Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Behaviors / SynchronizationScope.cs / 1305376 / SynchronizationScope.cs
namespace System.Workflow.ComponentModel { #region Imports using System; using System.Globalization; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using System.Drawing.Design; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; using System.Workflow.ComponentModel.Compiler; #endregion [SRDescription(SR.SynchronizationScopeActivityDescription)] [ToolboxItem(typeof(ActivityToolboxItem))] [ToolboxBitmap(typeof(SynchronizationScopeActivity), "Resources.Sequence.png")] [SupportsSynchronization] [Designer(typeof(SequenceDesigner), typeof(IDesigner))] public sealed class SynchronizationScopeActivity : CompositeActivity, IActivityEventListener{ public SynchronizationScopeActivity() { } public SynchronizationScopeActivity(string name) :base(name) { } [SRDisplayName(SR.SynchronizationHandles)] [SRDescription(SR.SynchronizationHandlesDesc)] [TypeConverter(typeof(SynchronizationHandlesTypeConverter))] [EditorAttribute(typeof(SynchronizationHandlesEditor), typeof(System.Drawing.Design.UITypeEditor))] public ICollection SynchronizationHandles { get { return this.GetValue(SynchronizationHandlesProperty) as ICollection ; } set { this.SetValue(SynchronizationHandlesProperty, value); } } protected internal override ActivityExecutionStatus Execute(ActivityExecutionContext executionContext) { return SequenceHelper.Execute(this, executionContext); } protected internal override ActivityExecutionStatus Cancel(ActivityExecutionContext executionContext) { return SequenceHelper.Cancel(this, executionContext); } void IActivityEventListener .OnEvent(Object sender, ActivityExecutionStatusChangedEventArgs e) { SequenceHelper.OnEvent(this, sender, e); } protected internal override void OnActivityChangeRemove(ActivityExecutionContext executionContext, Activity removedActivity) { SequenceHelper.OnActivityChangeRemove(this, executionContext, removedActivity); } protected internal override void OnWorkflowChangesCompleted(ActivityExecutionContext executionContext) { SequenceHelper.OnWorkflowChangesCompleted(this, executionContext); } } #region Class SynchronizationHandlesTypeConverter internal sealed class SynchronizationHandlesTypeConverter : TypeConverter { public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) return true; return base.CanConvertTo(context, destinationType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && value is ICollection ) return Stringify(value as ICollection ); return base.ConvertTo(context, culture, value, destinationType); } public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) return true; return base.CanConvertFrom(context, sourceType); } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) return UnStringify(value as string); return base.ConvertFrom(context, culture, value); } internal static string Stringify(ICollection synchronizationHandles) { string stringifiedValue = string.Empty; if (synchronizationHandles == null) return stringifiedValue; foreach (string handle in synchronizationHandles) { if (handle == null) continue; if (stringifiedValue != string.Empty) stringifiedValue += ", "; stringifiedValue += handle.Replace(",", "\\,"); } return stringifiedValue; } internal static ICollection UnStringify(string stringifiedValue) { ICollection synchronizationHandles = new List (); stringifiedValue = stringifiedValue.Replace("\\,", ">"); foreach (string handle in stringifiedValue.Split(new char[] { ',', '\r', '\n' }, StringSplitOptions.RemoveEmptyEntries)) { string realHandle = handle.Trim().Replace('>', ','); if (realHandle != string.Empty && !synchronizationHandles.Contains(realHandle)) synchronizationHandles.Add(realHandle); } return synchronizationHandles; } } #endregion internal sealed