Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SymmetricKey.cs / 1305376 / SymmetricKey.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.IdentityModel.Selectors; using System.Security.Cryptography; public class InMemorySymmetricSecurityKey : SymmetricSecurityKey { int keySize; byte[] symmetricKey; public InMemorySymmetricSecurityKey(byte[] symmetricKey) : this(symmetricKey, true) { } public InMemorySymmetricSecurityKey(byte[] symmetricKey, bool cloneBuffer) { if (symmetricKey == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("symmetricKey")); } if (symmetricKey.Length == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SymmetricKeyLengthTooShort, symmetricKey.Length))); } this.keySize = symmetricKey.Length * 8; if (cloneBuffer) { this.symmetricKey = new byte[symmetricKey.Length]; Buffer.BlockCopy(symmetricKey, 0, this.symmetricKey, 0, symmetricKey.Length); } else { this.symmetricKey = symmetricKey; } } public override int KeySize { get { return this.keySize; } } public override byte[] DecryptKey(string algorithm, byte[] keyData) { return CryptoHelper.UnwrapKey(this.symmetricKey, keyData, algorithm); } public override byte[] EncryptKey(string algorithm, byte[] keyData) { return CryptoHelper.WrapKey(this.symmetricKey, keyData, algorithm); } public override byte[] GenerateDerivedKey(string algorithm, byte[] label, byte[] nonce, int derivedKeyLength, int offset) { return CryptoHelper.GenerateDerivedKey(this.symmetricKey, algorithm, label, nonce, derivedKeyLength, offset); } public override ICryptoTransform GetDecryptionTransform(string algorithm, byte[] iv) { return CryptoHelper.CreateDecryptor(this.symmetricKey, iv, algorithm); } public override ICryptoTransform GetEncryptionTransform(string algorithm, byte[] iv) { return CryptoHelper.CreateEncryptor(this.symmetricKey, iv, algorithm); } public override int GetIVSize(string algorithm) { return CryptoHelper.GetIVSize(algorithm); } public override KeyedHashAlgorithm GetKeyedHashAlgorithm(string algorithm) { return CryptoHelper.CreateKeyedHashAlgorithm(this.symmetricKey, algorithm); } public override SymmetricAlgorithm GetSymmetricAlgorithm(string algorithm) { return CryptoHelper.GetSymmetricAlgorithm(this.symmetricKey, algorithm); } public override byte[] GetSymmetricKey() { byte[] local = new byte[this.symmetricKey.Length]; Buffer.BlockCopy(this.symmetricKey, 0, local, 0, this.symmetricKey.Length); return local; } public override bool IsAsymmetricAlgorithm(string algorithm) { return (CryptoHelper.IsAsymmetricAlgorithm(algorithm)); } public override bool IsSupportedAlgorithm(string algorithm) { return (CryptoHelper.IsSymmetricSupportedAlgorithm(algorithm, this.KeySize)); } public override bool IsSymmetricAlgorithm(string algorithm) { return CryptoHelper.IsSymmetricAlgorithm(algorithm); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.IdentityModel.Selectors; using System.Security.Cryptography; public class InMemorySymmetricSecurityKey : SymmetricSecurityKey { int keySize; byte[] symmetricKey; public InMemorySymmetricSecurityKey(byte[] symmetricKey) : this(symmetricKey, true) { } public InMemorySymmetricSecurityKey(byte[] symmetricKey, bool cloneBuffer) { if (symmetricKey == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("symmetricKey")); } if (symmetricKey.Length == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SymmetricKeyLengthTooShort, symmetricKey.Length))); } this.keySize = symmetricKey.Length * 8; if (cloneBuffer) { this.symmetricKey = new byte[symmetricKey.Length]; Buffer.BlockCopy(symmetricKey, 0, this.symmetricKey, 0, symmetricKey.Length); } else { this.symmetricKey = symmetricKey; } } public override int KeySize { get { return this.keySize; } } public override byte[] DecryptKey(string algorithm, byte[] keyData) { return CryptoHelper.UnwrapKey(this.symmetricKey, keyData, algorithm); } public override byte[] EncryptKey(string algorithm, byte[] keyData) { return CryptoHelper.WrapKey(this.symmetricKey, keyData, algorithm); } public override byte[] GenerateDerivedKey(string algorithm, byte[] label, byte[] nonce, int