Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / PropertyEditing / categoryentry.cs / 1305376 / categoryentry.cs
namespace System.Activities.Presentation.PropertyEditing { using System.ComponentModel; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Activities.Presentation; using System; ////// The CategoryEntry class is a part of the property editing object model. It models a /// Category which has a localized name along with a collection of properties. /// public abstract class CategoryEntry : INotifyPropertyChanged, IPropertyFilterTarget { private string _name; private bool _matchesFilter; ////// Creates a new CategoryEntry. For host Infrastructure use /// /// The localized name of the corresponding Category as defined by the /// CategoryAttribute ///When name is either empty or null. protected CategoryEntry(string name) { if (string.IsNullOrEmpty(name)) throw FxTrace.Exception.ArgumentNull("name"); _name = name; } ////// Returns the localized Category name /// public string CategoryName { get { return _name; } } ////// Returns an IEnumerable collection of all of the properties in the category. /// public abstract IEnumerableProperties { get; } /// /// Indexer that returns a Property instance given the property name. /// /// The string property name to return a Property instance for. ///Property corresponding to the passed in propertyName if it exists, otherwise null public abstract PropertyEntry this[string propertyName] { get; } // INotifyPropertyChanged Members ////// INotifyPropertyChanged event /// public event PropertyChangedEventHandler PropertyChanged; ////// Raises the INotifyPropertyChanged.PropertyChanged event /// /// the name of the property that is changing ///When propertyName is null protected virtual void OnPropertyChanged(string propertyName) { if (propertyName == null) throw FxTrace.Exception.ArgumentNull("propertyName"); if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } // IPropertyFilterTarget Members ////// IPropertyFilterTarget event /// public event EventHandlerFilterApplied; /// /// Raises the IPropertyFilterTarget.FilterApplied event /// /// The PropertyFilter being applied protected virtual void OnFilterApplied(PropertyFilter filter) { if (FilterApplied != null) { FilterApplied(this, new PropertyFilterAppliedEventArgs(filter)); } } ////// IPropertyFilterTarget method /// /// public virtual void ApplyFilter(PropertyFilter filter) { this.MatchesFilter = filter == null ? true : filter.Match(this); OnFilterApplied(filter); } ////// IPropertyFilterTarget property /// public virtual bool MatchesFilter { get { return _matchesFilter; } protected set { if (_matchesFilter != value) { _matchesFilter = value; this.OnPropertyChanged("MatchesFilter"); } } } ////// IPropertyFilterTarget method /// /// The PropertyFilterPredicate to match against ///true if there is a match, otherwise false public abstract bool MatchesPredicate(PropertyFilterPredicate predicate); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Activities.Presentation.PropertyEditing { using System.ComponentModel; using System.Diagnostics; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Activities.Presentation; using System; ////// The CategoryEntry class is a part of the property editing object model. It models a /// Category which has a localized name along with a collection of properties. /// public abstract class CategoryEntry : INotifyPropertyChanged, IPropertyFilterTarget { private string _name; private bool _matchesFilter; ////// Creates a new CategoryEntry. For host Infrastructure use /// /// The localized name of the corresponding Category as defined by the /// CategoryAttribute ///When name is either empty or null. protected CategoryEntry(string name) { if (string.IsNullOrEmpty(name)) throw FxTrace.Exception.ArgumentNull("name"); _name = name; } ////// Returns the localized Category name /// public string CategoryName { get { return _name; } } ////// Returns an IEnumerable collection of all of the properties in the category. /// public abstract IEnumerableProperties { get; } /// /// Indexer that returns a Property instance given the property name. /// /// The string property name to return a Property instance for. ///Property