Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / ModelPropertyImpl.cs / 1305376 / ModelPropertyImpl.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections.Generic; using System.ComponentModel; using System.Windows.Documents; // This class provides the implementation for a model property. // this intercepts sets /gets to the property and works with modeltreemanager // to keep the xaml in [....]. class ModelPropertyImpl : ModelProperty { object defaultValue; ModelItem parent; PropertyDescriptor propertyDescriptor; AttributeCollection attributes; bool isAttached = false; public ModelPropertyImpl(ModelItem parent, PropertyDescriptor propertyDescriptor, bool isAttached) { this.parent = parent; this.propertyDescriptor = propertyDescriptor; // using this a marker to indicate this hasnt been computed yet. this.defaultValue = this; this.isAttached = isAttached; } public override Type AttachedOwnerType { get { return this.parent.GetType(); } } public override AttributeCollection Attributes { get { if (this.attributes == null) { if (propertyDescriptor.ComponentType.IsGenericType) { ListattributeList = new List (this.propertyDescriptor.Attributes.Count); foreach (Attribute attr in this.propertyDescriptor.Attributes) { attributeList.Add(attr); } // Merge propertyDescriptor.Attributes with generic type definition Attributes Type genericInstanceType = propertyDescriptor.ComponentType.GetGenericTypeDefinition(); PropertyDescriptor pd = TypeDescriptor.GetProvider(genericInstanceType).GetTypeDescriptor(genericInstanceType).GetProperties()[this.Name]; if (null != pd) { foreach (Attribute attr in pd.Attributes) { if (!attributeList.Contains(attr)) { attributeList.Add(attr); } } } this.attributes = new AttributeCollection(attributeList.ToArray()); } else { this.attributes = propertyDescriptor.Attributes; } } return this.attributes; } } public override ModelItemCollection Collection { get { return this.Value as ModelItemCollection; } } public override object ComputedValue { get { object computedValue = null; if (this.Value != null) { computedValue = Value.GetCurrentValue(); } return computedValue; } set { SetValue(value); } } public override System.ComponentModel.TypeConverter Converter { get { return new ModelTypeConverter(((IModelTreeItem)this.Parent).ModelTreeManager, propertyDescriptor.Converter); } } public override object DefaultValue { get { if (Object.ReferenceEquals(this.defaultValue, this)) { DefaultValueAttribute defaultValueAttribute = this.propertyDescriptor.Attributes[typeof(DefaultValueAttribute)] as DefaultValueAttribute; this.defaultValue = (defaultValueAttribute == null) ? null : defaultValueAttribute.Value; } return this.defaultValue; } } public override ModelItemDictionary Dictionary { get { return Value as ModelItemDictionary; } } public override bool IsAttached { get { return this.isAttached; } } public override bool IsBrowsable { get { return propertyDescriptor.IsBrowsable; } } public override bool IsCollection { get { return this.Value is ModelItemCollection; } } public override bool IsDictionary { get { return this.Value is ModelItemDictionary; } } public override bool IsReadOnly { get { return propertyDescriptor.IsReadOnly; } } public override bool IsSet { get { return this.Value != null; } } public override string Name { get { return propertyDescriptor.Name; } } public override ModelItem Parent { get { return parent; } } public override Type PropertyType { get { return propertyDescriptor.PropertyType; } } public override ModelItem Value { get { return ((IModelTreeItem)parent).ModelTreeManager.GetValue(this); } } internal PropertyDescriptor PropertyDescriptor { get { return propertyDescriptor; } } public override void ClearValue() { if (!this.IsReadOnly) { ((IModelTreeItem)parent).ModelTreeManager.ClearValue(this); } } public override ModelItem SetValue(object value) { return ((IModelTreeItem)parent).ModelTreeManager.SetValue(this, value); } internal object SetValueCore(ModelItem newValueModelItem) { object newValueInstance = (newValueModelItem == null) ? null : newValueModelItem.GetCurrentValue(); ModelItem oldValueModelItem = this.Value; IModelTreeItem