derivedKeyLength, int offset) { return CryptoHelper.GenerateDerivedKey(this.symmetricKey, algorithm, label, nonce, derivedKeyLength, offset); } public override ICryptoTransform GetDecryptionTransform(string algorithm, byte[] iv) { return CryptoHelper.CreateDecryptor(this.symmetricKey, iv, algorithm); } public override ICryptoTransform GetEncryptionTransform(string algorithm, byte[] iv) { return CryptoHelper.CreateEncryptor(this.symmetricKey, iv, algorithm); } public override int GetIVSize(string algorithm) { return CryptoHelper.GetIVSize(algorithm); } public override KeyedHashAlgorithm GetKeyedHashAlgorithm(string algorithm) { return CryptoHelper.CreateKeyedHashAlgorithm(this.symmetricKey, algorithm); } public override SymmetricAlgorithm GetSymmetricAlgorithm(string algorithm) { return CryptoHelper.GetSymmetricAlgorithm(this.symmetricKey, algorithm); } public override byte[] GetSymmetricKey() { byte[] local = new byte[this.symmetricKey.Length]; Buffer.BlockCopy(this.symmetricKey, 0, local, 0, this.symmetricKey.Length); return local; } public override bool IsAsymmetricAlgorithm(string algorithm) { return (CryptoHelper.IsAsymmetricAlgorithm(algorithm)); } public override bool IsSupportedAlgorithm(string algorithm) { return (CryptoHelper.IsSymmetricSupportedAlgorithm(algorithm, this.KeySize)); } public override bool IsSymmetricAlgorithm(string algorithm) { return CryptoHelper.IsSymmetricAlgorithm(algorithm); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
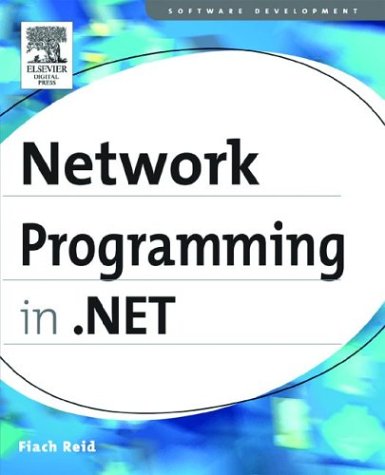
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServicePoint.cs
- ToolStripDropTargetManager.cs
- ACL.cs
- PropertyGrid.cs
- DbConnectionOptions.cs
- SchemaDeclBase.cs
- InvalidCommandTreeException.cs
- followingsibling.cs
- ImageMapEventArgs.cs
- StorageComplexTypeMapping.cs
- BitmapInitialize.cs
- altserialization.cs
- AlternateView.cs
- MarshalByRefObject.cs
- BoundsDrawingContextWalker.cs
- CommonRemoteMemoryBlock.cs
- UncommonField.cs
- Vector3DCollectionConverter.cs
- DataGridColumnDropSeparator.cs
- RichTextBox.cs
- BitmapEffectInput.cs
- HashMembershipCondition.cs
- WCFServiceClientProxyGenerator.cs
- DataBindEngine.cs
- DBSchemaTable.cs
- PolyLineSegmentFigureLogic.cs
- RegexFCD.cs
- MinMaxParagraphWidth.cs
- DataColumnCollection.cs
- DecimalStorage.cs
- DetailsViewRow.cs
- PageSettings.cs
- DropTarget.cs
- Column.cs
- GridEntryCollection.cs
- TreeNodeCollectionEditor.cs
- SerializationUtility.cs
- _ConnectOverlappedAsyncResult.cs
- CanExecuteRoutedEventArgs.cs
- HttpHostedTransportConfiguration.cs
- EncoderNLS.cs
- ViewStateModeByIdAttribute.cs
- ModulesEntry.cs
- StateInitializationDesigner.cs
- DataGridViewComboBoxCell.cs
- RichTextBox.cs
- DesignerForm.cs
- ViewKeyConstraint.cs
- PropertyInfoSet.cs
- DurableInstance.cs
- wmiutil.cs
- ExceptionRoutedEventArgs.cs
- TrustLevel.cs
- ListViewInsertionMark.cs
- TransformCollection.cs
- GlyphInfoList.cs
- AnnotationHighlightLayer.cs
- ThousandthOfEmRealPoints.cs
- LinqToSqlWrapper.cs
- BufferedReadStream.cs
- DetectEofStream.cs
- ProcessingInstructionAction.cs
- PartitionResolver.cs
- PropertyPathWorker.cs
- XmlSchemaComplexContent.cs
- iisPickupDirectory.cs
- TableCellCollection.cs
- OleDbTransaction.cs
- dbdatarecord.cs
- SettingsSavedEventArgs.cs
- StylusPointPropertyInfoDefaults.cs
- EntityDataSourceContextCreatingEventArgs.cs
- SchemaTypeEmitter.cs
- Tuple.cs
- TransformationRules.cs
- XmlSchemaAnnotated.cs
- HostDesigntimeLicenseContext.cs
- EntitySetBaseCollection.cs
- AuthenticationModuleElement.cs
- AsyncResult.cs
- TextServicesProperty.cs
- ZipIOExtraField.cs
- WriteFileContext.cs
- TreePrinter.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- BamlBinaryReader.cs
- TextServicesHost.cs
- EntityTransaction.cs
- BamlRecordReader.cs
- SeverityFilter.cs
- MultiPropertyDescriptorGridEntry.cs
- Token.cs
- RawAppCommandInputReport.cs
- ValidationVisibilityAttribute.cs
- HTTP_SERVICE_CONFIG_URLACL_KEY.cs
- ReceiveCompletedEventArgs.cs
- InputMethodStateChangeEventArgs.cs
- XmlSchemaIdentityConstraint.cs
- BindingWorker.cs
- PartBasedPackageProperties.cs