corresponding to the passed in propertyName if it exists, otherwise null public abstract PropertyEntry this[string propertyName] { get; } // INotifyPropertyChanged Members ////// INotifyPropertyChanged event /// public event PropertyChangedEventHandler PropertyChanged; ////// Raises the INotifyPropertyChanged.PropertyChanged event /// /// the name of the property that is changing ///When propertyName is null protected virtual void OnPropertyChanged(string propertyName) { if (propertyName == null) throw FxTrace.Exception.ArgumentNull("propertyName"); if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } // IPropertyFilterTarget Members ////// IPropertyFilterTarget event /// public event EventHandlerFilterApplied; /// /// Raises the IPropertyFilterTarget.FilterApplied event /// /// The PropertyFilter being applied protected virtual void OnFilterApplied(PropertyFilter filter) { if (FilterApplied != null) { FilterApplied(this, new PropertyFilterAppliedEventArgs(filter)); } } ////// IPropertyFilterTarget method /// /// public virtual void ApplyFilter(PropertyFilter filter) { this.MatchesFilter = filter == null ? true : filter.Match(this); OnFilterApplied(filter); } ////// IPropertyFilterTarget property /// public virtual bool MatchesFilter { get { return _matchesFilter; } protected set { if (_matchesFilter != value) { _matchesFilter = value; this.OnPropertyChanged("MatchesFilter"); } } } ////// IPropertyFilterTarget method /// /// The PropertyFilterPredicate to match against ///true if there is a match, otherwise false public abstract bool MatchesPredicate(PropertyFilterPredicate predicate); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
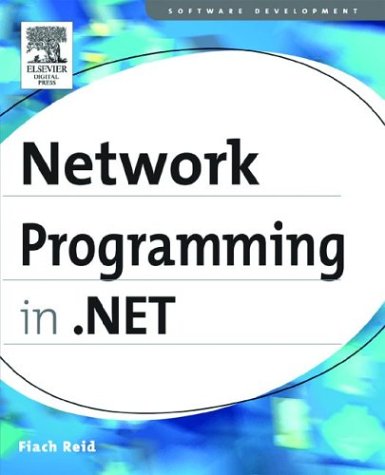
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewActionList.cs
- FieldNameLookup.cs
- FileDialog_Vista.cs
- PropertyExpression.cs
- Util.cs
- NetSectionGroup.cs
- PagedControl.cs
- TreeNodeCollection.cs
- BoolExpressionVisitors.cs
- GenerateScriptTypeAttribute.cs
- EntityDataSourceStatementEditor.cs
- RotateTransform3D.cs
- StackBuilderSink.cs
- DataRecordObjectView.cs
- FontUnitConverter.cs
- SystemWebSectionGroup.cs
- MemberInfoSerializationHolder.cs
- RegexReplacement.cs
- VisualTreeUtils.cs
- GlyphsSerializer.cs
- OdbcException.cs
- InvalidProgramException.cs
- WorkflowApplicationCompletedException.cs
- SimplePropertyEntry.cs
- ServiceDesigner.cs
- QfeChecker.cs
- ResourceExpression.cs
- Control.cs
- HwndProxyElementProvider.cs
- PropertyCollection.cs
- ActivityCompletionCallbackWrapper.cs
- DynamicQueryableWrapper.cs
- UserControlDocumentDesigner.cs
- FixedDSBuilder.cs
- TextBoxBase.cs
- AppSettingsExpressionBuilder.cs
- KeyToListMap.cs
- storagemappingitemcollection.viewdictionary.cs
- SizeAnimation.cs
- BitStack.cs
- ThreadInterruptedException.cs
- AddInControllerImpl.cs
- WeakReferenceEnumerator.cs
- ViewStateModeByIdAttribute.cs
- AttributeCollection.cs
- HitTestWithGeometryDrawingContextWalker.cs
- StrokeIntersection.cs
- RSAPKCS1KeyExchangeFormatter.cs
- TextEndOfSegment.cs
- ObjectQuery_EntitySqlExtensions.cs
- GridItem.cs
- DockAndAnchorLayout.cs
- CodeMemberField.cs
- DesignerVerb.cs
- TableRowGroup.cs
- FileDialogPermission.cs
- SpecularMaterial.cs
- AsyncStreamReader.cs
- EdmTypeAttribute.cs
- SelectionRangeConverter.cs
- InternalConfigSettingsFactory.cs
- WrapPanel.cs
- XmlValueConverter.cs
- DataServiceBehavior.cs
- ToolStripPanelRow.cs
- TextMetrics.cs
- Rfc2898DeriveBytes.cs
- SerializationInfoEnumerator.cs
- DetailsViewModeEventArgs.cs
- NamespaceEmitter.cs
- DesignerCategoryAttribute.cs
- SettingsAttributes.cs
- IChannel.cs
- NamespaceListProperty.cs
- XmlToDatasetMap.cs
- WinInet.cs
- DBConnection.cs
- _ConnectionGroup.cs
- ComMethodElement.cs
- OperatingSystem.cs
- DispatchChannelSink.cs
- CommandExpr.cs
- TemplateBaseAction.cs
- SqlFunctions.cs
- MaterialGroup.cs
- DataException.cs
- TemplateControlCodeDomTreeGenerator.cs
- TypefaceMetricsCache.cs
- FamilyMapCollection.cs
- ObjectParameterCollection.cs
- CollectionConverter.cs
- FolderBrowserDialog.cs
- XPathNode.cs
- TextEditorSpelling.cs
- Dictionary.cs
- ModelFunctionTypeElement.cs
- SapiGrammar.cs
- TimeSpanSecondsConverter.cs
- Screen.cs
- DocumentViewerAutomationPeer.cs