class SynchronizationHandlesEditor : UITypeEditor { private MultilineStringEditor stringEditor = new MultilineStringEditor(); public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { string stringValue = SynchronizationHandlesTypeConverter.Stringify(value as ICollection ); stringValue = stringEditor.EditValue(context, provider, stringValue) as string; value = SynchronizationHandlesTypeConverter.UnStringify(stringValue); return value; } public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return stringEditor.GetEditStyle(context); } public override bool GetPaintValueSupported(ITypeDescriptorContext context) { return stringEditor.GetPaintValueSupported(context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel { #region Imports using System; using System.Globalization; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using System.Drawing.Design; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; using System.Workflow.ComponentModel.Compiler; #endregion [SRDescription(SR.SynchronizationScopeActivityDescription)] [ToolboxItem(typeof(ActivityToolboxItem))] [ToolboxBitmap(typeof(SynchronizationScopeActivity), "Resources.Sequence.png")] [SupportsSynchronization] [Designer(typeof(SequenceDesigner), typeof(IDesigner))] public sealed class SynchronizationScopeActivity : CompositeActivity, IActivityEventListener { public SynchronizationScopeActivity() { } public SynchronizationScopeActivity(string name) :base(name) { } [SRDisplayName(SR.SynchronizationHandles)] [SRDescription(SR.SynchronizationHandlesDesc)] [TypeConverter(typeof(SynchronizationHandlesTypeConverter))] [EditorAttribute(typeof(SynchronizationHandlesEditor), typeof(System.Drawing.Design.UITypeEditor))] public ICollection SynchronizationHandles { get { return this.GetValue(SynchronizationHandlesProperty) as ICollection ; } set { this.SetValue(SynchronizationHandlesProperty, value); } } protected internal override ActivityExecutionStatus Execute(ActivityExecutionContext executionContext) { return SequenceHelper.Execute(this, executionContext); } protected internal override ActivityExecutionStatus Cancel(ActivityExecutionContext executionContext) { return SequenceHelper.Cancel(this, executionContext); } void IActivityEventListener .OnEvent(Object sender, ActivityExecutionStatusChangedEventArgs e) { SequenceHelper.OnEvent(this, sender, e); } protected internal override void OnActivityChangeRemove(ActivityExecutionContext executionContext, Activity removedActivity) { SequenceHelper.OnActivityChangeRemove(this, executionContext, removedActivity); } protected internal override void OnWorkflowChangesCompleted(ActivityExecutionContext executionContext) { SequenceHelper.OnWorkflowChangesCompleted(this, executionContext); } } #region Class SynchronizationHandlesTypeConverter internal sealed class SynchronizationHandlesTypeConverter : TypeConverter { public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) return true; return base.CanConvertTo(context, destinationType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && value is ICollection ) return Stringify(value as ICollection ); return base.ConvertTo(context, culture, value, destinationType); } public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) return true; return base.CanConvertFrom(context, sourceType); } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) return UnStringify(value as string); return base.ConvertFrom(context, culture, value); } internal static string Stringify(ICollection synchronizationHandles) { string stringifiedValue = string.Empty; if (synchronizationHandles == null) return stringifiedValue; foreach (string handle in synchronizationHandles) { if (handle == null) continue; if (stringifiedValue != string.Empty) stringifiedValue += ", "; stringifiedValue += handle.Replace(",", "\\,"); } return