parent = (IModelTreeItem)this.Parent; // try update the instance this.PropertyDescriptor.SetValue(this.Parent.GetCurrentValue(), newValueInstance); // if old value is not null remove parent/source from it and release it if (oldValueModelItem != null) { ((IModelTreeItem)oldValueModelItem).RemoveSource(this); parent.ModelTreeManager.ReleaseModelItem(oldValueModelItem, this.Parent); } // if new value is non null, update parent/source for it. if (newValueModelItem != null) { parent.ModelTreeManager.ReAddModelItemToModelTree(newValueModelItem); parent.ModelPropertyStore[this.Name] = newValueModelItem; ((IModelTreeItem)newValueModelItem).SetSource(this); } else { parent.ModelPropertyStore.Remove(this.Name); } // notify observers ((IModelTreeItem)this.Parent).OnPropertyChanged(this.Name); return newValueInstance; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections.Generic; using System.ComponentModel; using System.Windows.Documents; // This class provides the implementation for a model property. // this intercepts sets /gets to the property and works with modeltreemanager // to keep the xaml in [....]. class ModelPropertyImpl : ModelProperty { object defaultValue; ModelItem parent; PropertyDescriptor propertyDescriptor; AttributeCollection attributes; bool isAttached = false; public ModelPropertyImpl(ModelItem parent, PropertyDescriptor propertyDescriptor, bool isAttached) { this.parent = parent; this.propertyDescriptor = propertyDescriptor; // using this a marker to indicate this hasnt been computed yet. this.defaultValue = this; this.isAttached = isAttached; } public override Type AttachedOwnerType { get { return this.parent.GetType(); } } public override AttributeCollection Attributes { get { if (this.attributes == null) { if (propertyDescriptor.ComponentType.IsGenericType) { List attributeList = new List (this.propertyDescriptor.Attributes.Count); foreach (Attribute attr in this.propertyDescriptor.Attributes) { attributeList.Add(attr); } // Merge propertyDescriptor.Attributes with generic type definition Attributes Type genericInstanceType = propertyDescriptor.ComponentType.GetGenericTypeDefinition(); PropertyDescriptor pd = TypeDescriptor.GetProvider(genericInstanceType).GetTypeDescriptor(genericInstanceType).GetProperties()[this.Name]; if (null != pd) { foreach (Attribute attr in pd.Attributes) { if (!attributeList.Contains(attr)) { attributeList.Add(attr); } } } this.attributes = new AttributeCollection(attributeList.ToArray()); } else { this.attributes = propertyDescriptor.Attributes; } } return this.attributes; } } public override ModelItemCollection Collection { get { return this.Value as ModelItemCollection; } } public override object ComputedValue { get { object computedValue = null; if (this.Value != null) { computedValue = Value.GetCurrentValue(); } return computedValue; } set { SetValue(value); } } public override System.ComponentModel.TypeConverter Converter { get { return new ModelTypeConverter(((IModelTreeItem)this.Parent).ModelTreeManager, propertyDescriptor.Converter); } } public override object DefaultValue { get { if (Object.ReferenceEquals(this.defaultValue, this)) { DefaultValueAttribute defaultValueAttribute = this.propertyDescriptor.Attributes[typeof(DefaultValueAttribute)] as DefaultValueAttribute; this.defaultValue = (defaultValueAttribute == null) ? null : defaultValueAttribute.Value; } return this.defaultValue; } } public override ModelItemDictionary Dictionary { get { return Value as ModelItemDictionary; } } public override bool IsAttached { get { return this.isAttached; } } public override bool IsBrowsable { get { return propertyDescriptor.IsBrowsable; } } public override bool IsCollection { get { return this.Value is ModelItemCollection; } } public override bool IsDictionary { get { return this.Value is ModelItemDictionary; } } public override bool IsReadOnly { get { return propertyDescriptor.IsReadOnly; } } public override bool IsSet { get { return this.Value != null; } } public override string Name { get { return propertyDescriptor.Name; } } public override ModelItem Parent { get { return parent; } } public override Type PropertyType { get { return propertyDescriptor.PropertyType; } } public override ModelItem Value { get { return ((IModelTreeItem)parent).ModelTreeManager.GetValue(this); } } internal PropertyDescriptor PropertyDescriptor { get { return propertyDescriptor; } } public override void ClearValue() { if (!this.IsReadOnly) { ((IModelTreeItem)parent).ModelTreeManager.ClearValue(this); } } public override ModelItem SetValue(object value) { return ((IModelTreeItem)parent).ModelTreeManager.SetValue(this, value); } internal object SetValueCore(ModelItem newValueModelItem) { object newValueInstance = (newValueModelItem == null) ? null : newValueModelItem.GetCurrentValue(); ModelItem oldValueModelItem = this.Value; IModelTreeItem parent = (IModelTreeItem)this.Parent; // try update the instance this.PropertyDescriptor.SetValue(this.Parent.GetCurrentValue(), newValueInstance); // if old value is not null remove parent/source from it and release it if (oldValueModelItem != null) { ((IModelTreeItem)oldValueModelItem).RemoveSource(this); parent.ModelTreeManager.ReleaseModelItem(oldValueModelItem, this.Parent); } // if new value is non null, update parent/source for it. if (newValueModelItem != null) { parent.ModelTreeManager.ReAddModelItemToModelTree(newValueModelItem); parent.ModelPropertyStore[this.Name] = newValueModelItem; ((IModelTreeItem)newValueModelItem).SetSource(this); } else { parent.ModelPropertyStore.Remove(this.Name); } // notify observers ((IModelTreeItem)this.Parent).OnPropertyChanged(this.Name); return newValueInstance; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
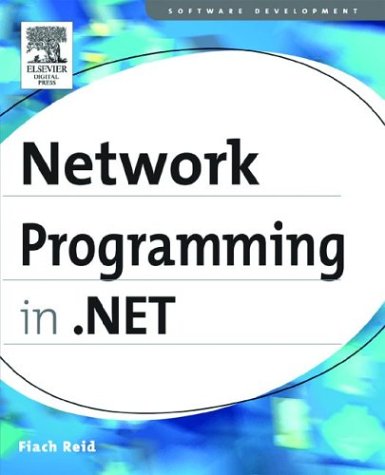
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProxyFragment.cs
- CapabilitiesPattern.cs
- VisualBasicReference.cs
- CodeAttachEventStatement.cs
- ConfigurationSectionCollection.cs
- StaticTextPointer.cs
- ColumnMapTranslator.cs
- VBIdentifierNameEditor.cs
- WindowsAltTab.cs
- SaveRecipientRequest.cs
- CalendarAutoFormat.cs
- Journal.cs
- AmbientLight.cs
- sqlstateclientmanager.cs
- IndexedEnumerable.cs
- UnsafeNativeMethods.cs
- ExpressionDumper.cs
- SmtpAuthenticationManager.cs
- ContractNamespaceAttribute.cs
- MenuAdapter.cs
- SmtpDigestAuthenticationModule.cs
- LinkLabelLinkClickedEvent.cs
- Page.cs
- PrivacyNoticeBindingElement.cs
- DataGridViewAdvancedBorderStyle.cs
- DbProviderFactoriesConfigurationHandler.cs
- Visual3DCollection.cs
- SymmetricKeyWrap.cs
- DataGridViewAdvancedBorderStyle.cs
- AsymmetricKeyExchangeFormatter.cs
- Geometry3D.cs
- ScrollChrome.cs
- RelationshipSet.cs
- ConnectionStringsExpressionBuilder.cs
- ToolStripSettings.cs
- ObfuscationAttribute.cs
- AttributeCollection.cs
- QueryOutputWriter.cs
- PropertyStore.cs
- InvokePatternIdentifiers.cs
- SoapTypeAttribute.cs
- IPAddress.cs
- Keywords.cs
- IConvertible.cs
- OrthographicCamera.cs
- EnumConverter.cs
- SamlConstants.cs
- XmlSerializerSection.cs
- DataList.cs
- FactoryGenerator.cs
- EventDescriptor.cs
- ExpandCollapseProviderWrapper.cs
- DragEvent.cs
- UnwrappedTypesXmlSerializerManager.cs
- _LocalDataStore.cs
- GradientSpreadMethodValidation.cs
- Constraint.cs
- VirtualizingStackPanel.cs
- XmlSchemaAttribute.cs
- InternalControlCollection.cs
- ThemeInfoAttribute.cs
- ProtocolsConfigurationEntry.cs
- ImageIndexEditor.cs
- SqlInternalConnectionSmi.cs
- PriorityChain.cs
- WsatServiceCertificate.cs
- PinnedBufferMemoryStream.cs
- Animatable.cs
- ValueProviderWrapper.cs
- WebBrowser.cs
- PersonalizationState.cs
- GlobalizationSection.cs
- newinstructionaction.cs
- FrameworkTextComposition.cs
- Pointer.cs
- UrlPropertyAttribute.cs
- _Win32.cs
- HwndSubclass.cs
- RadialGradientBrush.cs
- RectValueSerializer.cs
- OrderedParallelQuery.cs
- HtmlInputImage.cs
- TimeSpanConverter.cs
- PowerStatus.cs
- PolicyValidationException.cs
- NativeMethods.cs
- HtmlInputButton.cs
- DataGridViewSelectedCellCollection.cs
- ObjectCloneHelper.cs
- ToolStripDesignerAvailabilityAttribute.cs
- Transform3D.cs
- WebServiceMethodData.cs
- WebPartActionVerb.cs
- XmlILOptimizerVisitor.cs
- ProtocolViolationException.cs
- HierarchicalDataBoundControl.cs
- ItemChangedEventArgs.cs
- Visual.cs
- SortDescription.cs
- XmlCharCheckingReader.cs