) { MethodImplOptions all = MethodImplOptions.Unmanaged | MethodImplOptions.ForwardRef | MethodImplOptions.PreserveSig | MethodImplOptions.InternalCall | MethodImplOptions.Synchronized | MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization; _val = ((MethodImplOptions)methodImplAttributes) & all; } public MethodImplAttribute(MethodImplOptions methodImplOptions) { _val = methodImplOptions; } public MethodImplAttribute(short value) { _val = (MethodImplOptions)value; } public MethodImplAttribute() { } public MethodImplOptions Value { get {return _val;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Runtime.CompilerServices { using System; using System.Reflection; // This Enum matchs the miImpl flags defined in corhdr.h. It is used to specify // certain method properties. [Serializable] [Flags] [System.Runtime.InteropServices.ComVisible(true)] public enum MethodImplOptions { Unmanaged = System.Reflection.MethodImplAttributes.Unmanaged, ForwardRef = System.Reflection.MethodImplAttributes.ForwardRef, PreserveSig = System.Reflection.MethodImplAttributes.PreserveSig, InternalCall = System.Reflection.MethodImplAttributes.InternalCall, Synchronized = System.Reflection.MethodImplAttributes.Synchronized, NoInlining = System.Reflection.MethodImplAttributes.NoInlining, NoOptimization = System.Reflection.MethodImplAttributes.NoOptimization, // **** If you add something, update internal MethodImplAttribute(MethodImplAttributes methodImplAttributes)! **** } [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public enum MethodCodeType { IL = System.Reflection.MethodImplAttributes.IL, Native = System.Reflection.MethodImplAttributes.Native, /// OPTIL = System.Reflection.MethodImplAttributes.OPTIL, Runtime = System.Reflection.MethodImplAttributes.Runtime } // Custom attribute to specify additional method properties. [Serializable] [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor, Inherited = false)] [System.Runtime.InteropServices.ComVisible(true)] sealed public class MethodImplAttribute : Attribute { internal MethodImplOptions _val; public MethodCodeType MethodCodeType; internal MethodImplAttribute(MethodImplAttributes methodImplAttributes) { MethodImplOptions all = MethodImplOptions.Unmanaged | MethodImplOptions.ForwardRef | MethodImplOptions.PreserveSig | MethodImplOptions.InternalCall | MethodImplOptions.Synchronized | MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization; _val = ((MethodImplOptions)methodImplAttributes) & all; } public MethodImplAttribute(MethodImplOptions methodImplOptions) { _val = methodImplOptions; } public MethodImplAttribute(short value) { _val = (MethodImplOptions)value; } public MethodImplAttribute() { } public MethodImplOptions Value { get {return _val;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
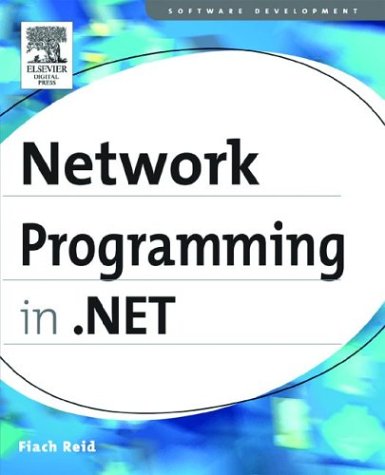
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CalendarData.cs
- Camera.cs
- ZoneLinkButton.cs
- GeneratedView.cs
- SingleAnimationBase.cs
- AssociationSetEnd.cs
- WeakReferenceKey.cs
- MessageSecurityProtocolFactory.cs
- SettingsAttributes.cs
- CompilerWrapper.cs
- RSACryptoServiceProvider.cs
- TraceHandlerErrorFormatter.cs
- PropertyPath.cs
- UiaCoreTypesApi.cs
- DataGridColumnHeaderAutomationPeer.cs
- CodeNamespaceCollection.cs
- Regex.cs
- DataGridColumnDropSeparator.cs
- SocketPermission.cs
- Identifier.cs
- NextPreviousPagerField.cs
- CoTaskMemHandle.cs
- WindowsFormsLinkLabel.cs
- CharacterMetricsDictionary.cs
- externdll.cs
- UIElementAutomationPeer.cs
- ColorDialog.cs
- URIFormatException.cs
- BuilderInfo.cs
- DeobfuscatingStream.cs
- Point4DValueSerializer.cs
- StaticFileHandler.cs
- HttpWebRequestElement.cs
- CompressionTransform.cs
- OdbcConnectionOpen.cs
- CodeCastExpression.cs
- TriggerBase.cs
- NativeRecognizer.cs
- EventItfInfo.cs
- XPathSingletonIterator.cs
- InputBinder.cs
- PathFigure.cs
- Brush.cs
- XmlSchemaSet.cs
- ListenerBinder.cs
- DrawingContextDrawingContextWalker.cs
- SafeLibraryHandle.cs
- OperationCanceledException.cs
- SoapInteropTypes.cs
- WSSecurityPolicy12.cs
- ListViewItem.cs
- backend.cs
- DtdParser.cs
- IBuiltInEvidence.cs
- CapabilitiesState.cs
- MsmqOutputChannel.cs
- ImmComposition.cs
- ToolboxItemAttribute.cs
- ExtenderControl.cs
- PolicyValidationException.cs
- ReverseInheritProperty.cs
- SignatureResourcePool.cs
- ValidatingPropertiesEventArgs.cs
- PerformanceCounterPermissionEntry.cs
- COAUTHIDENTITY.cs
- WebPartEditorApplyVerb.cs
- ProcessHost.cs
- BmpBitmapDecoder.cs
- SystemInformation.cs
- GlobalizationSection.cs
- StringWriter.cs
- RemoteWebConfigurationHostServer.cs
- DataGridViewTextBoxCell.cs
- ImageBrush.cs
- PassportPrincipal.cs
- AsymmetricKeyExchangeDeformatter.cs
- ActivationArguments.cs
- LowerCaseStringConverter.cs
- ObjectQuery.cs
- ToolStripSeparator.cs
- InlinedLocationReference.cs
- RepeaterDesigner.cs
- BooleanToVisibilityConverter.cs
- UnsettableComboBox.cs
- CacheMemory.cs
- SQLUtility.cs
- COM2ExtendedUITypeEditor.cs
- CompilationSection.cs
- PathFigureCollectionConverter.cs
- EUCJPEncoding.cs
- ParserStreamGeometryContext.cs
- FileDialog.cs
- CollectionsUtil.cs
- DataMisalignedException.cs
- StrongName.cs
- CellConstant.cs
- SQLDateTime.cs
- WeakReferenceList.cs
- MenuEventArgs.cs
- FloatUtil.cs