stringifiedValue; } internal static ICollection UnStringify(string stringifiedValue) { ICollection synchronizationHandles = new List (); stringifiedValue = stringifiedValue.Replace("\\,", ">"); foreach (string handle in stringifiedValue.Split(new char[] { ',', '\r', '\n' }, StringSplitOptions.RemoveEmptyEntries)) { string realHandle = handle.Trim().Replace('>', ','); if (realHandle != string.Empty && !synchronizationHandles.Contains(realHandle)) synchronizationHandles.Add(realHandle); } return synchronizationHandles; } } #endregion internal sealed class SynchronizationHandlesEditor : UITypeEditor { private MultilineStringEditor stringEditor = new MultilineStringEditor(); public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { string stringValue = SynchronizationHandlesTypeConverter.Stringify(value as ICollection ); stringValue = stringEditor.EditValue(context, provider, stringValue) as string; value = SynchronizationHandlesTypeConverter.UnStringify(stringValue); return value; } public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return stringEditor.GetEditStyle(context); } public override bool GetPaintValueSupported(ITypeDescriptorContext context) { return stringEditor.GetPaintValueSupported(context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
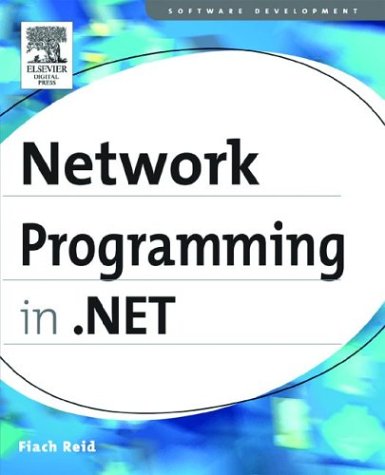
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsltLibrary.cs
- CodeNamespaceCollection.cs
- DelegateArgumentValue.cs
- PropertyGridView.cs
- SingleSelectRootGridEntry.cs
- AudioLevelUpdatedEventArgs.cs
- PhysicalAddress.cs
- DataGridViewCheckBoxCell.cs
- DropTarget.cs
- ProcessHostMapPath.cs
- GZipStream.cs
- EventLogPermissionEntry.cs
- PropertyEntry.cs
- WebPartEditorCancelVerb.cs
- ReflectPropertyDescriptor.cs
- DbConnectionPool.cs
- RelatedImageListAttribute.cs
- TextViewSelectionProcessor.cs
- XmlQualifiedName.cs
- DiffuseMaterial.cs
- DrawListViewSubItemEventArgs.cs
- WpfGeneratedKnownProperties.cs
- TouchPoint.cs
- LineInfo.cs
- LineServicesCallbacks.cs
- MissingFieldException.cs
- AnimationStorage.cs
- ColumnMapTranslator.cs
- PageStatePersister.cs
- ProfileModule.cs
- DecimalConstantAttribute.cs
- SQLDecimalStorage.cs
- ChangePassword.cs
- Permission.cs
- Floater.cs
- EmissiveMaterial.cs
- TrackingProfile.cs
- IndicShape.cs
- EdgeModeValidation.cs
- PlatformNotSupportedException.cs
- ListControlBoundActionList.cs
- NativeObjectSecurity.cs
- CodeDOMUtility.cs
- RuntimeHandles.cs
- DataGridColumn.cs
- AutoResetEvent.cs
- GridViewSelectEventArgs.cs
- SessionStateModule.cs
- IntranetCredentialPolicy.cs
- SharedPersonalizationStateInfo.cs
- ControlAdapter.cs
- SafeThemeHandle.cs
- Soap12ServerProtocol.cs
- Debug.cs
- ScrollItemPatternIdentifiers.cs
- WmpBitmapDecoder.cs
- InstanceDataCollectionCollection.cs
- RuleSet.cs
- HttpInputStream.cs
- PartialClassGenerationTaskInternal.cs
- HelpEvent.cs
- VScrollProperties.cs
- NullableDoubleMinMaxAggregationOperator.cs
- Matrix.cs
- XmlTextWriter.cs
- UidManager.cs
- _UriSyntax.cs
- ValidatorCollection.cs
- ScriptReferenceBase.cs
- OLEDB_Util.cs
- TypeUsageBuilder.cs
- ListBoxChrome.cs
- CacheRequest.cs
- ActiveXHost.cs
- COM2ICategorizePropertiesHandler.cs
- HandoffBehavior.cs
- ResourceProperty.cs
- ProcessDesigner.cs
- SingleConverter.cs
- BinaryFormatter.cs
- SignatureDescription.cs
- BamlStream.cs
- Process.cs
- ObjectQueryProvider.cs
- HttpServerVarsCollection.cs
- ExpressionBinding.cs
- GridViewSortEventArgs.cs
- DefinitionUpdate.cs
- XmlDataSourceNodeDescriptor.cs
- ValueUnavailableException.cs
- FixedBufferAttribute.cs
- AbsoluteQuery.cs
- EventLogLink.cs
- DependencyPropertyAttribute.cs
- ComboBoxDesigner.cs
- ItemsControlAutomationPeer.cs
- DataGridViewCellConverter.cs
- UdpContractFilterBehavior.cs
- StreamDocument.cs
- FrameworkPropertyMetadata.cs